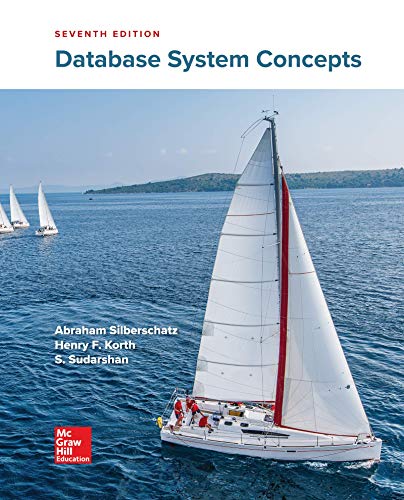
Python please
compare_sorts.py
import random #Import module for generating random numbers
import time #Import module for getting the current time
maxvalue = 1000
def merge(left, right):
result = []
left_idx, right_idx = 0, 0
while left_idx < len(left) and right_idx < len(right):
# to change direction of sort, change direction of comparison
if left[left_idx] <= right[right_idx]:
result.append(left[left_idx])
left_idx += 1
else:
result.append(right[right_idx])
right_idx += 1
if left:
result.extend(left[left_idx:])
if right:
result.extend(right[right_idx:])
return result
def merge_sort(m):
if len(m) <= 1:
return m
middle = len(m) // 2
left = m[:middle]
right = m[middle:]
left = merge_sort(left)
right = merge_sort(right)
return list(merge(left, right))
def insertion_sort(array):
for slot in range(1, len(array)):
value = array[slot]
test_slot = slot - 1
while test_slot > -1 and array[test_slot] > value:
array[test_slot + 1] = array[test_slot]
test_slot = test_slot - 1
array[test_slot + 1] = value
return array
def bubble_sort(array):
index = len(array) - 1
while index >= 0:
for j in range(index):
if array[j] > array[j + 1]:
array[j], array[j + 1] = array[j + 1], array[j]
index -= 1
return array
def main():
#ADD CODE to compare the three list-sorting functions
# See Canvas assignment for details.
main()
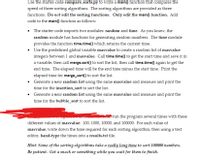

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 5 images

- show example on implementing Merge sort and Quick sortarrow_forwardDefine a sort function as below void sort(struct Employee * base], int n, int (*compareFunc)(struct Employee ** struct Employee **)) { qsort((void **)base,n,sizeof(void *),(int (*)(const void *,const void *))compareFunc); } Q1) What does the line in the provided sort function do? Describe each of the 4 arguments being passed to qsort. What do we need that big strange cast for the fourth argument?arrow_forwardFor the list shown below, demonstrate the following sort: 10, 1, 5, 2, 6, 8, 4, 10, 6, 6, 2, 4, 1, 8, 7, 3 External sort. Use a run size of 4. Note: continue until the final list is sorted!arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
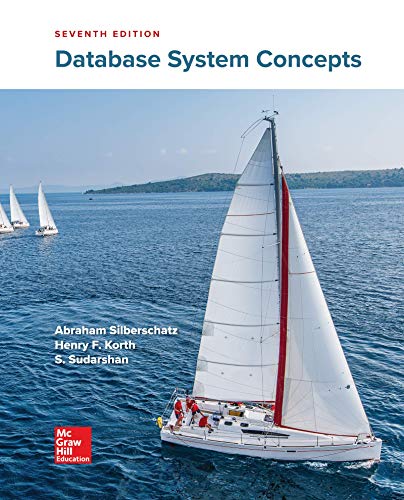
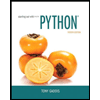
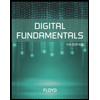
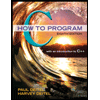
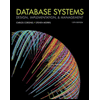
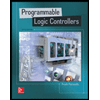