PLEASE USE C++ AND CORRECT THE CODE ERROR USING YELLOW HIGHLIGHTED ERRORS IN ATTACHED PHOTO Program Specifications: Write a program to calculate U.S. income tax owed given wages, taxable interest, unemployment compensation, status (single or married), and taxes withheld. Taxpayers are only allowed to use this short form if adjusted gross income (AGI) is less than $120000. Dollar amounts are displayed as integers with no comma separators. For example, cout << "Deduction: $" << deduction Note: this program is designed for incremental development. Complete each step and submit it for grading before starting the next step. Only a portion of tests pass after each step but confirm progress. Step 1. Input wages, taxable interest, unemployment compensation, status (1=single and 2=married), and taxes withheld as integers. Calculate and output AGI (wages + interest + unemployment). Output error message if AGI is above $120000 and the program stops with no additional output. Submit for grading to confirm two tests pass. Ex: If the input is: 20000 23 500 1 400 The output is: AGI: $20523 Ex: If the input is: 120000 23 500 1 400 The output is: AGI: $120523 Error: Income too high to use this form Step 2. Identify deduction amount based on status: (1) Single=$12000 or (2) Married=$24000. Set status to 1 if not input as 1 or 2. Calculate taxable income (AGI - deduction). Set taxable income to zero if negative. Output deduction and taxable income. Submit for grading to confirm five tests pass. Ex: If the input is: 20000 23 500 1 400 Ex: The additional output is: AGI: $20523 Deduction: $12000 Taxable income: $8523 Step 3. Calculate tax amount based on exemption and taxable income (see tables below). The tax amount should be stored as a double and rounded to the nearest whole number using round(). Submit for grading to confirm eight tests pass. Ex: If the input is: 20000 23 500 1 400 Ex: The additional output is: AGI: $20523 Deduction: $12000 Taxable income: $8523 Federal tax: $852 Income Tax for Single Filers $0 - $10000 10% of the income $10001 - $40000 $1000 + 12% of the amount over $10000 $40001 - $85000 $4600 + 22% of the amount over $40000 over $85000 $14500 + 24% of the amount over $85000 Income Tax for Married Filers $0 - $20000 10% of the income $20001 - $80000 $2000 + 12% of the amount over $20000 over $80000 $9200 + 22% of the amount over $80000 Step 4. Calculate the amount of tax due (tax - withheld). If the amount due is negative the taxpayer receives a refund. Output tax due or tax refund as positive values. Submit for grading to confirm all tests pass. Ex: If the input is: 80000 0 500 2 12000 Ex: The additional output is: AGI: $80500 Deduction: $24000 Taxable income: $56500 Federal tax: $6380 Tax refund: $5620 CODE: #include #include using namespace std; int main() { int wages, taxable_interest, unemployment_compensation, status, taxes_withheld; cin >> wages >> taxable_interest >> unemployment_compensation >> status >> taxes_withheld; int AGI = wages + taxable_interest + unemployment_compensation; if (AGI > 120000) { cout << "Error: Income too high to use this form" << endl; return 0; } cout << "AGI: $" << AGI << endl; int deduction = (status == 2) ? 24000 : 12000; int taxable_income = max(AGI - deduction, 0); cout << "Deduction: $" << deduction << endl; cout << "Taxable income: $" << taxable_income << endl; double tax_amount = 0.0; if (taxable_income <= 20000) { tax_amount = taxable_income * 0.1; } else if (taxable_income <= 50000) { tax_amount = 2000 + (taxable_income - 20000) * 0.12; } else if (taxable_income <= 75000) { tax_amount = 2000 + 3600 + (taxable_income - 50000) * 0.22; } else { tax_amount = 2000 + 3600 + 5500 + (taxable_income - 75000) * 0.24; } int rounded_tax_amount = round(tax_amount); cout << "Federal tax: $" << rounded_tax_amount << endl; int tax_due_or_refund = rounded_tax_amount - taxes_withheld; if (tax_due_or_refund >= 0) { cout << "Tax due: $" << tax_due_or_refund << endl; } else { cout << "Tax refund: $" << abs(tax_due_or_refund) << endl; } return 0; }
PLEASE USE C++ AND CORRECT THE CODE ERROR USING YELLOW HIGHLIGHTED ERRORS IN ATTACHED PHOTO
Program Specifications: Write a program to calculate U.S. income tax owed given wages, taxable interest, unemployment compensation, status (single or married), and taxes withheld. Taxpayers are only allowed to use this short form if adjusted gross income (AGI) is less than $120000. Dollar amounts are displayed as integers with no comma separators. For example, cout << "Deduction: $" << deduction
Note: this program is designed for incremental development. Complete each step and submit it for grading before starting the next step. Only a portion of tests pass after each step but confirm progress.
Step 1. Input wages, taxable interest, unemployment compensation, status (1=single and 2=married), and taxes withheld as integers. Calculate and output AGI (wages + interest + unemployment). Output error message if AGI is above $120000 and the program stops with no additional output. Submit for grading to confirm two tests pass.
Ex: If the input is:
The output is:
AGI: $20523Ex: If the input is:
120000 23 500 1 400The output is:
AGI: $120523Step 2. Identify deduction amount based on status: (1) Single=$12000 or (2) Married=$24000. Set status to 1 if not input as 1 or 2. Calculate taxable income (AGI - deduction). Set taxable income to zero if negative. Output deduction and taxable income. Submit for grading to confirm five tests pass.
Ex: If the input is:
Ex: The additional output is:
AGI: $20523
Step 3. Calculate tax amount based on exemption and taxable income (see tables below). The tax amount should be stored as a double and rounded to the nearest whole number using round(). Submit for grading to confirm eight tests pass.
Ex: If the input is:
Ex: The additional output is:
AGI: $20523Income | Tax for Single Filers |
---|---|
$0 - $10000 | 10% of the income |
$10001 - $40000 | $1000 + 12% of the amount over $10000 |
$40001 - $85000 | $4600 + 22% of the amount over $40000 |
over $85000 | $14500 + 24% of the amount over $85000 |
Income | Tax for Married Filers |
---|---|
$0 - $20000 | 10% of the income |
$20001 - $80000 | $2000 + 12% of the amount over $20000 |
over $80000 | $9200 + 22% of the amount over $80000 |
Step 4. Calculate the amount of tax due (tax - withheld). If the amount due is negative the taxpayer receives a refund. Output tax due or tax refund as positive values. Submit for grading to confirm all tests pass.
Ex: If the input is:
Ex: The additional output is:
AGI: $80500#include <iostream>
#include <cmath>
using namespace std;
int main() {
int wages, taxable_interest, unemployment_compensation, status, taxes_withheld;
cin >> wages >> taxable_interest >> unemployment_compensation >> status >> taxes_withheld;
int AGI = wages + taxable_interest + unemployment_compensation;
if (AGI > 120000) {
cout << "Error: Income too high to use this form" << endl;
return 0;
}
cout << "AGI: $" << AGI << endl;
int deduction = (status == 2) ? 24000 : 12000;
int taxable_income = max(AGI - deduction, 0);
cout << "Deduction: $" << deduction << endl;
cout << "Taxable income: $" << taxable_income << endl;
double tax_amount = 0.0;
if (taxable_income <= 20000) {
tax_amount = taxable_income * 0.1;
} else if (taxable_income <= 50000) {
tax_amount = 2000 + (taxable_income - 20000) * 0.12;
} else if (taxable_income <= 75000) {
tax_amount = 2000 + 3600 + (taxable_income - 50000) * 0.22;
} else {
tax_amount = 2000 + 3600 + 5500 + (taxable_income - 75000) * 0.24;
}
int rounded_tax_amount = round(tax_amount);
cout << "Federal tax: $" << rounded_tax_amount << endl;
int tax_due_or_refund = rounded_tax_amount - taxes_withheld;
if (tax_due_or_refund >= 0) {
cout << "Tax due: $" << tax_due_or_refund << endl;
} else {
cout << "Tax refund: $" << abs(tax_due_or_refund) << endl;
}
return 0;
}



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

Could you please fix the yellow highlighted errors in attachedment? The expected output is not correct.
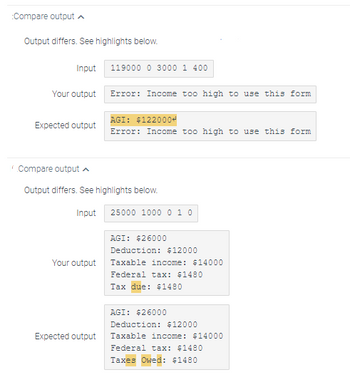
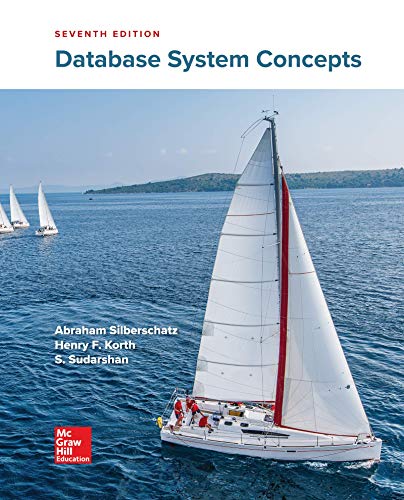
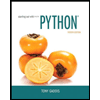
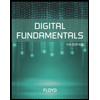
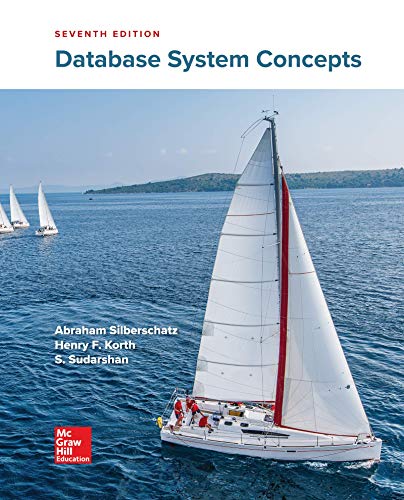
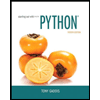
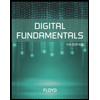
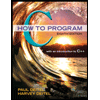
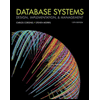
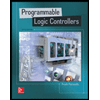