Please follow the instructions in the screenshots provided and use that to implement the code given below. Done in python 3.10 or later please. class WeightedAdjacencyMatrix : """A weighted graph represented as a matrix.""" __slots__ = ['_W'] def __init__(self, size, edges=[], weights=[]) : """Initializes a weighted adjacency matrix for a graph with size nodes. Graph is initialized with size nodes and a specified set of edges and edge weights. Keyword arguments: size -- Number of nodes of the graph. edges -- a list of ordered pairs (2-tuples) indicating the edges of the graph. The default value is an empty list which means no edges by default. weights -- a list of weights for the edges, which should be the same length as the edges list. The position of a value in the weights list corresponds to the edge in the same position of the edges list. """ pass # replace this pass statement with the code needed to implement this def add_edge(self, u, v, weight) : """Adds an undirected edge between u to v with the specified weight. Keyword arguments: u -- vertex id (0-based index) v -- vertex id (0-based index) weight -- edge weight """ pass # replace this pass statement with the code needed to implement this def floyd_warshall(self) : """Floyd Warshall algorithm for all pairs shortest paths. Returns a matrix D consisting of the weights of the shortest paths between all pairs of vertices, and a matrix P for the predecessors matrix (what the textbook called PI). This method MUST NOT change the weight matrix of the graph itself. """ # Your return statement will look something like this one # in the comment on the following line. That returns # the two matrices, with the D matrix first. The return None # is just a placeholder so that this is valid Python syntax before # you've completed the assignment. This comment line is # more like what it should look like: # return D, P return None class WeightedDirectedAdjacencyMatrix(WeightedAdjacencyMatrix) : """A weighted digraph represented as a matrix.""" def add_edge(self, u, v, weight) : """Adds a directed edge from u to v with the specified weight. Keyword arguments: u -- source vertex id (0-based index) v -- target vertex id (0-based index) weight -- edge weight """ pass # replace this pass statement with the code needed to implement this def test_floyd_warshall() : """See assignment instructions at top.""" pass # replace this pass statement with the code needed to implement this
Please follow the instructions in the screenshots provided and use that to implement the code given below. Done in python 3.10 or later please.
class WeightedAdjacencyMatrix :
"""A weighted graph represented as a matrix."""
__slots__ = ['_W']
def __init__(self, size, edges=[], weights=[]) :
"""Initializes a weighted adjacency matrix for a graph with size nodes.
Graph is initialized with size nodes and a specified set of
edges and edge weights.
Keyword arguments:
size -- Number of nodes of the graph.
edges -- a list of ordered pairs (2-tuples) indicating the
edges of the graph. The default value is an empty list
which means no edges by default.
weights -- a list of weights for the edges, which should be the same
length as the edges list. The position of a value in
the weights list corresponds to the edge in the same
position of the edges list.
"""
pass # replace this pass statement with the code needed to implement this
def add_edge(self, u, v, weight) :
"""Adds an undirected edge between u to v with the specified weight.
Keyword arguments:
u -- vertex id (0-based index)
v -- vertex id (0-based index)
weight -- edge weight
"""
pass # replace this pass statement with the code needed to implement this
def floyd_warshall(self) :
"""Floyd Warshall
Returns a matrix D consisting of the weights of the shortest
paths between all pairs of vertices, and a matrix P for
the predecessors matrix (what the textbook called PI).
This method MUST NOT change the weight matrix of the graph
itself.
"""
# Your return statement will look something like this one
# in the comment on the following line. That returns
# the two matrices, with the D matrix first. The return None
# is just a placeholder so that this is valid Python syntax before
# you've completed the assignment. This comment line is
# more like what it should look like:
# return D, P
return None
class WeightedDirectedAdjacencyMatrix(WeightedAdjacencyMatrix) :
"""A weighted digraph represented as a matrix."""
def add_edge(self, u, v, weight) :
"""Adds a directed edge from u to v with the specified weight.
Keyword arguments:
u -- source vertex id (0-based index)
v -- target vertex id (0-based index)
weight -- edge weight
"""
pass # replace this pass statement with the code needed to implement this
def test_floyd_warshall() :
"""See assignment instructions at top."""
pass # replace this pass statement with the code needed to implement this
![4) Implement the floyd_warshall method in the WeightedAdjacencyMatrix class.
Since it is in the parent class, you'll be able to use it with either
undirected or directed graphs. Read the docstring for details of what to
implement.
Your method MUST NOT change self. W. So make sure when you initialize
D, that you make a copy of self. W. Do NOT do: D = self. W. That
doesn't copy the list, it just assigns an additional reference to it.
So, changing D would change self. W. Also, do NOT do: D = self._W[:].
That only does a shallow copy. Since W is a 2D list, that will only
copy the first dimension. The first dimension contains references
to 1D list objects, so although D will be a different list than _W,
D[i] will be a reference to the same list object as self._W[i],
so changing D[i][j] will change self._W[i][j]. You need to do a
deep copy. To get this correct, you will either need to write a loop
that does a slice on each row to copy the rows one at a time. Or
try importing Python's copy module, and take a look at the documentation
of the functions in the copy module. One of them will do the deep copy
that you need.
5) Implement the function test_floyd_warshall to test your implementation.
Your test should construct a WeightedAdjacencyMatrix object, call the
floyd_warshall method to compute all pairs shortest paths, and then
output the result with print statements. Make sure you use a case
that you know the correct solution, such as a small graph where you
compute the solution by hand (perhaps the problem from the problem set)
or an example from the textbook might be good since you know the correct
solution to that from the book. You can just call the function from the
shell. You don't need to call it from an if main block. The if main
block is for something else for extra credit. See #6 below.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0d59b49e-3987-421f-888e-fd2b8b6a0cc6%2Ff9f34db2-11ed-4df3-ae1f-a67bf6ba1c20%2Fdq4wypm_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 1 images

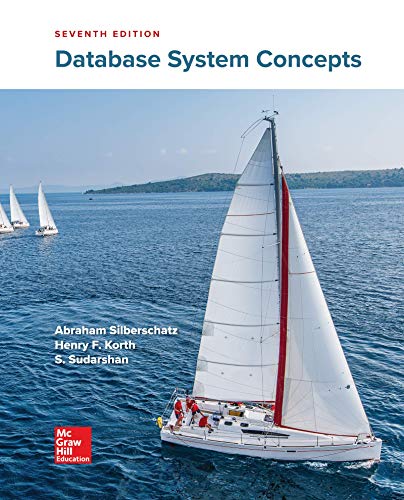
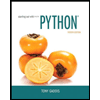
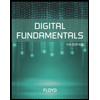
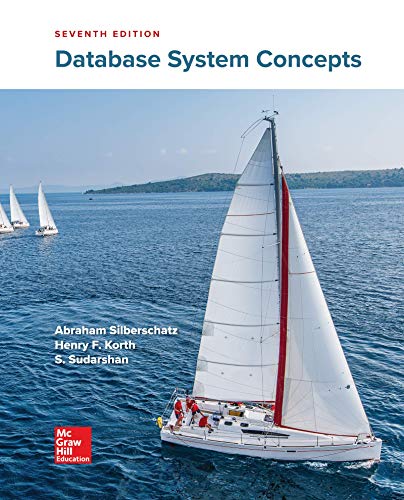
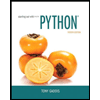
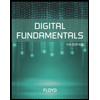
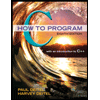
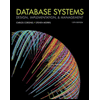
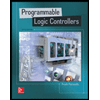