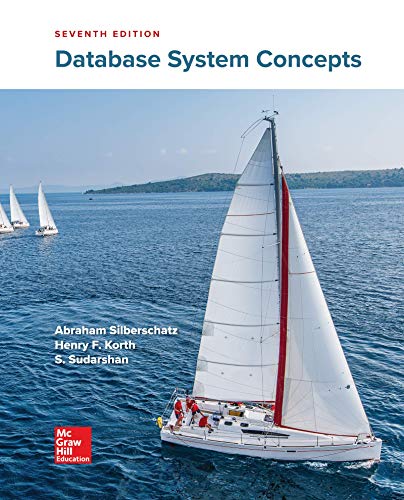
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
program7.py
This assignment requires the main function and a custom value-returning function. The value-returning function takes a list of random integers as its only argument and returns a smaller list of only the elements that end with 7. This value-returning function must use a list comprehension to create this smaller list.
In the main function, code these steps in this sequence:
- Set random seed to 42 import random
random.seed(42) - create an empty list that will the hold random integers.
- use a loop to add 50 random integers to the list. All integers should be between 200 and 250, inclusive. Duplicates are okay.
- sort the list in ascending order and then use another loop to display all 50 sorted integers on one line separated by spaces.
- print a slice showing list elements indexed 5 through 10, inclusive.
- print a second slice showing the final 5 elements in the sorted list.
- execute the custom function with the entire original list as its sole argument.
- report the number of elements in the new "sevens" list returned by the custom function.
- if 207 is not in the "sevens" list, add it to the start of "sevens" and report that this happened. Otherwise, report that nothing was added.
- if 247 is in the "sevens" list, report the index of its first occurrence. Otherwise, append 247 to the end of the list.
- use another loop to display all elements in "sevens" on one line separated by spaces.
- Finally, report the total of the "sevens" list.
See attatched image for what the output needs to be
I need pseudocode and an explanation of each step so I can learn
![Here is the complete list of 50 elements,sorted low to high...
200 200 201 203 203 203 203 204 205 207 209 210 210 211 212 213 213 213 216 217
218 218 219 220 221 221 221 222 224 224 224 225 225 226 228 229 231 234 234 237
241 244 245 246 246 247 247 248 248 250
Elements indexed 5 to 10 in the sorted list are [203, 203, 204, 205, 207, 209]
THe final 5 elements in the sorted list are [247, 247, 248, 248, 250]
Number of elements ending with 7: 5
207 was already in the "sevens" list, so nothing was added
Found 247 at index 3 in the "sevens" list, so nothing was added
Here are the elements in the "sevens" list...
207 217 237 247 247
The total of all elements in the "sevens" list is 1155](https://content.bartleby.com/qna-images/question/098cc80e-1667-4a39-bf33-ff7a640d05d4/be7a3d1c-34cc-4d61-84b7-c1ec20cfdd20/avmrwio_thumbnail.png)
Transcribed Image Text:Here is the complete list of 50 elements,sorted low to high...
200 200 201 203 203 203 203 204 205 207 209 210 210 211 212 213 213 213 216 217
218 218 219 220 221 221 221 222 224 224 224 225 225 226 228 229 231 234 234 237
241 244 245 246 246 247 247 248 248 250
Elements indexed 5 to 10 in the sorted list are [203, 203, 204, 205, 207, 209]
THe final 5 elements in the sorted list are [247, 247, 248, 248, 250]
Number of elements ending with 7: 5
207 was already in the "sevens" list, so nothing was added
Found 247 at index 3 in the "sevens" list, so nothing was added
Here are the elements in the "sevens" list...
207 217 237 247 247
The total of all elements in the "sevens" list is 1155
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In Python programming languagearrow_forwardin PYTHON Write a function that accepts a list as an argument and calculates the sum of each elementof the list.arrow_forward1) Write a function that takes one argument, a list named sample-list. 2 ) If the length of the sample-list is > 8, then use the last element of sample-list and return it in a list. 3) If the length of sample-list is <= 8 and > 4, then return the first elements of sample-list in a list. 4) Otherwise, return the string “This is the third option.”. Please make sure to test your function!!! and explain your code shortly by adding comments to each line.arrow_forward
- Im Python pleasearrow_forwardHow to write reporting function on this question This program implements the buying and selling of stocks. It starts by printing a welcome message Welcome to mystocks.com Then a main menu of choices for the user is output. Reporting, buying or selling? (0=quit, 1=report, 2=buy, 3=sell): The program ends with a goodbye message. Thank you for trading with mystocks.com Use doubly linked list of stock_t structures. stock_t structure looks like: #define MAX_TICKER_LENGTH 6 typedef struct stock_t { char ticker[MAX_TICKER_LENGTH]; date_t date; // date bought int numShares; double pricePerShare; } stock_t; date_t structure looks like: typedef struct date_t { int month, day, year; } date_t; Create the following files in a directory: date.h: contains the above date_t structure • stock.h and stock.c: contain the stock structure and any constants and stock function prototypes. Eg: a print stock function. • node.h, node.c: contain at least the node typdef and initNode function •…arrow_forwardpython LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forward
- QUESTION 4 in phython language Write a program that creates a list of N elements (Hint: use append() function), swaps the first and the last element and prints the list on the screen. N should be a dynamic number entered by the user. Sample output: How many numbers would you like to enter?: 5 Enter a number: 34 Enter a number: 67 Enter a number: 23 Enter a number: 90 Enter a number: 12 The list is: [12, 67,23, 90, 34]arrow_forwardWrite a function called find_duplicates which accepts one list as a parameter. This function needs to sort through the list passed to it and find values that are duplicated in the list. The duplicated values should be compiled into another list which the function will return. No matter how many times a word is duplicated, it should only be added once to the duplicates list. NB: Only write the function. Do not call it. For example: Test Result random_words = ("remember","snakes","nappy","rough","dusty","judicious","brainy","shop","light","straw","quickest", "adventurous","yielding","grandiose","replace","fat","wipe","happy","brainy","shop","light","straw", "quickest","adventurous","yielding","grandiose","motion","gaudy","precede","medical","park","flowers", "noiseless","blade","hanging","whistle","event","slip") print(find_duplicates(sorted(random_words))) ['adventurous', 'brainy', 'grandiose', 'light', 'quickest', 'shop', 'straw', 'yielding']…arrow_forwardQuestion 15 kk .Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this linearrow_forward
- Create a list L containing at least 10 values ranging from j to k. Using a for loop, code a function that will compute and display the following list: L_out[0] = L[0] L_out[i] = L[i] + L[i-1] if i is odd and i is not equal to 0 L_out[i] = L[i] * 3 if i is even and i is not equal to 0 where L[i] represents the value stored in L at the position i. Example: L ‘(2 3 4 5) L_out ‘(2 5 12 9)arrow_forwardb. Write a function cut12 that removes the last two items from a list given as its argument. The existing list should be changed in-place; the function should not return anything,arrow_forwardComplete the reverse_list() function that returns a new string list containing all contents in the parameter but in reverse order. Ex: If the input list is: ['a', 'b', 'c'] then the returned list will be: ['c', 'b', 'a'] Note: Use a for loop. DO NOT use reverse() or reversed(). This is my code so far: def reverse_list(letters): for x in (letters): letters.sort(reverse=True) return lettersif __name__ == '__main__': ch = ['a', 'b', 'c'] print(reverse_list(ch)) # Should print ['c', 'b', 'a'] When submitting I get 4/10 and it says: Test input list ['a', 'b', 'c'] returns ['c', 'b', 'a'] Your output reverse_list() function reversed array incorrectly: ['c', 'b', 'a'] reverse_list() function should have returned: ['a', 'b', 'c'] Test input list ['a', 'b', 'c', 'd'], first element of returned list is ['d'] Your output First character of returned array should be a instead of d Test empty input array TypeError: object of type 'NoneType' has no len()arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
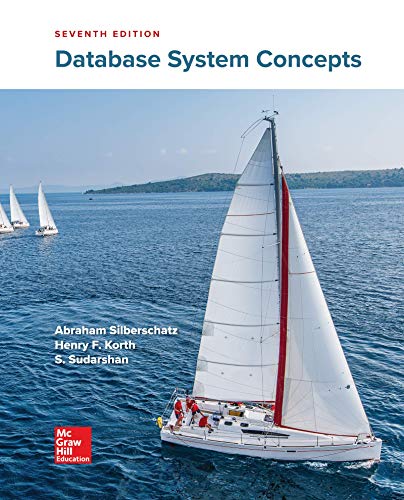
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
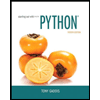
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
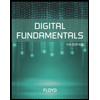
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
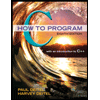
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
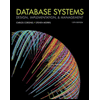
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
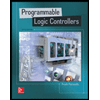
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education