Please help me implement this C++ function that is supposed to partition the array a into smaller than pivot and others. // f is the beginning of the section to be partitioned // r is the end of the section to be partitioned // return the first slot of the Large section int partition(int a[], int f, int r) { int pivot = a[f]; // pivot is the first element int small = f; // small pointer from the left int large = r; // large pointer from the right while (small <= large) //until they cross { // loop for finding out-of-place pairs and swap them // until the small and large cross // you will be checking a[small] and a[large] against pivot // if both are bad (and not crossed yet), swap and then move // if a[small] is OK, move small // if a[large] is OK, move large // TODO: ** } //end of while // return the partition point where // those smaller than pivot are before what is returned // there is a special cases (small is at the beginning) // and a regular case // TODO: }
Please help me implement this C++ function that is supposed to partition the array a into smaller than pivot and others.
// f is the beginning of the section to be partitioned
// r is the end of the section to be partitioned
// return the first slot of the Large section
int partition(int a[], int f, int r) {
int pivot = a[f];
// pivot is the first element
int small = f;
// small pointer from the left
int large = r;
// large pointer from the right
while (small <= large)
//until they cross
{
// loop for finding out-of-place pairs and swap them
// until the small and large cross
// you will be checking a[small] and a[large] against pivot
// if both are bad (and not crossed yet), swap and then move
// if a[small] is OK, move small
// if a[large] is OK, move large
// TODO: **
}
//end of while
// return the partition point where
// those smaller than pivot are before what is returned
// there is a special cases (small is at the beginning)
// and a regular case
// TODO:
}
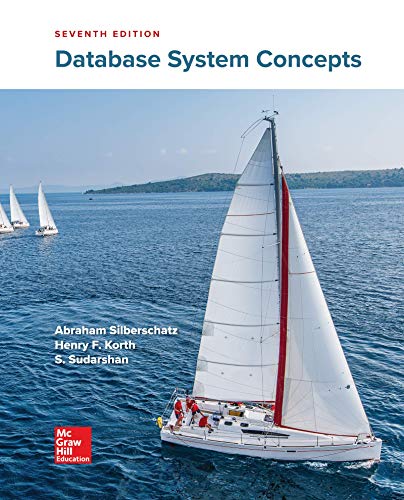
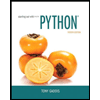
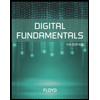
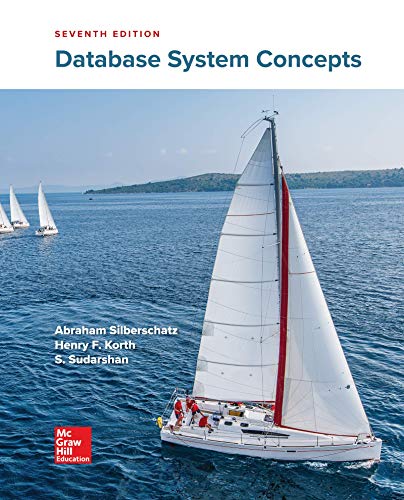
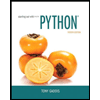
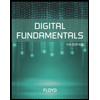
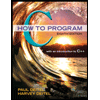
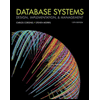
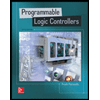