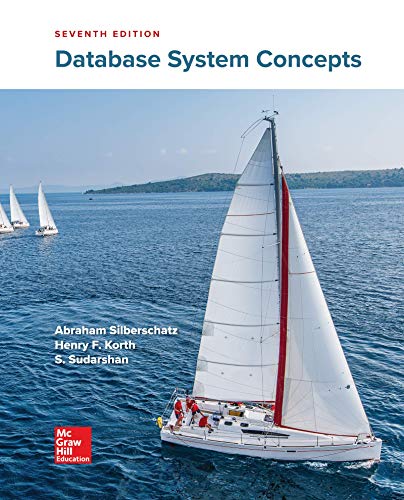
**
This lab is to to add another option for the user to print warnings of any inventory running low. More specifically:
- Add another option to the menu, called W for Warning
- Look at all items, in all locations. If an item has less than 10 left, print out on one line the item name,
location, and quantity.
A photo of the example run is attached
Contents of Inventory.txt
Red delicious apples
1.00 25 6 8 10
Assorted bouquets
4.00 50 10 10 0
Camembert cheese
2.00 25 10 12 4
END
Program that is being added to:
#include <iostream>
#include <fstream>
#include <
using namespace std;
struct Record {
string name;
double cost;
int markup;
int count[3];
};
const string places[3] = {"counter", "shelf", "warehouse"};
bool read_file(vector <Record> &v);
void placement (vector <Record> &v);
int main()
{
vector <Record> invent;
char choice;
if (read_file(invent) == false) {
return 1;
}
cout << "You have " << invent.size() << endl;
while (true)
{
cout << "(P)lacement e(X)it: ";
cin >> choice;
switch(choice) {
case 'P':
case 'p':
placement(invent);
break;
case 'X':
case 'x':
return(0);
break;
default:
cout << choice << " is not a chice\n";
}
}
return 0;
}
void placement (vector <Record> &v)
{
int which = -1;
string input;
cout << "Which? ";
cin >> input;
for (int i = 0; i < 3; i++) {
if (input.substr(0,3) == places[i].substr(0,3)) which = i;
}
if (which == -1) {
cout << "No match\n";
return;
}
cout.setf(ios::left);
cout.unsetf (ios::right);
cout.width(30);
cout << "Item";
cout.setf(ios::right);
cout.width(6);
cout << places[which] << "\n";
int j;
for (j = 0; j < v.size(); j++) {
cout.setf(ios::left);
cout.unsetf (ios::right);
cout.width(30);
cout << v[j].name;
cout.setf(ios::right);
cout.width(6);
cout << v[j].count[which] << endl;
}
}
bool read_file(vector <Record> &v)
{
Record r;
ifstream infile;
infile.open("inventory.txt");
if (infile.fail()) {
cout << "can't open file\n";
return (false);
}
while (true) {
getline (infile, r.name);
cout << "Read " << r.name << endl;
if (r.name == "END") {
infile.close();
return true;
}
else {
infile >> r.cost >> r.markup;
for (int i = 0; i < 3; i++) infile >> r.count[i];
infile.get();
v.push_back(r);
}
}
return true;
}
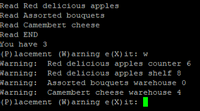

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Write the code to allow the user to enter a list of positive integers via input boxes and add each number entered to the lstNums list box. Use a Do-While or Do-Until Loop to let the user continue to enter numbers until -1 is entered. Do not add the -1 entry to the list box. In visual Basic please.arrow_forwardFill in the first blank to compute the total number of calories for all items on the menu and fill in the 2nd blank to compute the number of items on the menu:arrow_forwardAlert dont submit AI generated answer.arrow_forward
- Which is correct option for below question. Options are 0,7,none of these. With proper explanationarrow_forwardLAB ASSIGNMENTS IMPORTANT: you should complete the PDP thinking process for each program. Turn in items: 1) fullname_regex.py - Ask the user to enter the source text to search, such as the name_source variable here: name_source = input('Enter full name in this format - first middle last'). Then, you can adapt the first two code lines from lesson slide 13, to search this new source. Edit the code to match the new situation and change the regex pattern to identify text that could be a full name. Hints: Initially write your regex pattern to check if the user enters three words separated by a space. Then, strive to make the regex adaptable if the person's full name has more than or less than 3 words. Then, think about allowing (not requiring) common characters like a period, hyphen or '. Slides 11-13 should be helpful. 08 For printing, end with a conditional block that provides an appropriate message if there is a match or not. Match Enter your full name: first middle last Betty Lou Who…arrow_forwardPython question please include all steps and screenshot of code. Also please provide a docstring, and comments throughout the code, and test the given examples below. Thanks. A single playing card can be represented by a string where the first character is a digit2-9 or a letter ‘A’, ‘T’, ‘J’, ‘Q’, or ‘K’, for Ace, Ten, Jack, Queen, King respectively, andthe second character is one of ‘S’, ‘H’, ‘D’, or ‘C’ for Spades, Hearts, Diamonds, orClubs. A hand of cards can be represented by a list of strings. Write a function calledthreeOfAKind() that takes a list of strings representing a hand of cards, and returns Trueif there are exactly three cards with the same value (regardless of suit), False otherwise.>>> threeOfAKind(['3D', 'KD', 'JD', '3H', '3S'])True>>> threeOfAKind(['3D', 'KD', 'JD', '3H', '4S', '5S'])False>>> threeOfAKind(['3D', '3H', '3S', '3C', 'AC'])Falsearrow_forward
- Monthly Payment Program (in the attached photo), update it with the following in java code: If applicable, remove hard coding for the principal, annual interest rate and number of years. Replace these with Input Dialog Boxes requesting data be entered. The calculation should remain the same. Replace the output area with a showMessage box. Allow the user to request a detailed display of the payment schedule. This will include the ending balance and interest paid per month in a line-by-line display. Add a loop to the program allowing the user to continue entering new data as many times as they like (all input and output should be displayed in dialog boxes).arrow_forwardA program is needed to manage sales of Super Bowl souvenir t-shirts. There are three types of shirts that we are selling: Chiefs t-shirts Eagles t-shirts Super Bowl t-shirts The t-shirts are all priced at $40 each without any customization. Input: Your program is to display a menu listing each type of shirt. It should then prompt the user for the number of Chiefs t-shirts they would like to order. After the number of Chiefs t-shirts has been entered (which may be 0), if the number was greater than 0, we will ask if they need to add lettering to the shirt (costs 1 dollar per printed letter) the program should continue displaying a prompt and reading the number to order for the other two types of t-shirts in the same way. The program also needs to keep track of the customer name and mailing address. Prompt and read the customer’s name, street address, city, state, and zip code.arrow_forwardTaking a Restaurant Order: User Confirmation Style. Using the restaurant menu you selected below you will create a program that will take an order from a customer and calculate the total. Your program shall, Display the menu with the prices. Take an order using the User Confirmation approach The user will input numbers between 1 and 10, according to the menu you created in assigment below. Inform the user about invalid input values (greater than 10 or less than 1) Add the prices of the dishes ordered (you will need a variable to add the prices as in Listing 5.5, p. 141). After taking the order, the program displays the subtotal, the sales tax (8.75%), the grand total, and a suggested 15% tip. You can use the program you created below and modify to incorporate the new requirements. Notice that you will still need the multi-way conditional to add the right price value to the running total of the order. import sys #Enter number for each dish and price for users DishNumber=…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
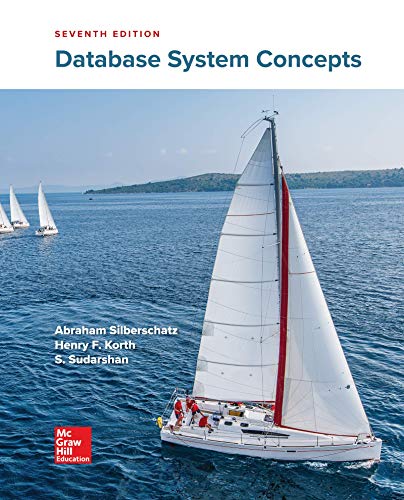
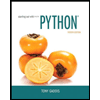
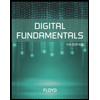
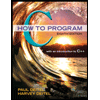
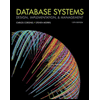
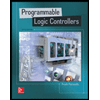