Please help add a data validation (ex. let the user only input int) using exception handling class Rectangle: res = 0 res1 = 0 vol = 0 def __init__(self, length, width): self.length = length self.width = width def perimeter(self): self.res = 2 * ((self.length + self.width)) def area(self): self.res1 = self.length * self.width def display(self): print("\n\nLength of a rectangle: ", self.length) print("Width of a rectangle: ", self.width) Rectangle.perimeter(self) print("Perimeter of a rectangle: ", self.res) Rectangle.area(self) print("Area of a rectangle: ", self.res1) class Parallelepipede(Rectangle): def __init__(self, length, width, height): super().__init__(length,width) self.height = height def volume(self): self.vol = self.length * self.width * self.height def display(self): print("Height of a parallelepipede: ", self.height) Parallelepipede.volume(self) print("Volume of parallelepipede: ", self.vol) if __name__ == "__main__": length=eval(input("Length?: ")) width=eval(input("Width?: ")) height=eval(input("Height?: ")) r = Rectangle(length, width) r.display() p = Parallelepipede(length, width, height) p.display()
Please help add a data validation (ex. let the user only input int) using exception handling class Rectangle: res = 0 res1 = 0 vol = 0 def __init__(self, length, width): self.length = length self.width = width def perimeter(self): self.res = 2 * ((self.length + self.width)) def area(self): self.res1 = self.length * self.width def display(self): print("\n\nLength of a rectangle: ", self.length) print("Width of a rectangle: ", self.width) Rectangle.perimeter(self) print("Perimeter of a rectangle: ", self.res) Rectangle.area(self) print("Area of a rectangle: ", self.res1) class Parallelepipede(Rectangle): def __init__(self, length, width, height): super().__init__(length,width) self.height = height def volume(self): self.vol = self.length * self.width * self.height def display(self): print("Height of a parallelepipede: ", self.height) Parallelepipede.volume(self) print("Volume of parallelepipede: ", self.vol) if __name__ == "__main__": length=eval(input("Length?: ")) width=eval(input("Width?: ")) height=eval(input("Height?: ")) r = Rectangle(length, width) r.display() p = Parallelepipede(length, width, height) p.display()
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Please help add a data validation (ex. let the user only input int) using exception handling
class Rectangle:
res = 0
res1 = 0
vol = 0
def __init__(self, length, width):
self.length = length
self.width = width
def perimeter(self):
self.res = 2 * ((self.length + self.width))
def area(self):
self.res1 = self.length * self.width
def display(self):
print("\n\nLength of a rectangle: ", self.length)
print("Width of a rectangle: ", self.width)
Rectangle.perimeter(self)
print("Perimeter of a rectangle: ", self.res)
Rectangle.area(self)
print("Area of a rectangle: ", self.res1)
class Parallelepipede(Rectangle):
def __init__(self, length, width, height):
super().__init__(length,width)
self.height = height
def volume(self):
self.vol = self.length * self.width * self.height
def display(self):
print("Height of a parallelepipede: ", self.height)
Parallelepipede.volume(self)
print("Volume of parallelepipede: ", self.vol)
if __name__ == "__main__":
length=eval(input("Length?: "))
width=eval(input("Width?: "))
height=eval(input("Height?: "))
r = Rectangle(length, width)
r.display()
p = Parallelepipede(length, width, height)
p.display()
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
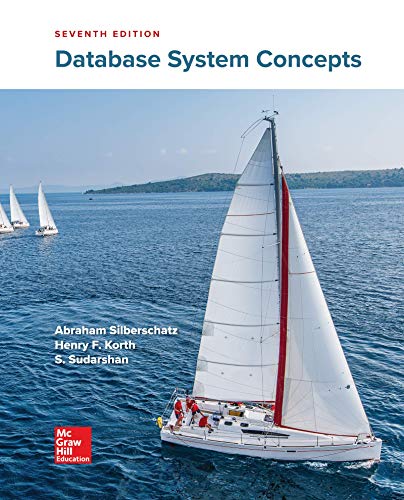
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
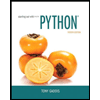
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
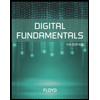
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
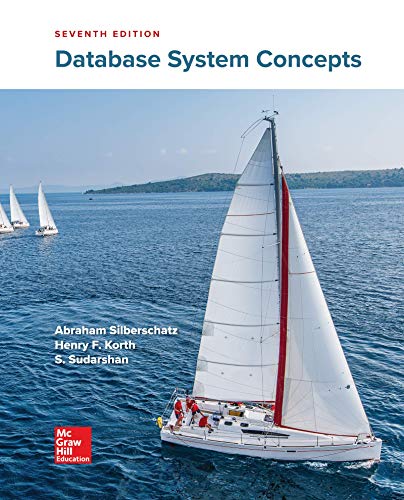
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
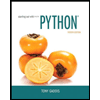
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
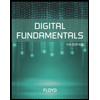
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
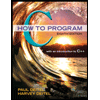
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
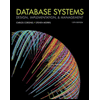
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
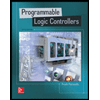
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education