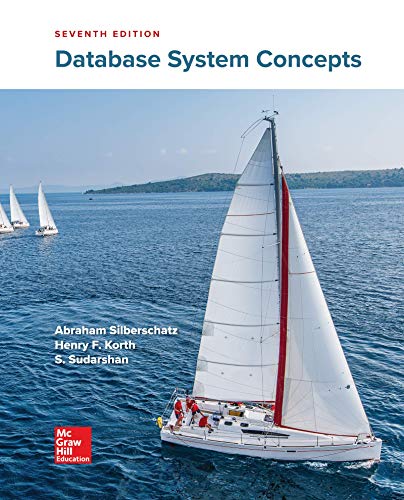
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
rite a complete program that will do the following in C++
- Declare an integer
vector without specifying any size. - Insert the values 5,10,15, 8, 4 into the vector
- Multiply the elements of vector using a for loop.
- Print the product of all the elements of the vector.
Expert Solution

arrow_forward
Step 1
c++ code is given below
arrow_forward
Step 2
#include <iostream>
#include <vector>
using namespace std;
int main()
{
vector<double> a = {5,10,15, 8, 4};
int multi = 1;
for(int i = 0; (i < a.size()); i++)
{
multi *= a[i];
}
cout << "\nProduct of vector elements : " <<multi;
return 0;
}
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Declare 2D array whose size and elements must be according to the desire of user then perform the following functions on it Display a lower triangular matrix. Find the sum of an upper triangular matrix. DO IT IN C++arrow_forwardIn C++, define an integer vector and ask the user to give you values for the vector. Because you used a vector, so you don't need to know the size. user should be able to enter a number until he wants(you can use while loop for termination. for example if the user enters any negative number, but until he enters a positive number, your program should read and push it inside of the vector). the calculate the average of all numbers he entered.arrow_forwardUsing C++ Using only these simpler vector methods Create a vector of at least 10 integers. Cout the vector with spaces in between the integers. Cout the vector backward. Using loops that traverse the elements, create a new vector with the elements arranged from high to low. Cout the new vector. Do not use iterators for this exercise, or any other advanced methods--only the ones listed below. Hint: Use multiple vectors, including the copying of vectors. vector<int> grades1 = {45, 67, 88, 45, 23}; vector<int> grades2 = grades1; May Only Use These Vector Methods: size() // Returns the number of elements empty() // Returns a boolean True if the vector is empty push_back(element) // Adds an element to the back of the vector pop_back() // Removes the element at the back of the vector front() // Returns the element at the front of the vector back()…arrow_forward
- USING C++ Program Specifications: Write a program to calculate the minimum, maximum, mean, median, mode, and whether a vector is a palindrome. Step 0. Review the starter code in main(). A vector is filled with integers from standard input. The first value indicates how many numbers are to follow and be placed in the vector. Step 1. Use a loop to process each vector element and output the minimum and maximum values. Submit for grading to confirm one test passes. Ex: If the input is: 6 4 1 5 4 99 17 the output is: Minimum: 1 Maximum: 99 Step 2. Use a loop to sum all vector elements and calculate the mean (or average). Output the mean with one decimal place using cout << fixed << setprecision(1); once before all other cout statements. Submit for grading to confirm two tests pass. Ex: If the input is: 6 4 1 5 4 99 17 the output is: Minimum: 1 Maximum: 99 Mean: 21.7 Step 3. Use a loop to determine if the vector is a palindrome, meaning values are the same from front to back and…arrow_forwardWrite a C++ Program that does the following: Implement a sort function using a vector.Ask the user to enter numbers to be sorted. Add them to the vector. The user will signal the end of input bygiving you a negative number.Then, sort the vector. Because this is a vector, you don’t need to pass the number of entriesin the vector.Then, print out the vector in order.Remember the following things about vectors.a) You declare a vector v of ints by saying vector⟨int⟩ v;b) You add elements to a vector by saying v.push back(thing to be added);c) You access elements of a vector with [ ] just like an array;d) One of the vector member functions is .length()arrow_forwardIs there any advantage to utilising vectors instead of arrays?arrow_forward
- Answer in C++ code please! (Duplicate Elimination with vector) Use a vector to solve the following problem. Read in 20 numbers, each of which is between 10 and 100, inclusive. As each number is read, validate it and store it in the vector only if it isn't a duplicate of a number already read. After reading all the values, display only the unique values that the user entered. Begin with an empty vector and use its push_back function to add each unique value to the vector.arrow_forwardAs part of the bring your own device (BYOD) program, the company CIO is encouraging employees to use their personal devices for business purposes. However, an attacker with the right kind of antenna can access the wireless network from a great distance, putting internal assets at risk. Of the following, what is the best solution? options: Turn off all wireless access Physically isolate wireless access from the wired network. Use a firewall on each device Use virtual private networkingarrow_forwardComplete the C++ code below#include "std_lib_facilities.h"/*1. Define a function which calculates the outer product of two vectors. The function return is a matrix. */vector<vector<int>> outerProduct(vector<int>& A, vector<int>& B){//implement here}/*2. Define a function which transposes a matrix. */vector<vector<int>> transpose(vector<vector<int>>& A){//implementation}/*3. Define a function which calculates the multiplication of a matrix and it's transpose. */vector<vector<int>> product(vector<vector<int>>& A){// implementation}/*4. Define a print out function that will print out a matrix in the following format:Example: a 3 by 4 matrix should have the print out:1 2 3 42 3 4 53 4 5 6*/void printMatrix(vector<vector<int>>& A){// implementation}int main(){// Define both vector A and vector Bvector<int> A = {1 ,2, 3, 4, 5};vector<int> B = {1, 2, 3};/*Test outerProduct…arrow_forward
- Complete this code using Python m1 = [] #Matrix 1m2 = [] #Matrix 2#Write a function that will return the addition of Matrix A and B.#Create a new matrix C that will hold the addtion result of Matrix A and B (A+B).#Return the resultant matrix Cdef addMatrix(A,B):#Write your code here#Write a function that will return the subtraction of Matrix B from A.#Create a new matrix C that will hold the substraction result of Matrix B from A (A-B).#Return the resultant matrix Cdef subsMatrix(A,B):#Write your code here#Write a function that will return the multiplication of Matrix A and B.#Create a new matrix C that will hold the multiplication result of Matrix A and B (A*B).#Keep in mind,in order to perform matrix multiplication, the number of columns in Matrix A must be equal to the number of columns in Matrix B. #Return the resultant matrix Cdef multipyMatrix(A,B):#Write your code here#Write a function that will transform matrix A to the transpose of matrix A.#The transpose of a matrix means…arrow_forwardWrite the following function #input: a numerical vector x #output:the normalized vector, i.e. the vector rescaled into [0,1] # the formula to normalize is (x-min(x))/ (max(x)-min(x)) # you can NOT use the functions min and max # you can use %%, nrow, ncol, and length # you can write multiple functionsarrow_forwardC++ Create a function append(v1, v2) that adds a copy of all the elements of vector v1 to the end of vector v2. The arguments are vectors of integers. Write a test driver.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
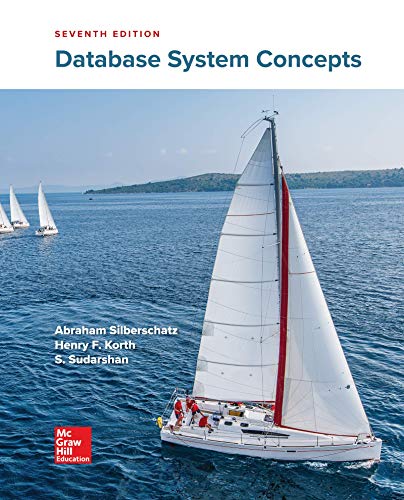
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
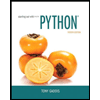
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
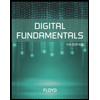
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
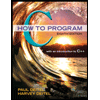
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
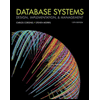
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
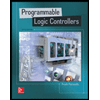
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education