Problem 2-Python We have a dictionary where the keys are the names of all your relatives and the values are their ages. Write a Python program to iterate/loop over the dictionary and find the oldest member of your family. Sample output: The oldest relative is Helko. Recall: Iterating over a dictionary iterates over its KEYS. HINT: First we initialize maximum_age to 0 and maximum_age_relative to and empty string. These variables will be updated during the loop. Then we need to iterate over the keys of the dictionary my_family to find the member and their age. If the age is greater than the current maximum_age, we update maximum_age with this new maximum age. Then we update the maximum_age_relative with the new member. Given Code: my_family = {'Bob':72, 'Susan':39, 'Helko':81, 'Gertrude':4} maximum_age = 0 maximum_age_relative = ""
Problem 2-Python
We have a dictionary where the keys are the names of all your relatives and the values are their ages. Write a Python program to iterate/loop over the dictionary and find the oldest member of your family.
Sample output: The oldest relative is Helko.
Recall: Iterating over a dictionary iterates over its KEYS.
HINT: First we initialize maximum_age to 0 and maximum_age_relative to and empty string. These variables will be updated during the loop.
Then we need to iterate over the keys of the dictionary my_family to find the member and their age.
If the age is greater than the current maximum_age, we update maximum_age with this new maximum age. Then we update the maximum_age_relative with the new member.
Given Code:
my_family = {'Bob':72, 'Susan':39, 'Helko':81, 'Gertrude':4}
maximum_age = 0
maximum_age_relative = ""

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

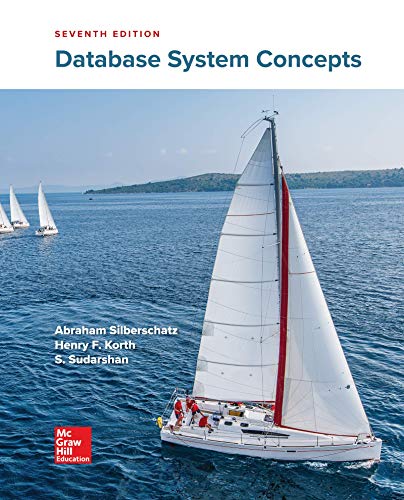
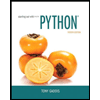
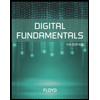
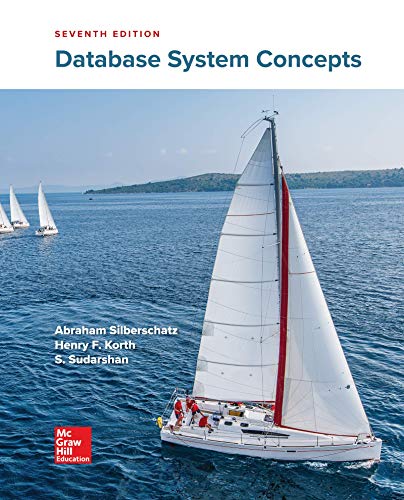
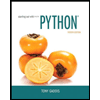
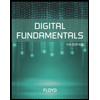
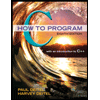
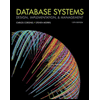
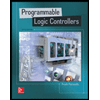