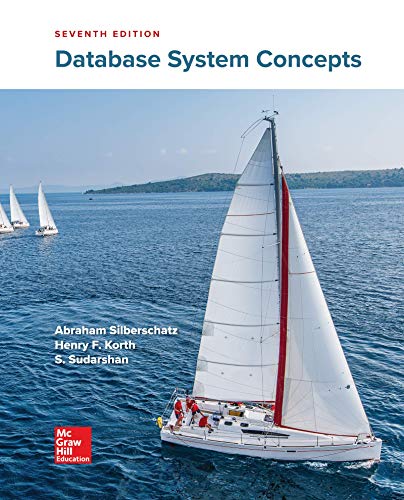
Python Turtle: How do I increase my circle size by 2x its current diamater, add/fix random mountain generation, keep lakes and mountains generating on the island (not off in the ocean), and have 'river' cuts going through the WHOLE circle instead of just stopping at the middle?
My code so far:
import turtle
import random
# Define colors
ocean = "#000066"
sand = "#ffff66"
grass = "#00cc00"
lake = "#0066ff"
mountain_color = "#808080" # Gray color for mountains
def draw_circle(radius, line_color, fill_color):
my_turtle.color(line_color)
my_turtle.fillcolor(fill_color)
my_turtle.begin_fill()
my_turtle.circle(radius)
my_turtle.end_fill()
def move_turtle(x, y):
my_turtle.penup()
my_turtle.goto(x, y)
my_turtle.pendown()
def draw_lake():
x = random.randint(-100, 100)
y = random.randint(-50, 100)
move_turtle(x, y)
draw_circle(30, lake, lake)
screen = turtle.Screen()
screen.bgcolor(ocean)
screen.title("Island Generator")
my_turtle = turtle.Turtle()
my_turtle.pensize(4)
my_turtle.shape("circle")
# Draw the sand area
draw_circle(150, sand, sand)
# Draw the grass area
move_turtle(0, 30)
draw_circle(120, grass, grass)
# Draw lakes as bigger random dots
num_lakes = 2 # Number of lakes to draw
for _ in range(num_lakes):
draw_lake()
#Draw mountains
num_mountains = 5 # Number of lakes to draw
for _ in range(num_mountains):
draw_mountains()
# Draw mountains as separate color
for pos in mountain_positions:
move_turtle(pos[0], pos[1])
draw_circle(15, mountain_color, mountain_color)
# Draw rivers
num_cuts = int(input("How many rivers do you want on your Island? "))
move_turtle(0, 150)
my_turtle.color(ocean)
for _ in range(num_cuts):
my_turtle.pendown()
my_turtle.left(360 / num_cuts)
my_turtle.forward(150)
my_turtle.penup()
my_turtle.backward(150)
turtle.done()

Step by stepSolved in 2 steps

- This is in Python. I'm using IDLE. Create a shape with turns of 45 which is 50 on a side. Make sure the sides come back together forming a octagon (stop sign) shape. Note: So far I had to make a square and a circle. This was the code. import turtle turtle.write("micpor9577")turtle.pencolor("purple")turtle.right(90)turtle.forward(100)turtle.right(90)turtle.forward(100)turtle.right(90)turtle.forward(100)turtle.right(90)turtle.forward(100) turtle.pencolor("blue")turtle.circle(50)turtle.donearrow_forwardYou are working as a software developer on NASA's Mars Explorer robotic rover project. You need to implement some code to compute the surface area of the 3D, tube-shaped part shown below: a cylinder of radius r and height h (in units centimeters) with a hollowed-out center also in the shape of a cylinder with radius n and height h (also in cm). Both the outside and the inside of the tube will need to be coated in a thin layer of gold, which is why we need to know its surface area. Write code that will compute and print the total surface area of the shape. n esc r Examples: Inputs r = 10, n = 8.5, h = 22.5 r = 9.25, n = 6.8, h r = 4.15, n = 3.9, h = 11.7 = 12.16 X h Output Value 2789.7342763877364 1473.347264273195 604.4267185874082 2 3 80 F3 000 DO F4 ✓0 F5 完成时间:18:42 PNEUE MacBook Air F6 F7 DII F8 tv 8 F9 F10 Farrow_forwardJAVA SWINGI have definied a straight TUBE as: int rectWidth = 25; // replace 50 with the desired width of the rectangleint rectHeight = 200; // replace 200 with the desired height of the rectangleint rectX = (this.getWidth() - rectWidth) / 2;int rectY = (this.getHeight() - rectHeight) / 2;game.fillRect(rectX, rectY, rectWidth, rectHeight);I need L tube, which connects the straight tubes. How to define L tubes?arrow_forward
- This question is in javaYou are walking along a hiking trail. On this hiking trail, there is elevation marker at every kilometer. The elevation information is represented in an array of integers. For example, if the elevation array is [100, 50, 20, 30, 50, 40], that means at kilometer 0, the elevation is 100 meters; at kilometer 1, the elevation is 50 meters; at kilometer 2, the elevation is 20 meters; at kilometer 3, the elevation is 30 meters; at kilometer 4, the elevation is 50 meters; at kilometer 5, the elevation is 40 meters. a) Write a method called dangerousDescent that determines whether there is a downhill section in the hiking trail with a slope of more than -0.05. The slope is calculated by the rise in elevation over the run in distance. For example, the last kilometer section has a gradient of -0.01 because the rise in elevation is 40 - 50 = -10 meters. The distance covered in 1000 meters. -10 / 1000 = 0.01. Your method should return true if there exists a dangerous…arrow_forwardPython Turtle: How do I fix the code to make it generate lakes as bigger random dots, and mountains as a separate color (gray) from the grass area? (Big error: lake generates as entire circle inside land, want it generating like mountains).AKA: How do I separate each of the values to generate on its own without conflicting eachother. import turtleocean = "#000066"sand = "#ffff66"grass = "#00cc00"lake = "#0066ff"mountain = [[-60, 115], [-65, 200], [-10, 145], [-30, 70], [50, 115], [100, 150], [70, 200], [30, 70], [10, 180], [-10, 220]]def draw_circle(radius, line_color, fill_color): my_turtle.color(line_color) my_turtle.fillcolor(fill_color) my_turtle.begin_fill() my_turtle.circle(radius) my_turtle.end_fill()def move_turtle(x, y): my_turtle.penup() my_turtle.goto(x, y) my_turtle.pendown()num_cuts = int(input("How many rivers do you want on your Island? ")) screen = turtle.Screen()screen.bgcolor(ocean)screen.title("Island Generator")my_turtle =…arrow_forwardThere are three main operations on rectangles: intersection, union, and difference. Among them, only intersection is guaranteed to return another rectangle. In general, the union of two rectangles is... two rectangles, and the difference between two rectangles is ... a whole lot of rectangles, as we will see. We let you implement rectangle intersection. Of course, the intersection is defined only if the rectangles have the same number of dimensions. The intersection is computed by taking the intersection of the intervals of the two rectangles for corresponding dimensions. If one of the intervals is empty, you should return None. [] # @ title Rectangle intersection def rectangle_and(self, other): if self.ndims != other.ndims: raise TypeError("The rectangles have different dimensions: {} and {}".format( )) self.ndims, other.ndims ### YOUR SOLUTION HERE Rectangle. _and_ = rectangle_and [ ] r1 = Rectangle((2, 3), (0, 4)) r2 = Rectangle((0, 4), (1, 3)) draw rectangles (r1, r2) draw…arrow_forward
- This is needed in Java Given an existing ArrayList named friendList, find the first index of a friend named Sasha and store it in a new variable named index.arrow_forwardJava programming help please. Main and other class of code is below. Thank you so so much. i have to Write a program that does the following: You have an array of integers with positive and negative values. using one ourArray object (will post code of "ourArrary" below) , and in two loops maximum, How can you reorganize the content of the array so that all negative numbers are on one side and the positive numbers are on the other side. [-3, 2, -4, 5, -6, -8,-9, 7, 13] The output should be [-3, -4, -6, -8, -9, 2, 5, 7, 13] Our array code: public class ourArray<T> implements iQueue{ private T[] Ar; private int size = 0; private int increment = 100; //default constructor @SuppressWarnings("unchecked") public ourArray () { Ar = (T[]) new Object[100]; size = 0; } //constructor that accepts the size @SuppressWarnings("unchecked") public ourArray (int n) { Ar = (T[]) new Object[n]; size = 0; } //constructor that specifies the initial capacity and the increment…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
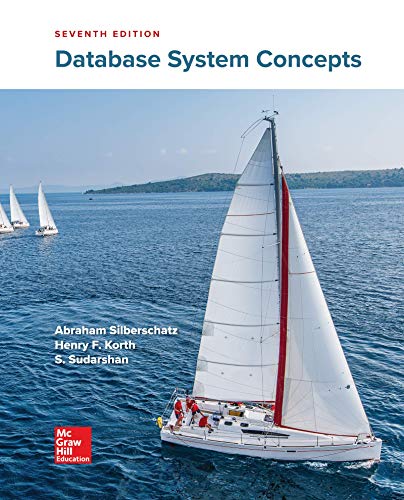
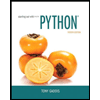
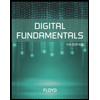
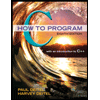
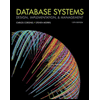
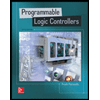