Please help, thank you. Replace the user-specified number of messages to be sent with a variable numMsgs that you can define internally in your program. We will set numMsgs according to the experiments specified below. • Replace the user-specified message string with a string of the size defined by the experiments below. Please utilize the following example that allows us to create a string comprising of null values of a size specified using the variable numBytes. In the following example, we are creating a string called message of a 1000 bytes. We can similarly create a message string of 5000 and 10000 Bytes by changing the value of numBytes. numBytes = 1000 message = ‘\x00’ * numBytes To get the size of this message (needed later as a part of your code output), you may use the getsizeof utility from the system library in Python. Please import the system library as ‘import sys’. The following example shows how to get the size of the message we created earlier: sys.getsizeof(message) Create and use variables, timeBefore and timeAfter, to measure the time BEFORE and AFTER each sendto( ) call sending a message to the server. Use the time( )function in Python to measure the time. This function returns the current time in seconds since the "epoch." The epoch is a reference point in time, and the specific epoch used by Python is the "Unix epoch," which is defined as 00:00:00 Coordinated Universal Time (UTC) on January 1, 1970. When time( ) function is called, it returns a floating-point number representing the number of seconds that have passed since January 1, 1970 (the Unix epoch) to the current time. We can call the time( ) function at multiple points in our program to find the time difference between these points. To use the time function, import the time library as ‘import time’. The following example shows how to store a time value in a variable timeBefore: timeBefore = time.time( ) • Create a variable RTT that measures the round trip time for each message within the message sending loop. • Create a variable SeqNum that reflects the sequence number of each message sent from client to the server. • For each message received from the server in response to a message sent from the client, display a message of the type: Message sequence number with size received from with round trip time seconds. Here’s a sample output from my client code: Message sequence 1 with size 5049 received from ('127.0.0.1', 12001) with RTT 0.0018 s. Message sequence 2 with size 5049 received from ('127.0.0.1', 12001) with RTT 0.0033 s. Message sequence 3 with size 5049 received from ('127.0.0.1', 12001) with RTT 0.0025 s. Message sequence 4 with size 5049 received from ('127.0.0.1', 12001) with RTT 0.0037 s. Message sequence 5 with size 5049 received from ('127.0.0.1', 12001) with RTT 0.0041 s. Here is my code from so far: import socket def udp_echo_client(): # Create a UDP socket client_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) server_address = ('localhost', 65432) # Get user input message = input("Enter a message to send to the server: ") num_times = int(input("How many times would you like to send the message? ")) for _ in range(num_times): # Send data to the server sent = client_socket.sendto(message.encode(), server_address) # Receive response from the server data, server = client_socket.recvfrom(4096) print(f"Received echo: '{data.decode()}' from {server}") if __name__ == "__main__": udp_echo_client()
Please help, thank you.
can define internally in your program. We will set numMsgs according to the experiments
specified below.
• Replace the user-specified message string with a string of the size defined by the
experiments below. Please utilize the following example that allows us to create a string
comprising of null values of a size specified using the variable numBytes.
In the following example, we are creating a string called message of a 1000 bytes. We can
similarly create a message string of 5000 and 10000 Bytes by changing the value of
numBytes.
numBytes = 1000
message = ‘\x00’ * numBytes
To get the size of this message (needed later as a part of your code output), you may use the
getsizeof utility from the system library in Python. Please import the system library as ‘import
sys’. The following example shows how to get the size of the message we created earlier:
sys.getsizeof(message)
each sendto( ) call sending a message to the server.
Use the time( )function in Python to measure the time. This function returns the current time
in seconds since the "epoch." The epoch is a reference point in time, and the specific epoch
used by Python is the "Unix epoch," which is defined as 00:00:00 Coordinated Universal
Time (UTC) on January 1, 1970. When time( ) function is called, it returns a floating-point
number representing the number of seconds that have passed since January 1, 1970 (the
Unix epoch) to the current time. We can call the time( ) function at multiple points in our
program to find the time difference between these points.
To use the time function, import the time library as ‘import time’. The following example
shows how to store a time value in a variable timeBefore:
timeBefore = time.time( )
• Create a variable RTT that measures the round trip time for each message within the
message sending loop.
• Create a variable SeqNum that reflects the sequence number of each message sent from
client to the server.
• For each message received from the server in response to a message sent from the client,
display a message of the type: Message sequence number <seqNum> with size <message
size> received from <server address> with round trip time <RTT> seconds.
Here’s a sample output from my client code:
Message sequence 1 with size 5049 received from ('127.0.0.1', 12001) with RTT 0.0018 s.
Message sequence 2 with size 5049 received from ('127.0.0.1', 12001) with RTT 0.0033 s.
Message sequence 3 with size 5049 received from ('127.0.0.1', 12001) with RTT 0.0025 s.
Message sequence 4 with size 5049 received from ('127.0.0.1', 12001) with RTT 0.0037 s.
Message sequence 5 with size 5049 received from ('127.0.0.1', 12001) with RTT 0.0041 s.
Here is my code from so far:
import socket
def udp_echo_client():
# Create a UDP socket
client_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
server_address = ('localhost', 65432)
# Get user input
message = input("Enter a message to send to the server: ")
num_times = int(input("How many times would you like to send the message? "))
for _ in range(num_times):
# Send data to the server
sent = client_socket.sendto(message.encode(), server_address)
# Receive response from the server
data, server = client_socket.recvfrom(4096)
print(f"Received echo: '{data.decode()}' from {server}")
if __name__ == "__main__":
udp_echo_client()

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

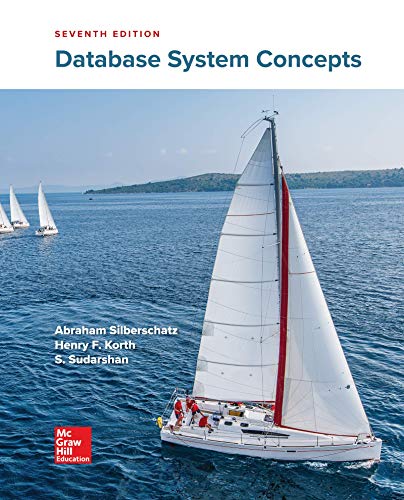
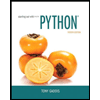
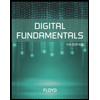
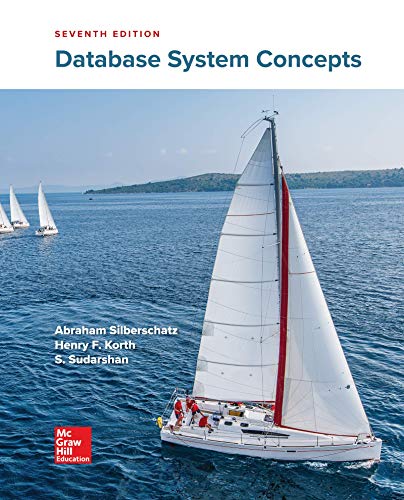
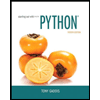
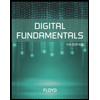
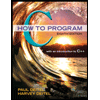
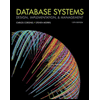
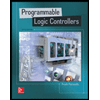