Python
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I'm not sure why my code keeps displaying: "* * * 1 REQUEST TIMED OUT" from a google.com host server.
Is there any issues on why it seems like it is stuck in a loop?
Here is my program in Python:
from socket import *
import socket
import os
import sys
import struct
import time
import select
import binascii
ICMP_ECHO_REQUEST = 8
MAX_HOPS = 30
TIMEOUT = 2.0
TRIES = 2
# The packet that we shall send to each router along the path is the ICMP echo
# request packet, which is exactly what we had used in the ICMP ping exercise.
# We shall use the same packet that we built in the Ping exercise
def checksum(str_):
# In this function we make the checksum of our packet
str_ = bytearray(str_)
csum = 0
countTo = (len(str_) // 2) * 2
for count in range(0, countTo, 2):
thisVal = str_[count + 1] * 256 + str_[count]
csum = csum + thisVal
csum = csum & 0xffffffff
csum = (csum >> 16) + (csum & 0xff00)
csum = csum + (csum >> 16)
answer = ~csum
answer = answer & 0xffff
answer = answer >> 8 | (answer << 8 & 0xff00)
return answer
def build_packet():
# In the sendOnePing() method of the ICMP Ping exercise, firstly the header of our
# packet to be sent was made, secondly the checksum was appended to the header and
# then finally the complete packet was sent to the destination.
# Make the header in a similar way to the ping exercise.
myChecksum = 0
myID = os.getpid() & 0xFFFF
# Make a dummy header with a 0 checksum.
# struct -- Interpret strings as packed binary data
header = struct.pack("bbHHh", ICMP_ECHO_REQUEST, 0, myChecksum, myID, 1)
data = struct.pack("d", time.time())
# Calculate the checksum on the data and the dummy header.
# Append checksum to the header.
myChecksum = checksum(header + data)
if sys.platform == 'darwin':
myChecksum = socket.htons(myChecksum) & 0xffff
# Convert 16-bit integers from host to network byte
else:
myChecksum = htons(myChecksum)
header = struct.pack("bbHHh", ICMP_ECHO_REQUEST, 0, myChecksum, myID, 1)
# So the function ending should look like this
packet = header + data
return packet
def ip_to_host(addr):
"""convert an IP address to a host name, returning shortname and fqdn to the caller"""
try:
fqdn = socket.gethostbyname(addr)[0]
shortname = fqdn.split('.')[0]
if fqdn == shortname:
fqdn = ""
except:
# can't resolve it, so default to the address given
shortname = addr
fqdn = "hostname not returnable"
return fqdn
def get_route(hostname):
timeLeft = TIMEOUT
tracelist2 = []
for ttl in range(1, MAX_HOPS):
for tries in range(TRIES):
destAddr = gethostbyname(hostname)
tracelist1 = []
tracelist2.append(tracelist1)
#Fill in start
# Make a raw socket named mySocket
icmp = socket.getprotobyname("ICMP")
mySocket = socket.socket(socket.AF_INET, socket.SOCK_RAW, icmp)
#Fill in end
mySocket.setsockopt(IPPROTO_IP, IP_TTL, struct.pack('I', ttl))
mySocket.settimeout(TIMEOUT)
try:
d = build_packet()
mySocket.sendto(d, (hostname,0))
t = time.time()
startedSelect = time.time()
whatReady = select.select([mySocket], [], [], timeLeft)
howLongInSelect = (time.time() - startedSelect)
if whatReady[0] == []: # Timeout
print(" * * * 1 Request timed out.")
tracelist1.append("* * * Request timed out.")
tracelist1.append(whatReady[0])
recvPakcet, addr = mySocket.recvfrom(1024)
timeRecieved = time.time()
timeLeft = timeLeft - howLongInSelect
if timeLeft <= 0:
print(" * * * 2 Request timed out.")
tracelist1.append("* * * Request timed out.")
except socket.timeout:
continue
else:
#Fill in start
#Fetch the icmp type from the IP packet
icmpHeader = recvPakcet[20:28]
request_type, code, checksum, packetID, sequence = struct.unpack("bbHHh", icmpHeader)
print(request_type)
#Fill in end
if request_type == 11:
bytes = struct.calcsize("d")
timeSent = struct.unpack("d", recvPakcet[28:28 + bytes])[0]
tracelist1.append((str(ttl),str(round((timeRecieved - t) * 1000)) + "ms", addr[0],ip_to_host(addr[0])))
elif request_type == 3:
bytes = struct.calcsize("d")
timeSent = struct.unpack("d", recvPakcet[28:28 + bytes])[0]
tracelist1.append((str(ttl),str(round((timeRecieved - t) * 1000)) + "ms", addr[0],ip_to_host(addr[0])))
elif request_type == 0:
bytes = struct.calcsize("d")
timeSent = struct.unpack("d", recvPakcet[28:28 + bytes])[0]
tracelist1.append((str(ttl),str(round((timeRecieved - t) * 1000)) + "ms", addr[0],ip_to_host(addr[0])))
else:
tracelist1.append("error")
break
finally:
mySocket.close()
get_route("google.com")
import socket
import os
import sys
import struct
import time
import select
import binascii
ICMP_ECHO_REQUEST = 8
MAX_HOPS = 30
TIMEOUT = 2.0
TRIES = 2
# The packet that we shall send to each router along the path is the ICMP echo
# request packet, which is exactly what we had used in the ICMP ping exercise.
# We shall use the same packet that we built in the Ping exercise
def checksum(str_):
# In this function we make the checksum of our packet
str_ = bytearray(str_)
csum = 0
countTo = (len(str_) // 2) * 2
for count in range(0, countTo, 2):
thisVal = str_[count + 1] * 256 + str_[count]
csum = csum + thisVal
csum = csum & 0xffffffff
csum = (csum >> 16) + (csum & 0xff00)
csum = csum + (csum >> 16)
answer = ~csum
answer = answer & 0xffff
answer = answer >> 8 | (answer << 8 & 0xff00)
return answer
def build_packet():
# In the sendOnePing() method of the ICMP Ping exercise, firstly the header of our
# packet to be sent was made, secondly the checksum was appended to the header and
# then finally the complete packet was sent to the destination.
# Make the header in a similar way to the ping exercise.
myChecksum = 0
myID = os.getpid() & 0xFFFF
# Make a dummy header with a 0 checksum.
# struct -- Interpret strings as packed binary data
header = struct.pack("bbHHh", ICMP_ECHO_REQUEST, 0, myChecksum, myID, 1)
data = struct.pack("d", time.time())
# Calculate the checksum on the data and the dummy header.
# Append checksum to the header.
myChecksum = checksum(header + data)
if sys.platform == 'darwin':
myChecksum = socket.htons(myChecksum) & 0xffff
# Convert 16-bit integers from host to network byte
else:
myChecksum = htons(myChecksum)
header = struct.pack("bbHHh", ICMP_ECHO_REQUEST, 0, myChecksum, myID, 1)
# So the function ending should look like this
packet = header + data
return packet
def ip_to_host(addr):
"""convert an IP address to a host name, returning shortname and fqdn to the caller"""
try:
fqdn = socket.gethostbyname(addr)[0]
shortname = fqdn.split('.')[0]
if fqdn == shortname:
fqdn = ""
except:
# can't resolve it, so default to the address given
shortname = addr
fqdn = "hostname not returnable"
return fqdn
def get_route(hostname):
timeLeft = TIMEOUT
tracelist2 = []
for ttl in range(1, MAX_HOPS):
for tries in range(TRIES):
destAddr = gethostbyname(hostname)
tracelist1 = []
tracelist2.append(tracelist1)
#Fill in start
# Make a raw socket named mySocket
icmp = socket.getprotobyname("ICMP")
mySocket = socket.socket(socket.AF_INET, socket.SOCK_RAW, icmp)
#Fill in end
mySocket.setsockopt(IPPROTO_IP, IP_TTL, struct.pack('I', ttl))
mySocket.settimeout(TIMEOUT)
try:
d = build_packet()
mySocket.sendto(d, (hostname,0))
t = time.time()
startedSelect = time.time()
whatReady = select.select([mySocket], [], [], timeLeft)
howLongInSelect = (time.time() - startedSelect)
if whatReady[0] == []: # Timeout
print(" * * * 1 Request timed out.")
tracelist1.append("* * * Request timed out.")
tracelist1.append(whatReady[0])
recvPakcet, addr = mySocket.recvfrom(1024)
timeRecieved = time.time()
timeLeft = timeLeft - howLongInSelect
if timeLeft <= 0:
print(" * * * 2 Request timed out.")
tracelist1.append("* * * Request timed out.")
except socket.timeout:
continue
else:
#Fill in start
#Fetch the icmp type from the IP packet
icmpHeader = recvPakcet[20:28]
request_type, code, checksum, packetID, sequence = struct.unpack("bbHHh", icmpHeader)
print(request_type)
#Fill in end
if request_type == 11:
bytes = struct.calcsize("d")
timeSent = struct.unpack("d", recvPakcet[28:28 + bytes])[0]
tracelist1.append((str(ttl),str(round((timeRecieved - t) * 1000)) + "ms", addr[0],ip_to_host(addr[0])))
elif request_type == 3:
bytes = struct.calcsize("d")
timeSent = struct.unpack("d", recvPakcet[28:28 + bytes])[0]
tracelist1.append((str(ttl),str(round((timeRecieved - t) * 1000)) + "ms", addr[0],ip_to_host(addr[0])))
elif request_type == 0:
bytes = struct.calcsize("d")
timeSent = struct.unpack("d", recvPakcet[28:28 + bytes])[0]
tracelist1.append((str(ttl),str(round((timeRecieved - t) * 1000)) + "ms", addr[0],ip_to_host(addr[0])))
else:
tracelist1.append("error")
break
finally:
mySocket.close()
get_route("google.com")
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
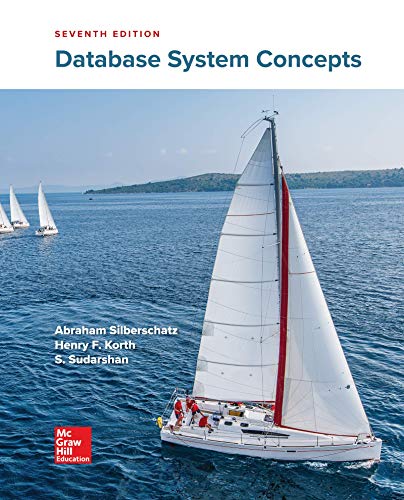
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
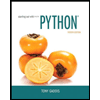
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
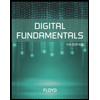
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
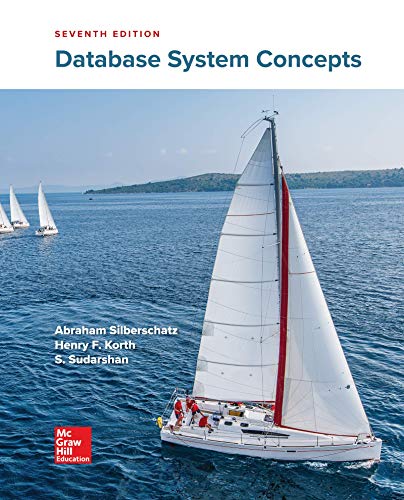
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
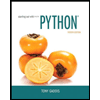
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
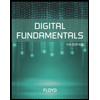
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
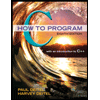
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
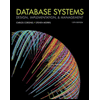
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
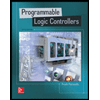
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education