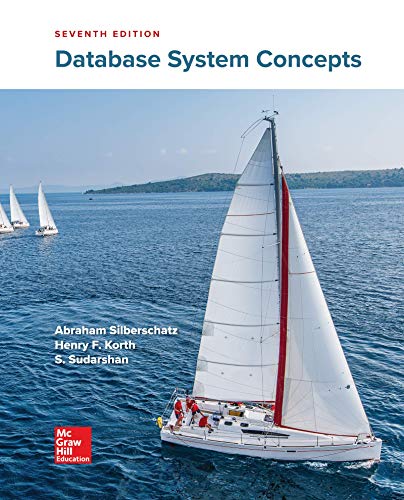
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
Returns True/False depending on the case (examples listed in the code). Write answers using one dimensional loops in Python.
![```python
def test1(arr):
"""
Return true if-and-only-if the sequence arr[0], * arr[arr[0]], arr[arr[arr[0]]], ... reaches 0 * after traversing all entries in arr.
Sample input/outputs:
* test1([3, 5, 7, 4, 1, 8, 0, 6, 2]) --> True
* test1([3, 5, 7, 4, 1, 8, 6, 0, 2]) --> False
* test1([3, 1, 0, 3, 0]) --> False
* test1([]) --> True
"""
pass
```
### Explanation
The function `test1` is designed to check if a sequence of indices in an array ultimately leads to a zero after traversing all entries. It evaluates the indices using the pattern `arr[0]`, `arr[arr[0]]`, `arr[arr[arr[0]]]`, and so on, returning `True` if this sequence results in reaching 0 and `False` otherwise.
#### Sample Inputs and Outputs
- `test1([3, 5, 7, 4, 1, 8, 0, 6, 2])` returns `True` because the sequence reaches 0 after the traversal.
- `test1([3, 5, 7, 4, 1, 8, 6, 0, 2])` returns `False` as the sequence does not end in a 0.
- `test1([3, 1, 0, 3, 0])` returns `False` indicating it doesn't resolve to 0.
- `test1([])` returns `True`, as an empty array trivially satisfies the condition without any elements.
This function could be used to solve problems related to array indexing and cyclical patterns in sequences.](https://content.bartleby.com/qna-images/question/cfa33f99-0a88-4c27-bdb9-7417e58c2439/579a2c34-394b-4c39-82a7-ce546e39b631/7qn7b5i_thumbnail.png)
Transcribed Image Text:```python
def test1(arr):
"""
Return true if-and-only-if the sequence arr[0], * arr[arr[0]], arr[arr[arr[0]]], ... reaches 0 * after traversing all entries in arr.
Sample input/outputs:
* test1([3, 5, 7, 4, 1, 8, 0, 6, 2]) --> True
* test1([3, 5, 7, 4, 1, 8, 6, 0, 2]) --> False
* test1([3, 1, 0, 3, 0]) --> False
* test1([]) --> True
"""
pass
```
### Explanation
The function `test1` is designed to check if a sequence of indices in an array ultimately leads to a zero after traversing all entries. It evaluates the indices using the pattern `arr[0]`, `arr[arr[0]]`, `arr[arr[arr[0]]]`, and so on, returning `True` if this sequence results in reaching 0 and `False` otherwise.
#### Sample Inputs and Outputs
- `test1([3, 5, 7, 4, 1, 8, 0, 6, 2])` returns `True` because the sequence reaches 0 after the traversal.
- `test1([3, 5, 7, 4, 1, 8, 6, 0, 2])` returns `False` as the sequence does not end in a 0.
- `test1([3, 1, 0, 3, 0])` returns `False` indicating it doesn't resolve to 0.
- `test1([])` returns `True`, as an empty array trivially satisfies the condition without any elements.
This function could be used to solve problems related to array indexing and cyclical patterns in sequences.
![```python
def test2(arr, k):
"""
Return True if-and-only-if the entries in arr are sorted sloppily
"up to k", that is, every entry precedes at most k smaller values
and follows at most k larger values.
Sample input/outputs:
* test2([3, 2, 1, 0, 4, 8, 7, 6, 5], 3) --> True
* test2([3, 2, 1, 0, 4, 8, 7, 6, 5], 2) --> False
* test2([0, 1, 2, 3, 4, 5, 6, 7, 8], 1) --> True
* test2([], 3) --> True
"""
pass
```
### Explanation
This Python function, `test2`, is designed to determine if the elements in an array `arr` are sorted in a relaxed manner with respect to a parameter `k`. This "sloppily sorted" condition means that each element can be preceded by at most `k` smaller elements and followed by at most `k` larger elements.
#### Sample Inputs and Outputs
1. **`test2([3, 2, 1, 0, 4, 8, 7, 6, 5], 3) --> True`**
The array allows each element to have at most 3 smaller and larger elements in its preceding or following positions.
2. **`test2([3, 2, 1, 0, 4, 8, 7, 6, 5], 2) --> False`**
Here, the condition fails because more than 2 smaller or larger elements can follow or precede some numbers.
3. **`test2([0, 1, 2, 3, 4, 5, 6, 7, 8], 1) --> True`**
This represents a normally sorted array that trivially satisfies the condition for `k=1`.
4. **`test2([], 3) --> True`**
An empty array is trivially considered sorted for any `k` value.
The function](https://content.bartleby.com/qna-images/question/cfa33f99-0a88-4c27-bdb9-7417e58c2439/579a2c34-394b-4c39-82a7-ce546e39b631/merf8y_thumbnail.png)
Transcribed Image Text:```python
def test2(arr, k):
"""
Return True if-and-only-if the entries in arr are sorted sloppily
"up to k", that is, every entry precedes at most k smaller values
and follows at most k larger values.
Sample input/outputs:
* test2([3, 2, 1, 0, 4, 8, 7, 6, 5], 3) --> True
* test2([3, 2, 1, 0, 4, 8, 7, 6, 5], 2) --> False
* test2([0, 1, 2, 3, 4, 5, 6, 7, 8], 1) --> True
* test2([], 3) --> True
"""
pass
```
### Explanation
This Python function, `test2`, is designed to determine if the elements in an array `arr` are sorted in a relaxed manner with respect to a parameter `k`. This "sloppily sorted" condition means that each element can be preceded by at most `k` smaller elements and followed by at most `k` larger elements.
#### Sample Inputs and Outputs
1. **`test2([3, 2, 1, 0, 4, 8, 7, 6, 5], 3) --> True`**
The array allows each element to have at most 3 smaller and larger elements in its preceding or following positions.
2. **`test2([3, 2, 1, 0, 4, 8, 7, 6, 5], 2) --> False`**
Here, the condition fails because more than 2 smaller or larger elements can follow or precede some numbers.
3. **`test2([0, 1, 2, 3, 4, 5, 6, 7, 8], 1) --> True`**
This represents a normally sorted array that trivially satisfies the condition for `k=1`.
4. **`test2([], 3) --> True`**
An empty array is trivially considered sorted for any `k` value.
The function
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- write program that uses recursion to calculate triangular numbers. Enter a value for the term number, n, and the program will display the value of the corresponding triangular number.shows the triangle.cpp program.arrow_forwardRecursion is similar to which of the following? a. Switch Case b. Loop c. If-else d. None of the mentionedarrow_forwardWrite a recursive program that takes a positive integer as an input and returns the sum of the digits of the integer. (Example: If the input is 45678, then output will be 30.)arrow_forward
- exponent = math.exp(-(math.pow(x-mean,2)/(2*math.pow(stdev,2)))) return (1 / (math.sqrt(2*math.pi) * stdev)) * exponent use laplace smoothing to remove the zero division error. Want a code in python The below code won't work as later when I use it create zero division error So laplace smoothing has to be added to the formula def calculateProbability(x, mean, stdev): try: exponent = math.exp(-(math.pow(x - mean, 2) / (2 * math.pow(stdev, 2)))) except ZeroDivisionError: exponent = 0 #or whatever print (stdev,"||",exponent) print (2 * math.pow(stdev, 2)) return (1 / (math.sqrt(2 * math.pi) * stdev)) * exponentarrow_forwardUse C++ Use nested for for loops.arrow_forwardWrite a recursive function called Rev takes a string argument (str) and and integerargument(i – the initial string position). Rev prints the str in reverse. You may not useany built-in reverse function or method. You may use string’s length method.arrow_forward
- Python Using recursion only No loops Note that in a correct solution the isdigit method or in operator will never be applied to the entire string s. The function must return and not print the resulting string. def getDigits(s):arrow_forwardExample in Java Write and trace sentinel-controlled while loop.arrow_forwardMake a code using Recursion The countSubstring function will take two strings as parameters and will return an integer that is the count of how many times the substring (the second parameter) appears in the first string without overlapping with itself. This method will be case insensitive. For example: countSubstring(“catwoman loves cats”, “cat”) would return 2 countSubstring(“aaa nice”, “aa”) would return 1 because “aa” only appears once without overlapping itself. public static int countSubstring(String s, String x) { if (s.length() == 0 || x.length() == 0) return 1; if (s.length() == 1 || x.length() == 1){ if (s.substring(0,1).equals(x.substring(0,1))){ s.replaceFirst((x), " "); return 1 + countSubstring(s.substring(1), x); } else { return 0 + countSubstring(s.substring(1), x); } } return countSubstring(s.substring(0,1), x) + countSubstring(s.substring(1), x); } public class Main { public static void main(String[] args) {…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
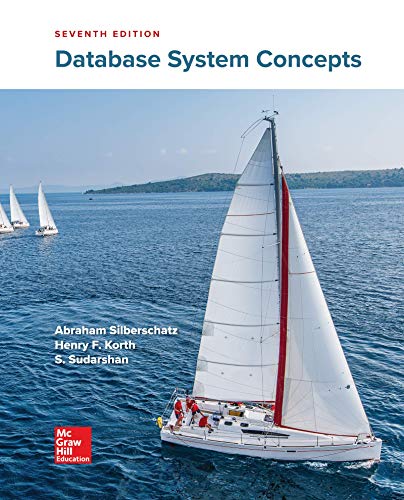
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
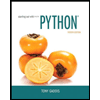
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
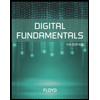
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
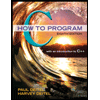
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
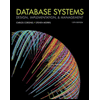
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
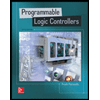
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education