Race object 1. The Race object utilises Horse objects to simulate races, displaying them within the Command Prompt. 2. Races are configurable with varying lengths, measured in meters (or yards). 3. Each race comprises a specified number of lanes, where horses are allocated before the race begins. Some lanes may remain vacant. 4. Throughout the race, an animated display of the race progress is presented within the command-line terminal, providing a visual representation of the race in real-time. For an example, refer to simulation.mov 5. Once the race concludes, the results are promptly displayed on the terminal screen. Begin by thoroughly examining the Race class provided by McFarewell, which encompasses numerous features covered in the lectures. a) The startRace () method serves as the main method. Enhance this method to display the name of the winning horse in the terminal upon race completion. b) Conduct comprehensive testing of the Race class to identify potential areas for improvement. Document any encountered issues within the code and attempt to resolve as many of them as possible. Credit will be given for recognising problems, even if you're uncertain about the solutions. Additionally, propose alternative solutions for identified issues, regardless of whether your code implementation is successful. Below is a sample snapshot for a three-horse race': 乞 x² ==== | PIPPI LONGSTOCKING (Current confidence 0.6) KOKOMO (Current confidence 0.6) EL JEFE (Current confidence 0.4) And the winner is KOKOMO
in the image below the Red "X" denotes the horse has fallen
While the Race class is almost complete, it still contains some errors that need attention:
import java.util.concurrent.TimeUnit;
import java.lang.Math;
/**
* A three-horse race, each horse running in its own lane
* for a given distance
*
* @author McFarewell
* @version 1.0
*/
public class Race {
private int raceLength;
private Horse lane1Horse;
private Horse lane2Horse;
private Horse lane3Horse;
public Race(int distance) {
raceLength = distance;
lane1Horse = null;
lane2Horse = null;
lane3Horse = null;
}
public void addHorse(Horse theHorse, int laneNumber) {
if (laneNumber == 1) {
lane1Horse = theHorse;
} else if (laneNumber == 2) {
lane2Horse = theHorse;
} else if (laneNumber == 3) {
lane3Horse = theHorse;
} else {
System.out.println("Cannot add horse to lane " + laneNumber + " because there is no such lane");
}
}
public void startRace() {
boolean finished = false;
lane1Horse.goBackToStart();
lane2Horse.goBackToStart();
lane3Horse.goBackToStart();
while (!finished) {
moveHorse(lane1Horse);
moveHorse(lane2Horse);
moveHorse(lane3Horse);
printRace();
if (raceWonBy(lane1Horse) || raceWonBy(lane2Horse) || raceWonBy(lane3Horse)) {
finished = true;
}
try {
TimeUnit.MILLISECONDS.sleep(100);
} catch (Exception e) {}
}
}
private void moveHorse(Horse theHorse) {
if (!theHorse.hasFallen()) {
if (Math.random() < theHorse.getConfidence()) {
theHorse.moveForward();
}
if (Math.random() < (0.1 * theHorse.getConfidence() * theHorse.getConfidence())) {
theHorse.fall();
}
}
}
private boolean raceWonBy(Horse theHorse) {
return theHorse.getDistanceTravelled() == raceLength;
}
private void printRace() {
System.out.print('\u000C');
multiplePrint('=', raceLength + 3);
System.out.println();
printLane(lane1Horse);
System.out.println();
printLane(lane2Horse);
System.out.println();
printLane(lane3Horse);
System.out.println();
multiplePrint('=', raceLength + 3);
System.out.println();
}
private void printLane(Horse theHorse) {
int spacesBefore = theHorse.getDistanceTravelled();
int spacesAfter = raceLength - spacesBefore;
System.out.print('|');
multiplePrint(' ', spacesBefore);
if (theHorse.hasFallen()) {
System.out.print('\u2322');
} else {
System.out.print(theHorse.getSymbol());
}
multiplePrint(' ', spacesAfter);
System.out.print('|');
}
private void multiplePrint(char aChar, int times) {
for (int i = 0; i < times; i++) {
System.out.print(aChar);
}
}
}



Step by step
Solved in 2 steps

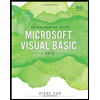
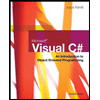
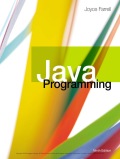
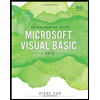
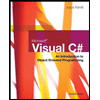
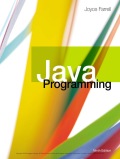
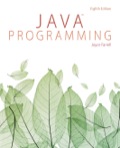