public static int addGrade(int[] allGrades, int grade, int listSize) { allGrades[listSize] = grade; ++listSize; return listSize; } public static void main(String[] args) { final int MAX_GRADES = 100; int[] courseGrades = new int[MAX_GRADES]; int numGrades = 0; } numGrades = addGrade(csGrades, 74, numGrades); numGrades = addGrade(csGrades, 74, numGrades); numGrades = addGrade (csGrades, 74, numGrades);
public static int addGrade(int[] allGrades, int grade, int listSize) { allGrades[listSize] = grade; ++listSize; return listSize; } public static void main(String[] args) { final int MAX_GRADES = 100; int[] courseGrades = new int[MAX_GRADES]; int numGrades = 0; } numGrades = addGrade(csGrades, 74, numGrades); numGrades = addGrade(csGrades, 74, numGrades); numGrades = addGrade (csGrades, 74, numGrades);
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
What is the length of array csGrades at the end of main()?
Image is shown below:
Group of answer choices
3
74
99
100
![```java
public static int addGrade(int[] allGrades, int grade, int listSize) {
allGrades[listSize] = grade;
++listSize;
return listSize;
}
public static void main(String[] args) {
final int MAX_GRADES = 100;
int[] courseGrades = new int[MAX_GRADES];
int numGrades = 0;
numGrades = addGrade(csGrades, 74, numGrades);
numGrades = addGrade(csGrades, 74, numGrades);
numGrades = addGrade(csGrades, 74, numGrades);
}
```
### Explanation
This Java program demonstrates a simple method for adding grades to an array:
- **addGrade Method:**
- It accepts an integer array `allGrades`, an integer `grade`, and an integer `listSize`.
- The method assigns the given `grade` to the `allGrades` array at the index specified by `listSize`.
- It then increments `listSize` to reflect the new number of grades.
- Finally, it returns the updated `listSize`.
- **main Method:**
- Defines a constant `MAX_GRADES` set to 100, indicating the maximum number of grades that can be stored.
- Creates an integer array `courseGrades` with a size of `MAX_GRADES`.
- Initializes `numGrades`, a counter for the number of grades, to 0.
- Calls `addGrade` three times, each time adding a grade of 74 to `courseGrades`.
- Updates `numGrades` with the new total number of grades after each addition.
### Usage
This program can be used as a foundation for managing student grades in a course, allowing you to add grades up to a predefined maximum limit.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4e135d5c-f867-4afd-9f0d-b505f4f19664%2F3e051943-e281-45f4-a06c-f4dbcfbcb68e%2Fkfdpctp_processed.png&w=3840&q=75)
Transcribed Image Text:```java
public static int addGrade(int[] allGrades, int grade, int listSize) {
allGrades[listSize] = grade;
++listSize;
return listSize;
}
public static void main(String[] args) {
final int MAX_GRADES = 100;
int[] courseGrades = new int[MAX_GRADES];
int numGrades = 0;
numGrades = addGrade(csGrades, 74, numGrades);
numGrades = addGrade(csGrades, 74, numGrades);
numGrades = addGrade(csGrades, 74, numGrades);
}
```
### Explanation
This Java program demonstrates a simple method for adding grades to an array:
- **addGrade Method:**
- It accepts an integer array `allGrades`, an integer `grade`, and an integer `listSize`.
- The method assigns the given `grade` to the `allGrades` array at the index specified by `listSize`.
- It then increments `listSize` to reflect the new number of grades.
- Finally, it returns the updated `listSize`.
- **main Method:**
- Defines a constant `MAX_GRADES` set to 100, indicating the maximum number of grades that can be stored.
- Creates an integer array `courseGrades` with a size of `MAX_GRADES`.
- Initializes `numGrades`, a counter for the number of grades, to 0.
- Calls `addGrade` three times, each time adding a grade of 74 to `courseGrades`.
- Updates `numGrades` with the new total number of grades after each addition.
### Usage
This program can be used as a foundation for managing student grades in a course, allowing you to add grades up to a predefined maximum limit.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
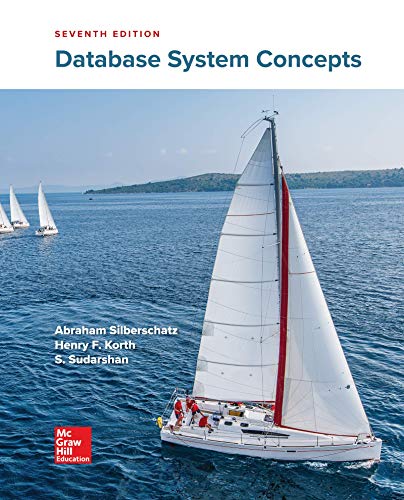
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
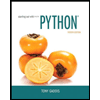
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
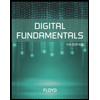
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
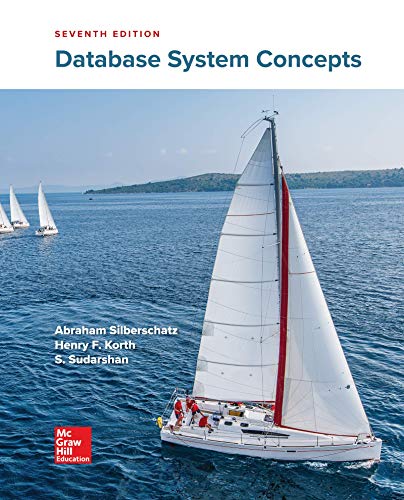
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
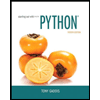
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
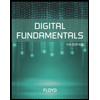
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
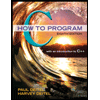
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
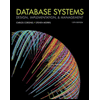
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
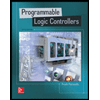
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education