Print first 2 Fib values (0, 1) before starting These are hard-coded since they are starting values...no calculations required Enter MainLoop Loop and call subroutines to calculate each subsequent value in the sequence Exit the loop once all single-digit values in the sequence have been printed USE 3 subroutines calcNextFib Uses R1 and R2 to calc next value in Fibonacci sequence printNum Outputs single-digit number to the display printCommaSpace Outputs a comma and a spaceCalculate all the single-digit Fibonacci numbers in order Start with the first 2 values in the sequence (0, 1) Calculate the next value by adding together the two largest values Print each number in the sequence With a comma and space after each No comma after the last number Use LC-3 I/O device to print number, commas, and spaces NO numbers printed using Trap Routine should check ddr, dsr , load and store modify the code to work using ALL Guidelines For each Calculation: ; Fib2 = Fib1 + Fib2 ; Fib1 = previous Fib2 ; R0 used for PUTS messages ; R1 contains current Fib1 value ; R2 contains current Fib2 value ; R6 is loop counter for main loop ; R7 is not used by this program. Used by Simulate for TRAP and Subroutines .ORIG x3000 Setup LEA R0, Info PUTS ADD R6, R6, #5; Init Main Loop counter (loop 5 times) ;Print first 2 Fib values before starting ;----- Your Code Here -----; ;----- End Your Code Here -----; ;Loop and call subroutines to calculate each subsequent value in the sequence ;Exit the loop once all single-digit values in the sequence have been printed MainLoop ;----- Your Code Here -----; ;----- End Your Code Here -----; Done HALT ;----------------------------------- ;Subroutines ;----------------------------------- ;Uses R1 and R2 to calc next value in Fibonacci sequence ;When complete, Fib2 (R2) will contain the new Fib number ;and Fib1 (R1) will contain the previous value of Fib2 (R2) calcNextFib ;----- Your Code Here -----; ;----- End Your Code Here -----; RET ;Outputs single-digit number to the display ;R2 contains number to print printNum ;----- Your Code Here -----; ;----- End Your Code Here -----; RET ;Outputs a comma and a space ;No data is passed in and no data is returned printCommaSpace ;----- Your Code Here -----; ;----- End Your Code Here -----; RET ;End of Program ;Data Declarations------------- DSR .FILL xFE04 DDR .FILL xFE06 Info .STRINGZ "This program will print the first 6 characters of the Fibboncci Sequence\n" ASCIIOFSET .FILL x0030 NegASCIIOFSET .FILL xFFD0 ASCIINewline .FILL x000d ; Newline ascii code ASCIISpace .FILL x0020 ; Space ascii code ASCIIComma .FILL x002C ; Comma ascii code ; Memory slots for subrountines to store/restore registers ; You may or may not need to use all of these SaveR3 .BLKW 1 SaveR4 .BLKW 1 SaveR5 .BLKW 1 SaveR6 .BLKW 1 . .END
- Print first 2 Fib values (0, 1) before starting
- These are hard-coded since they are starting values...no calculations required
- Enter MainLoop
- Loop and call subroutines to calculate each subsequent value in the sequence
- Exit the loop once all single-digit values in the sequence have been printed
- USE 3 subroutines
- calcNextFib Uses R1 and R2 to calc next value in Fibonacci sequence
- printNum Outputs single-digit number to the display
- printCommaSpace Outputs a comma and a spaceCalculate all the single-digit Fibonacci numbers in order
- Start with the first 2 values in the sequence (0, 1)
- Calculate the next value by adding together the two largest values
- Print each number in the sequence
- With a comma and space after each
- No comma after the last number
- Use LC-3 I/O device to print number, commas, and spaces
- NO numbers printed using Trap Routine
- should check ddr, dsr , load and store
modify the code to work using ALL Guidelines
For each Calculation:
; Fib2 = Fib1 + Fib2 ; Fib1 = previous Fib2
; R0 used for PUTS messages
; R1 contains current Fib1 value
; R2 contains current Fib2 value
; R6 is loop counter for main loop
; R7 is not used by this program. Used by Simulate for TRAP and Subroutines
.ORIG x3000
Setup
LEA R0, Info
PUTS
ADD R6, R6, #5; Init Main Loop counter (loop 5 times)
;Print first 2 Fib values before starting
;----- Your Code Here -----;
;----- End Your Code Here -----;
;Loop and call subroutines to calculate each subsequent value in the sequence
;Exit the loop once all single-digit values in the sequence have been printed
MainLoop
;----- Your Code Here -----;
;----- End Your Code Here -----;
Done
HALT
;----------------------------------- ;Subroutines ;-----------------------------------
;Uses R1 and R2 to calc next value in Fibonacci sequence
;When complete, Fib2 (R2) will contain the new Fib number
;and Fib1 (R1) will contain the previous value of Fib2 (R2)
calcNextFib
;----- Your Code Here -----;
;----- End Your Code Here -----;
RET
;Outputs single-digit number to the display
;R2 contains number to
print printNum
;----- Your Code Here -----;
;----- End Your Code Here -----;
RET
;Outputs a comma and a space
;No data is passed in and no data is returned
printCommaSpace
;----- Your Code Here -----;
;----- End Your Code Here -----;
RET
;End of Program
;Data Declarations-------------
DSR .FILL xFE04
DDR .FILL xFE06
Info .STRINGZ "This program will print the first 6 characters of the Fibboncci Sequence\n"
ASCIIOFSET .FILL x0030
NegASCIIOFSET .FILL xFFD0
ASCIINewline .FILL x000d ; Newline ascii code
ASCIISpace .FILL x0020 ; Space ascii code
ASCIIComma .FILL x002C ; Comma ascii code
; Memory slots for subrountines to store/restore registers
; You may or may not need to use all of these
SaveR3 .BLKW 1
SaveR4 .BLKW 1
SaveR5 .BLKW 1
SaveR6 .BLKW 1 .
.END

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

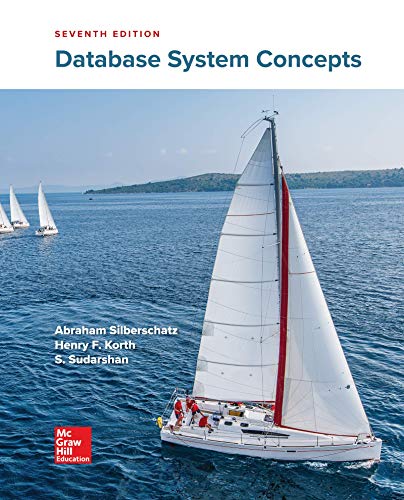
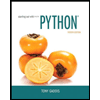
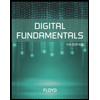
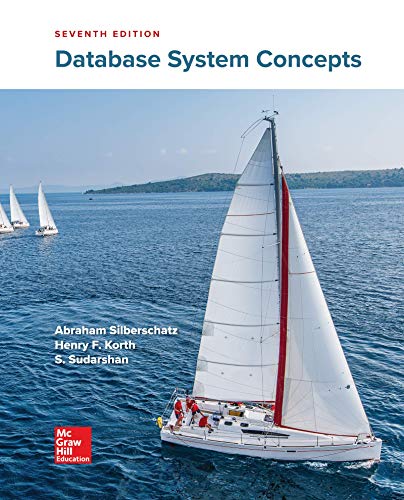
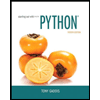
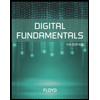
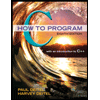
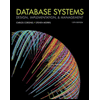
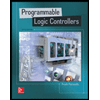