Program 2.3. MATLAB function for uniform quantization encoding. function [ I, pq]= biquant (NoBits, Xmin, Xmax,value) % function pq = biquant (NoBits, Xmin, Xmax, value) % This routine is created for simulation of uniform quantizer. % NOBits: number of bits used in quantization. % Xmax: overload value. % Xmin: minimum value % value: input to be quantized. % pq: output of quantized value % I: coded integer index L=2^NoBits; delta=(Xmax- Xmin)/L; I=round ((value-Xmin)/delta); if ( I==L) I=I-1; end if I<0 I=0; end pq=Xmin+I*delta; Program 2.4. MATLAB function for uniform quantization decoding. function pq =biqtdec(NoBits,Xmin,Xmax, I) % function pq = biqtdec(NoBits, Xmin, Xmax, I) % This routine recover the quantized value. % NOBits: number of bits used in quantization. % Xmax: overload value % Xmin: minimum value % pq: output of quantized value % I: coded integer index L=2°NoBits; delta=(Xmax- Xmin)/L; pq=Xmin+I*delta; Program 2.5. MATLAB function for calculation of signal-to-quantization noise ratio. function snr - calcsnr(speech, qspeech) % function snr = calcsnr(speech, qspeech) % this routine is created for calculation of SNR % speech: original speech waveform. % qspeech: quantized speech. % snr: output SNR in dB. qerr = speech-qspeech; snr = 10*1og10(s um(speech.*speech)/sum(qerr.*qerr))
Program 2.3. MATLAB function for uniform quantization encoding. function [ I, pq]= biquant (NoBits, Xmin, Xmax,value) % function pq = biquant (NoBits, Xmin, Xmax, value) % This routine is created for simulation of uniform quantizer. % NOBits: number of bits used in quantization. % Xmax: overload value. % Xmin: minimum value % value: input to be quantized. % pq: output of quantized value % I: coded integer index L=2^NoBits; delta=(Xmax- Xmin)/L; I=round ((value-Xmin)/delta); if ( I==L) I=I-1; end if I<0 I=0; end pq=Xmin+I*delta; Program 2.4. MATLAB function for uniform quantization decoding. function pq =biqtdec(NoBits,Xmin,Xmax, I) % function pq = biqtdec(NoBits, Xmin, Xmax, I) % This routine recover the quantized value. % NOBits: number of bits used in quantization. % Xmax: overload value % Xmin: minimum value % pq: output of quantized value % I: coded integer index L=2°NoBits; delta=(Xmax- Xmin)/L; pq=Xmin+I*delta; Program 2.5. MATLAB function for calculation of signal-to-quantization noise ratio. function snr - calcsnr(speech, qspeech) % function snr = calcsnr(speech, qspeech) % this routine is created for calculation of SNR % speech: original speech waveform. % qspeech: quantized speech. % snr: output SNR in dB. qerr = speech-qspeech; snr = 10*1og10(s um(speech.*speech)/sum(qerr.*qerr))
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Given an original speech segment “speech.dat” sampled at 8000 Hz with each sample encoded in 16 bits, use Programs 2.3–2.5 and modify Program 2.2 to quantize the speech segment using 3–15 bits, respectively. The SNR in dB must be measured for each quantization. MATLAB function: “sound(xmax(abs(x)),fs)” can be used to evaluate sound quality, where “x” is the speech segment while “fs” is the sampling rate of 8000 Hz. In this project, create a plot of the measured SNR (dB) versus the number of bits and discuss the effect of the sound quality. For comparisons, plot the original speech and the quantized one using 3, 8, and 15 bits.
![(a) Program 2.2 MATLAB program for Example 2.14.
%Example 2.14
clear all; close all
disp('load speech: We');
load we.dat
sig = we;
fs=8000;
% Load speech data at the current folder
% Provided by the instructor
% Sampling rate
% Length of signal vector
% Sampling period
lg=length(sig);
T=1/fs;
Co
CHAPTER 2 SIGNAL SAMPLING AND QUANTIZATION
EXAMPLE 2.14–CONT'D
t=[0:1:lg-1]*T;
sig=4.5*sig/max(abs(sig));
Xmax = max(abs(sig));
Xrms = sqrt( sum(sig .* sig) / length(sig))
disp('Xrms/Xmax’)
k=Xrms/Xmax
% Time instants in second
% Normalizes speech in the range from -4.5 to 4
% Maximum amplitude
% RMS value
disp('20*log10(k)=>');
k = 20*log10(k)
bits = input('input number of bits =>');
lg = length(sig);
for x=1:lg
[Index(x) pq]=biquant(bits, -5,5, sig(x));
%Output quantized index.
end
% transmitted
% received
for x=1:lg
qsig(x) = biqtdec(bits, -5,5, Index(x));
end
%Recover the quantized value
%Calculate the quantized error
qerr = sig-qsig;
subplot(3,1,1);plot(t,sig);
ylabel('Original speech');title(we.dat: we');
subplot(3,1,2);stairs(t, qsig);grid
ylabel('Quantized speech')
subplot(3,1,3);stairs(t, qerr);grid
ylabel('Quantized error’)
xlabel('Time (sec.)');axis([0 0.25 –1 1]);
disp('signal to noise ratio due to quantization noise')
snr(sig,qsig)
% Signal to noise ratio in dB:
% sig = original signal vector,
% qsig =quantized signal vector](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8023c736-00ce-4dfb-a41d-61b89c77978b%2F54ee0a7c-a668-47b1-a6ea-00f47c5854df%2Fajwfa2j_processed.jpeg&w=3840&q=75)
Transcribed Image Text:(a) Program 2.2 MATLAB program for Example 2.14.
%Example 2.14
clear all; close all
disp('load speech: We');
load we.dat
sig = we;
fs=8000;
% Load speech data at the current folder
% Provided by the instructor
% Sampling rate
% Length of signal vector
% Sampling period
lg=length(sig);
T=1/fs;
Co
CHAPTER 2 SIGNAL SAMPLING AND QUANTIZATION
EXAMPLE 2.14–CONT'D
t=[0:1:lg-1]*T;
sig=4.5*sig/max(abs(sig));
Xmax = max(abs(sig));
Xrms = sqrt( sum(sig .* sig) / length(sig))
disp('Xrms/Xmax’)
k=Xrms/Xmax
% Time instants in second
% Normalizes speech in the range from -4.5 to 4
% Maximum amplitude
% RMS value
disp('20*log10(k)=>');
k = 20*log10(k)
bits = input('input number of bits =>');
lg = length(sig);
for x=1:lg
[Index(x) pq]=biquant(bits, -5,5, sig(x));
%Output quantized index.
end
% transmitted
% received
for x=1:lg
qsig(x) = biqtdec(bits, -5,5, Index(x));
end
%Recover the quantized value
%Calculate the quantized error
qerr = sig-qsig;
subplot(3,1,1);plot(t,sig);
ylabel('Original speech');title(we.dat: we');
subplot(3,1,2);stairs(t, qsig);grid
ylabel('Quantized speech')
subplot(3,1,3);stairs(t, qerr);grid
ylabel('Quantized error’)
xlabel('Time (sec.)');axis([0 0.25 –1 1]);
disp('signal to noise ratio due to quantization noise')
snr(sig,qsig)
% Signal to noise ratio in dB:
% sig = original signal vector,
% qsig =quantized signal vector
![Program 2.3. MATLAB function for uniform quantization encoding.
function [ I, pq]= biquant(NoBits,Xmin, Xmax,value)
% function pq = biquant(NoBits, Xmin, Xmax, value)
% This routine is created for simulation of uniform quantizer.
% NoBits: number of bits used in quantization.
% Xmax: overload value.
% Xmin: minimum value
% value: input to be quantized.
% pq: output of quantized value
% I: coded integer index
L=2^NoBits;
delta=(Xmax - Xmin)/L;
I=round ((value-Xmin)/delta);
if ( I==L)
I=I-1;
end
if I<0
I=0;
end
pq=Xmin+I*delta;
Program 2.4. MATLAB function for uniform quantization decoding.
function pq =biqtdec(NoBits,Xmin,Xmax,I)
% function pq = biqtdec(NoBits,Xmin, Xmax, I)
% This routine recover the quantized value.
%
% NoBits: number of bits used in quantization.
% Xmax: overload value
% Xmin: minimum value
% pq: output of quantized value
% I: coded integer index
L=2°NoBits;
delta=(Xmax - Xmin)/L;
pq=Xmin+I*delta;
Program 2.5. MATLAB function for calculation of signal-to-quantization noise ratio.
function snr = calcsnr(speech, qspeech)
% function snr = calcsnr(speech, qspeech)
% this routine is created for calculation of SNR
% speech: original speech waveform.
% qspeech: quantized speech.
% snr: output SNR in dB.
qerr = speech-qspeech;
snr = 10*1og10(s um(speech.*speech)/sum(qerr.*qerr))](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8023c736-00ce-4dfb-a41d-61b89c77978b%2F54ee0a7c-a668-47b1-a6ea-00f47c5854df%2Fxc3iia9_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Program 2.3. MATLAB function for uniform quantization encoding.
function [ I, pq]= biquant(NoBits,Xmin, Xmax,value)
% function pq = biquant(NoBits, Xmin, Xmax, value)
% This routine is created for simulation of uniform quantizer.
% NoBits: number of bits used in quantization.
% Xmax: overload value.
% Xmin: minimum value
% value: input to be quantized.
% pq: output of quantized value
% I: coded integer index
L=2^NoBits;
delta=(Xmax - Xmin)/L;
I=round ((value-Xmin)/delta);
if ( I==L)
I=I-1;
end
if I<0
I=0;
end
pq=Xmin+I*delta;
Program 2.4. MATLAB function for uniform quantization decoding.
function pq =biqtdec(NoBits,Xmin,Xmax,I)
% function pq = biqtdec(NoBits,Xmin, Xmax, I)
% This routine recover the quantized value.
%
% NoBits: number of bits used in quantization.
% Xmax: overload value
% Xmin: minimum value
% pq: output of quantized value
% I: coded integer index
L=2°NoBits;
delta=(Xmax - Xmin)/L;
pq=Xmin+I*delta;
Program 2.5. MATLAB function for calculation of signal-to-quantization noise ratio.
function snr = calcsnr(speech, qspeech)
% function snr = calcsnr(speech, qspeech)
% this routine is created for calculation of SNR
% speech: original speech waveform.
% qspeech: quantized speech.
% snr: output SNR in dB.
qerr = speech-qspeech;
snr = 10*1og10(s um(speech.*speech)/sum(qerr.*qerr))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
Recommended textbooks for you
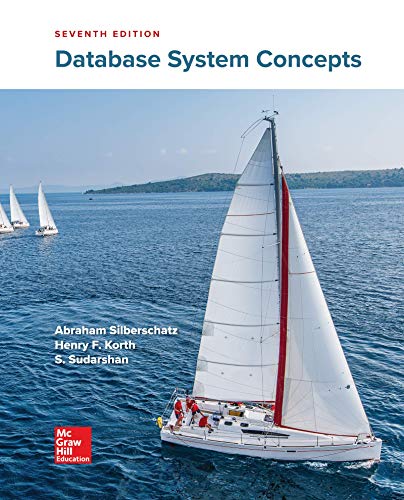
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
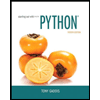
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
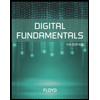
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
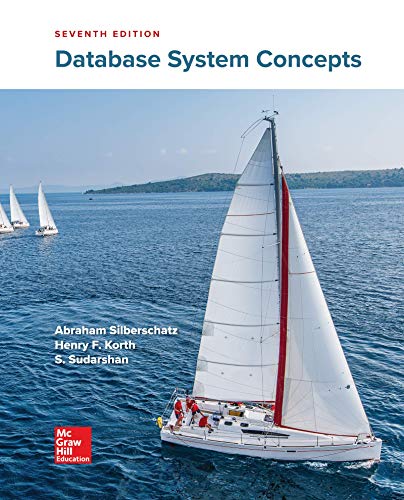
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
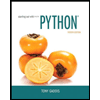
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
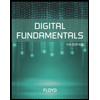
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
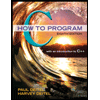
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
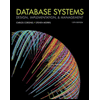
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
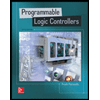
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education