[Python (py3)] The error in the code below is if the number of rows of the matrix is not equal to its number of columns, matrix addition will not be performed. This should not be the case since the only requirement for matrices addition is that the dimension of Matrix A is equal to the dimension of Matrix B. Please resolve the error in the code below such that Matrix A and Matrix B can be added if the dimension of Matrix A is equal to the dimension of Matrix B. PLEASE do not just copy the code below and use it as the answer itself. I've encountered such case many times. Please modify the code. When the dimension of Matrix A is not equal to the dimension of Matrix B, print "Matrix addition cannot be performed; dimensions are unequal." The input will come from file1.txt, and the output should only be printed to output.txt Format of the input from file1.txt: First Line: type of operation (add) Second Line: matrix A dimension (example: if 3 rows and 2 columns, type 3 2) Third Line: matrix A elements Fourth Line: matrix B dimension Fifth Line: matrix B elements Fix the code such that it will work like this:
[Python (py3)]
The error in the code below is if the number of rows of the matrix is not equal to its number of columns, matrix addition will not be performed. This should not be the case since the only requirement for matrices addition is that the dimension of Matrix A is equal to the dimension of Matrix B. Please resolve the error in the code below such that Matrix A and Matrix B can be added if the dimension of Matrix A is equal to the dimension of Matrix B.
PLEASE do not just copy the code below and use it as the answer itself. I've encountered such case many times. Please modify the code.
When the dimension of Matrix A is not equal to the dimension of Matrix B, print "Matrix addition cannot be performed; dimensions are unequal."
The input will come from file1.txt, and the output should only be printed to output.txt
Format of the input from file1.txt:
First Line: type of operation (add)
Second Line: matrix A dimension (example: if 3 rows and 2 columns, type 3 2)
Third Line: matrix A elements
Fourth Line: matrix B dimension
Fifth Line: matrix B elements
Fix the code such that it will work like this:
Sample input 1:
add
2 3
53 -4 1
7 31 2
2 3
67 2 2
-34 6 3
Sample output 1:
120 -2 3
-27 37 5
Sample input 2:
add
2 2
53 -4
7 31
2 3
67 2 1
-34 6 2
Sample Output 2:
Matrix addition cannot be performed; dimensions are unequal.
----------------------------------------------------------------------
import numpy as np
import sys
f1 = open("file1.txt","r")
lines = f1.readlines()
dim = lines[1].split()
k=2
mat1 = np.empty((0,int(dim[0])), int)
for i in range(0,int(dim[0])):
l = lines[k].split()
l = list(map(int, l))
ls = np.array([l])
k += 1
mat1 = np.append(mat1, ls, axis=0)
dim2 = lines[k].split()
k += 1
if dim != dim2:
sys.exit("Matrix addition cannot be performed; dimensions are unequal.")
mat2 = np.empty((0,int(dim2[0])), int)
for i in range(0,int(dim2[0])):
l = lines[k].split()
l = list(map(int, l))
ls = np.array([l])
k += 1
mat2 = np.append(mat2, ls, axis=0)
print(np.add(mat1,mat2))
f2 = open("output.txt","w")
s = np.add(mat1,mat2)
# write output matrix in output.txt file
for i in range(0, len(s)):
for j in range(0, len(s[i])):
line = str(s[i][j])+" "
f2.write(line)
f2.write("\n")
# close the output file
f2.close()

Step by step
Solved in 4 steps with 3 images

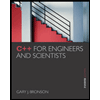
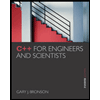