Procedure: 1. Develop a simple program for an individual savings account. Create two (2) classes named SavingsAccount (no class modifier) and RunSavingsAccount (public). 2. In the SavingsAccount class, declare/Initialize variables based on the table below.. Data Type double double Access Level Static? Variable Name balance interestRate Value None private No public Yes 3. Within the constructor, initialize balance with the value of 0. 4. Declare a static setter method named setInterestRate with a parameter of double type named newRate. This method should assign newRate to interestRate. All methods in the program should be public. 5. Add a static method named getInterestRate and a non-static method named getBalance, where each returns an appropriate variable. Both should of double type. 6. Declare a void method named deposit with a parameter of double type named amount. This method should update balance by adding an amount to it. 7. Add another method of double type named withdraw with a parameter of double type also named as amount. Create an if-else statement based on this condition: If balance is greater than or equal than amount, deduct amount from balance; else, amount is equal to 0. Return the amount afterwards. 8. Add a void method named addInterest. Within this method, declare a double variable named interest that accepts the product of balance and interestRate. Update balance by adding interest to it. 9. Declare a void static method named showBalance with a parameter of SavingsAccount type named account. This method should display the current balance of the account by calling the getBalance() method using the object account. 10. Move to the other class, RunSavingsAccount. Import the Scanner class for the user input. 11. Instantiate a SavingsAccount object named savings in the main method to use the methods you have created earlier. This should be the expected sequence of the program upon execution: a. Ask the user to input the interest rate. b. Ask the user to type an amount to be deposited. c. Ask the user to press either D for another deposit or W for withdraw. Show balance afterward. If savings is greater than 1000, display the new balance with applied interest.
Procedure: 1. Develop a simple program for an individual savings account. Create two (2) classes named SavingsAccount (no class modifier) and RunSavingsAccount (public). 2. In the SavingsAccount class, declare/Initialize variables based on the table below.. Data Type double double Access Level Static? Variable Name balance interestRate Value None private No public Yes 3. Within the constructor, initialize balance with the value of 0. 4. Declare a static setter method named setInterestRate with a parameter of double type named newRate. This method should assign newRate to interestRate. All methods in the program should be public. 5. Add a static method named getInterestRate and a non-static method named getBalance, where each returns an appropriate variable. Both should of double type. 6. Declare a void method named deposit with a parameter of double type named amount. This method should update balance by adding an amount to it. 7. Add another method of double type named withdraw with a parameter of double type also named as amount. Create an if-else statement based on this condition: If balance is greater than or equal than amount, deduct amount from balance; else, amount is equal to 0. Return the amount afterwards. 8. Add a void method named addInterest. Within this method, declare a double variable named interest that accepts the product of balance and interestRate. Update balance by adding interest to it. 9. Declare a void static method named showBalance with a parameter of SavingsAccount type named account. This method should display the current balance of the account by calling the getBalance() method using the object account. 10. Move to the other class, RunSavingsAccount. Import the Scanner class for the user input. 11. Instantiate a SavingsAccount object named savings in the main method to use the methods you have created earlier. This should be the expected sequence of the program upon execution: a. Ask the user to input the interest rate. b. Ask the user to type an amount to be deposited. c. Ask the user to press either D for another deposit or W for withdraw. Show balance afterward. If savings is greater than 1000, display the new balance with applied interest.
Chapter5: Making Decisions
Section: Chapter Questions
Problem 8PE
Related questions
Question
Objective: Create a program that invokes static methods and uses static variables.
Note: Please see attached pictures. Also please send a screenshot of the code (Java

Transcribed Image Text:Procedure:
1. Develop a simple program for an individual savings account. Create two (2) classes named
SavingsAccount (no class modifier) and RunSavingsAccount (public).
2. In the SavingsAccount class, declare/Initialize variables based on the table below.
Data Type
double
double
Access Level
private
public
Static?
Variable Name
balance
interestRate
Value
No
None
Yes
3. Within the constructor, initialize balance with the value of 0.
4. Declare a static setter method named setInterestRate with a parameter of double type named
newRate. This method should assign newRate to interestRate. All methods in the program should
be public.
5. Add a static method named getInterestRate and a non-static method named getBalance, where
each returns an appropriate variable. Both should of double type.
6. Declare a void method named deposit with a parameter of double type named amount. This
method should update balance by adding an amount to it.
7. Add another method of double type named withdraw with a parameter of double type also named
as amount. Create an if-else statement based on this condition: If balance is greater than or equal
than amount, deduct amount from balance; else, amount is equal to 0. Return the amount
afterwards.
8. Add a void method named addInterest. Within this method, declare a double variable named
interest that accepts the product of balance and interestRate. Update balance by adding
interest to it.
9. Declare a void static method named showBalance with a parameter of SavingsAccount type named
account. This method should display the current balance of the account by calling the
getBalance() method using the object account.
10. Move to the other class, RunSavingsAccount. Import the Scanner class for the user input.
11. Instantiate a SavingsAccount object named savings in the main method to use the methods you
have created earlier. This should be the expected sequence of the program upon execution:
a. Ask the user to input the interest rate.
b. Ask the user to type an amount to be deposited.
c. Ask the user to press either D for another deposit or W for withdraw. Show balance
afterward. If savings is greater than 1000, display the new balance with applied interest.

Transcribed Image Text:Sample Output:
Enter interest rate: .10
Enter deposit amount: 500
Your balance is 500.0
Press D for another deposit or W to withdraw: d
Enter deposit amount: 1000
Your new balance is 1650.0
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 9 images

Recommended textbooks for you
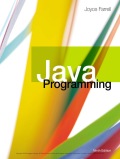
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
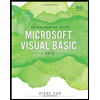
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
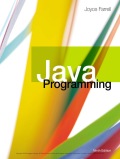
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
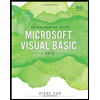
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning