Read the paint program below carefully and complete the source code paint.cpp by: 1. write function “myInit” of your own where you must a) setup viewport, b)set clear color to black and clear window, and c) setup matrix mode and viewing 2. Create your right menu containing the following submenus and main entries: a) color menu with eight entries “Red”, “Green”, “Blue”, “Cyan”, “Magenta”, “Yellow”, “White”, and “Black” b) pixel menu with two entries “Increase pixel size” and “Decrease pixel size” c) fill menu with two entries “Fill on” and “Fill off” d) two main entries “clear” and “quit” 3. Compile and run the program. Your complete program must be able to draw line segments, rectangles, triangles, pixels with specified color. It must also be able to accept keyboard input and display the text on the screen when work in text mode. /* paint.cpp */ #define LINE 1 #define RECTANGLE 2 #define TRIANGLE 3 #define POINTS 4 #define TEXT 5 #include #include // global variables GLsizei wh = 500, ww = 500; // initial window size GLfloat size = 3.0; // half side length of square int draw_mode = 0; // drawing mode int rx, ry; // raster position GLfloat r = 1.0, g = 1.0, b = 1.0; // drawing color int fill = 0; // fill flag // pick function int pick(int x, int y) { y = wh - y; if(y < wh-ww/10) return 0; else if(x < ww/10) return LINE; else if(x < ww/5) return RECTANGLE; else if(x < 3*ww/10) return TRIANGLE; else if(x < 2*ww/5) return POINTS; else if(x < ww/2) return TEXT; else return 0; } // draw square function void drawSquare(int x, int y) { y=wh-y; glColor3ub( (char) rand()%256, (char) rand()%256, (char) rand()%256); glBegin(GL_POLYGON); glVertex2f(x+size, y+size); glVertex2f(x-size, y+size); glVertex2f(x-size, y-size); glVertex2f(x+size, y-size); glEnd(); } // mouse event handlers void mouse(int btn, int state, int x, int y) { static int count; int where; static int xp[2],yp[2]; if(btn==GLUT_LEFT_BUTTON && state==GLUT_DOWN) { glPushAttrib(GL_ALL_ATTRIB_BITS); where = pick(x,y); glColor3f(r, g, b); if(where != 0) { count = 0; draw_mode = where; } else switch(draw_mode) { case(LINE): if(count==0) { count++; xp[0] = x; yp[0] = y; } else { glBegin(GL_LINES); glVertex2i(x,wh-y); glVertex2i(xp[0],wh-yp[0]); glEnd(); count=0; } break; case(RECTANGLE): if(count == 0) { count++; xp[0] = x; yp[0] = y; } else { if(fill) glBegin(GL_POLYGON); else glBegin(GL_LINE_LOOP); glVertex2i(x,wh-y); glVertex2i(x,wh-yp[0]); glVertex2i(xp[0],wh-yp[0]); glVertex2i(xp[0],wh-y); glEnd(); count=0; } break; case (TRIANGLE): switch(count) { case(0): count++; xp[0] = x; yp[0] = y; break; case(1): count++; xp[1] = x; yp[1] = y; break; case(2): if(fill) glBegin(GL_POLYGON); else glBegin(GL_LINE_LOOP); glVertex2i(xp[0],wh-yp[0]); glVertex2i(xp[1],wh-yp[1]); glVertex2i(x,wh-y); glEnd(); count=0; } break; case(POINTS): { drawSquare(x,y); count++; } break; case(TEXT): { rx=x; ry=wh-y; glRasterPos2i(rx,ry); count=0; } } glPopAttrib(); glFlush(); } } // keyboard input function void key(unsigned char k, int xx, int yy) { if(draw_mode!=TEXT) return; glColor3f(0.0,0.0,0.0); glRasterPos2i(rx,ry); glutBitmapCharacter(GLUT_BITMAP_9_BY_15, k); rx+=glutBitmapWidth(GLUT_BITMAP_9_BY_15,k); } // draw screen box void screen_box(int x, int y, int s ) { glBegin(GL_QUADS); glVertex2i(x, y); glVertex2i(x+s, y); glVertex2i(x+s, y+s); glVertex2i(x, y+s); glEnd(); }
The other half is in the photos
Read the paint program below carefully and complete the source code paint.cpp by:
/* paint.cpp */
#define LINE 1
#define RECTANGLE 2
#define TRIANGLE 3
#define POINTS 4
#define TEXT 5
#include <stdlib.h>
#include <GL/glut.h>
// global variables
GLsizei wh = 500, ww = 500; // initial window size
GLfloat size = 3.0; // half side length of square
int draw_mode = 0; // drawing mode
int rx, ry; // raster position
GLfloat r = 1.0, g = 1.0, b = 1.0; // drawing color
int fill = 0; // fill flag
// pick function
int pick(int x, int y)
{
y = wh - y;
if(y < wh-ww/10) return 0;
else if(x < ww/10) return LINE;
else if(x < ww/5) return RECTANGLE;
else if(x < 3*ww/10) return TRIANGLE;
else if(x < 2*ww/5) return POINTS;
else if(x < ww/2) return TEXT;
else return 0;
}
// draw square function
void drawSquare(int x, int y)
{
y=wh-y;
glColor3ub( (char) rand()%256, (char) rand()%256, (char) rand()%256);
glBegin(GL_POLYGON);
glVertex2f(x+size, y+size);
glVertex2f(x-size, y+size);
glVertex2f(x-size, y-size);
glVertex2f(x+size, y-size);
glEnd();
}
// mouse event handlers
void mouse(int btn, int state, int x, int y)
{
static int count;
int where;
static int xp[2],yp[2];
if(btn==GLUT_LEFT_BUTTON && state==GLUT_DOWN)
{
glPushAttrib(GL_ALL_ATTRIB_BITS);
where = pick(x,y);
glColor3f(r, g, b);
if(where != 0)
{
count = 0;
draw_mode = where;
}
else
switch(draw_mode)
{
case(LINE):
if(count==0)
{
count++;
xp[0] = x;
yp[0] = y;
}
else
{
glBegin(GL_LINES);
glVertex2i(x,wh-y);
glVertex2i(xp[0],wh-yp[0]);
glEnd();
count=0;
}
break;
case(RECTANGLE):
if(count == 0)
{
count++;
xp[0] = x;
yp[0] = y;
}
else
{
if(fill) glBegin(GL_POLYGON);
else glBegin(GL_LINE_LOOP);
glVertex2i(x,wh-y);
glVertex2i(x,wh-yp[0]);
glVertex2i(xp[0],wh-yp[0]);
glVertex2i(xp[0],wh-y);
glEnd();
count=0;
}
break;
case (TRIANGLE):
switch(count)
{
case(0):
count++;
xp[0] = x;
yp[0] = y;
break;
case(1):
count++;
xp[1] = x;
yp[1] = y;
break;
case(2):
if(fill) glBegin(GL_POLYGON);
else glBegin(GL_LINE_LOOP);
glVertex2i(xp[0],wh-yp[0]);
glVertex2i(xp[1],wh-yp[1]);
glVertex2i(x,wh-y);
glEnd();
count=0;
}
break;
case(POINTS):
{
drawSquare(x,y);
count++;
}
break;
case(TEXT):
{
rx=x;
ry=wh-y;
glRasterPos2i(rx,ry);
count=0;
}
}
glPopAttrib();
glFlush();
}
}
// keyboard input function
void key(unsigned char k, int xx, int yy)
{
if(draw_mode!=TEXT) return;
glColor3f(0.0,0.0,0.0);
glRasterPos2i(rx,ry);
glutBitmapCharacter(GLUT_BITMAP_9_BY_15, k);
rx+=glutBitmapWidth(GLUT_BITMAP_9_BY_15,k);
}
// draw screen box
void screen_box(int x, int y, int s )
{
glBegin(GL_QUADS);
glVertex2i(x, y);
glVertex2i(x+s, y);
glVertex2i(x+s, y+s);
glVertex2i(x, y+s);
glEnd();
}



Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

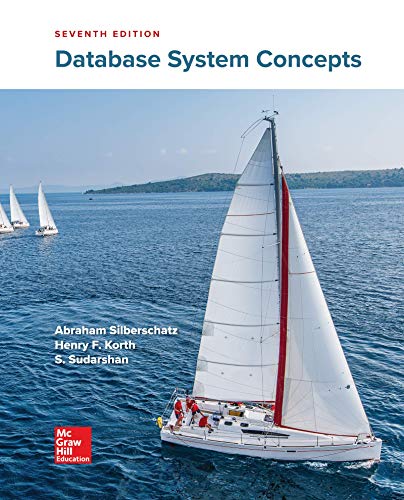
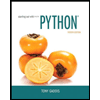
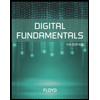
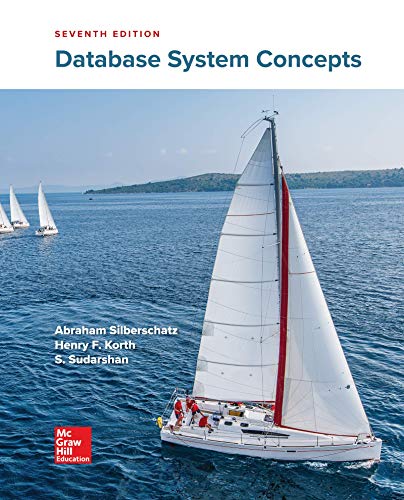
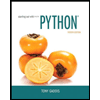
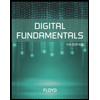
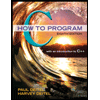
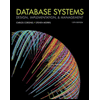
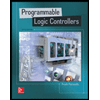