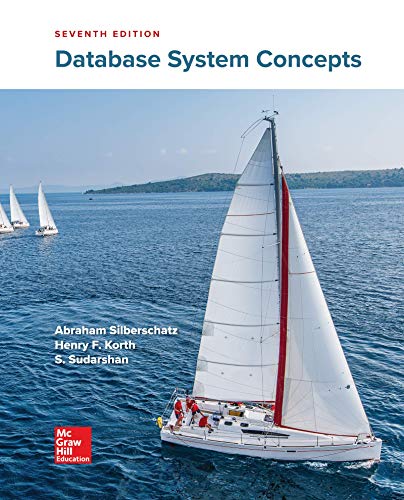
Problem 1: Consider an array-based queue implementation. Suppose we wish to use an extra bit
in queue records to indicate whether a queue is empty.
1. Modify the declarations and operations for a circular queue to accommodate this feature.
2. Would you expect the change to be worthwhile?
Problem 2: Consider an array-based queue implementation. A variant of the circular queue
records the position of the front element and the length of the queue.
1. Is it necessary in this implementation to limit the length of a queue to maxlength - 1?
2. Write the five queue operations for this implementation.
3. Compare this implementation with the circular queue implementation discussed in class.
Problem 3: A dequeue (double-ended queue) is a list from which elements can be inserted or
deleted at either end.
1. Develop an array-based implementation for dequeue.
2. Develop a pointer-based implementation for dequeue.

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Algorithms and Data Structures in Java.The exercise consists,Queues. Note you are notallowed to rely on Java library classes in your implementation.Part 1Implement in Java the Queue abstract data type using a singly-linked list with only one accesspointer (instead of two: Q.head and Q.tail). ENQUEUE and DEQUEUE operations should takeconstant time. Assume elements are integers. and please Briefly describe your implementation.arrow_forwardShow the contents of the queue, from front to back, after the execution of the following segment of code, given that queue is an object that fits our abstract description of a queue (originally empty). Assume that you can store and retrieve variables of type int on queue. queue.enqueue(100); for (int i = 1; i <= 3; i++) { qиеue.enqueие (і * 4)); queue.dequeue(); }arrow_forwardplease answer with proper explanation and step by step solution. don't copy paste from online website. i would appreciate your work. Question: What are the running times of each of the functions of the standard priority queue ADT if we implement it by adapting the STL priority_queue. Please answer thoroughly with examples while also using the Big O notation in it.arrow_forward
- c) Given a Python Queue class implemented with the abstract data type (ADT) below, write a new method popqueue(), which will “pop" (remove and return) the last-in element from the rear end of the queue. | Operation (Queue) Description sizeQ():int | GIVEN: Get (and return) the size of the queue (total number of elements) enqueue (elt): dequeue (): elt popqueue(): elt Remove (and return) the last-in element from rear end of Queue GIVEN: Insert elt into Queue GIVEN: Remove (and return) element from Queue Finish this new Python popqueue () method, by completing the method body below: def popqueue ( self ): # remove and return rear elt # body of the method. TO BE COMPLETED by student o No other extra variable could be added including Python's list, except int and range with function range(). o No more than 4 lines of typical python code (without semicolon symbol ;) allowed in the method body. * Hint: you may finish this task using its own given methods of the Queue class, and a for loop…arrow_forward5. Given an efficient circular bent array-based queue q capable of holding 7 objects. Show the final contents of the array after the following code is executed: for (int k = 1; k <= 6; k++) q.enqueue(k); for (int k = 1; k <= 3; k++) { q.dequeue(); q.enqueue(q.dequeue()); } 0 1 2 3 4 5 6arrow_forward1. consider an array based queue implementation. suppose we wish to use an extra bit in the queue records to indicate whether a queue is empty. a. Modify the declarationsand operations for a circular queue to accomodate this feature. b. Would you see the change to be worthwide.arrow_forward
- 29The Java interface construct is used to create a formal specification of our Queue ADT. T OR Farrow_forwarda) Given a typical Queue q with elements [E,D,C,B,A] (where Front/left of Queue at E and Rear/right of Queue at A), determine and list elements of the updated Queue in the similar form (Front/left and Rear/right), after the following execution: q.enqueue( q. dequeue() ) q. enqueue( G ) q.dequeue() b) Given a typical Stack s with elements [T,W,X,Y,Z] (where Top/left of Stack at T) and a typical Queue q with elements [E,D,C,B,A] (where Front/left of Queue at E and Rear/right of Queue at A), determine and list elements of the updated Stack and those of the updated Queue in their similar forms after the following execution: q. enqueue( s.pop() ) s.push( R ) q.enqueue( s. peek () ) s. push( q.dequeue() ) q.enqueue( s. pop() ) OParrow_forwardIf the sequence number space is k bits large, you should seriously consider using both the selective-repeat technique and the Go-Back-N strategy in your search for a solution. What is the maximum allowable size for the sender window that we are allowed to make use of?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
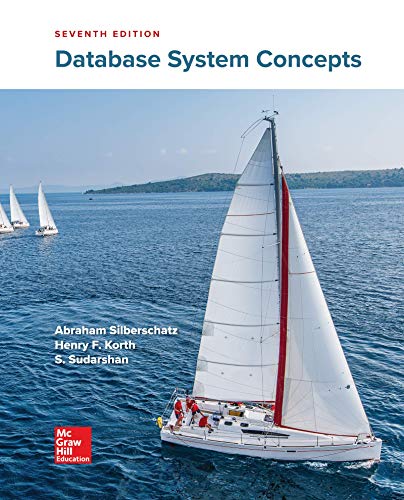
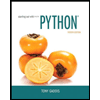
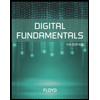
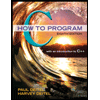
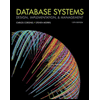
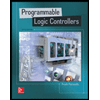