please follow instructions correctly. You are required to complete the LinkedList class. This class is used as a linked list that has many methods to perform operations on the linked list. To create a linked list, create an object of this class and use the addFirst or addLast or add(must be completed) to add nodes to this linked list. Your job is to complete the empty methods. For every method you have to complete, you are provided with a header, Do not modify those headers(method name, return type or parameters). You have to complete the body of the method. package chapter02;
public class LinkedList
{
protected LLNode list;
public LinkedList()
{
list = null;
}
public void addFirst(T info)
{
LLNode node = new LLNode(info);
node.setLink(list);
list = node;
}
public void addLast(T info)
{
LLNode curr = list;
LLNode newNode = new LLNode(info);
if(curr == null)
{
list = newNode;
}
else
{
while(curr.getLink() != null)
{
curr = curr.getLink();
}
curr.setLink(newNode);
}
}
public void add(T afterThis, T info)
{
//TODO Complete this method as required in the homework instructions
}
public void removeFirst()
{
//TODO Complete this method as required in the homework instructions
}
public void removeLast()
{
//TODO Complete this method as required in the homework instructions
}
public int size()
{
//TODO Complete this method as required in the homework instructions
}
public boolean isEmpty()
{
//TODO Complete this method as required in the homework instructions
}
public boolean contains(T item)
{
//TODO Complete this method as required in the homework instructions
}
public void display()
{
LLNode currNode = list;
while(currNode != null)
{
System.out.println(currNode.getInfo());
currNode = currNode.getLink();
}
}
}
please follow instructions correctly. You are required to complete the LinkedList class. This class is used as a linked list that has many methods to perform operations on the linked list. To create a linked list, create an object of this class and use the addFirst or addLast or add(must be completed) to add nodes to this linked list. Your job is to complete the empty methods. For every method you have to complete, you are provided with a header, Do not modify those headers(method name, return type or parameters). You have to complete the body of the method. package chapter02; public class LinkedList { protected LLNode list; public LinkedList() { list = null; } public void addFirst(T info) { LLNode node = new LLNode(info); node.setLink(list); list = node; } public void addLast(T info) { LLNode curr = list; LLNode newNode = new LLNode(info); if(curr == null) { list = newNode; } else { while(curr.getLink() != null) { curr = curr.getLink(); } curr.setLink(newNode); } } public void add(T afterThis, T info) { //TODO Complete this method as required in the homework instructions } public void removeFirst() { //TODO Complete this method as required in the homework instructions } public void removeLast() { //TODO Complete this method as required in the homework instructions } public int size() { //TODO Complete this method as required in the homework instructions } public boolean isEmpty() { //TODO Complete this method as required in the homework instructions } public boolean contains(T item) { //TODO Complete this method as required in the homework instructions } public void display() { LLNode currNode = list; while(currNode != null) { System.out.println(currNode.getInfo()); currNode = currNode.getLink(); } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
please follow instructions correctly.
You are required to complete the LinkedList class. This class is used as a linked list that has many methods to perform operations on the linked list. To create a linked list, create an object of this class and use the addFirst or addLast or add(must be completed) to add nodes to this linked list. Your job is to complete the empty methods. For every method you have to complete, you are provided with a header, Do not modify those headers(method name, return type or parameters). You have to complete the body of the method.
package chapter02;
public class LinkedList
{
protected LLNode list;
public LinkedList()
{
list = null;
}
public void addFirst(T info)
{
LLNode node = new LLNode(info);
node.setLink(list);
list = node;
}
public void addLast(T info)
{
LLNode curr = list;
LLNode newNode = new LLNode(info);
if(curr == null)
{
list = newNode;
}
else
{
while(curr.getLink() != null)
{
curr = curr.getLink();
}
curr.setLink(newNode);
}
}
public void add(T afterThis, T info)
{
//TODO Complete this method as required in the homework instructions
}
public void removeFirst()
{
//TODO Complete this method as required in the homework instructions
}
public void removeLast()
{
//TODO Complete this method as required in the homework instructions
}
public int size()
{
//TODO Complete this method as required in the homework instructions
}
public boolean isEmpty()
{
//TODO Complete this method as required in the homework instructions
}
public boolean contains(T item)
{
//TODO Complete this method as required in the homework instructions
}
public void display()
{
LLNode currNode = list;
while(currNode != null)
{
System.out.println(currNode.getInfo());
currNode = currNode.getLink();
}
}
}

Transcribed Image Text:list.addLast("Smith, John");
list.addLast("Lee, Kelvin");
list.addLast("Davids, Tim");
list.addLast("Willis, Linda");
list.addLast("Wilton, Lisa");

Transcribed Image Text:public void add(T afterThis, T info)
The parameter info will be the information the new node should contain, and the
new node will be added after the node that contains the parameter after This as
an information.
public void removeFirst()
Removes the first node in the linked list. Hint: be aware of empty lists.
public void removeLast()
Removes the last node in the linked list. Hint: be aware of empty lists.
public int size()
returns the size of the linked list.
public boolean isEmpty()
returns true if the linked list is empty, otherwise returns false.
public boolean contains (T item)
returns true if the list contains a node with the same information as the
parameter of the method.
Test your method with TestLinked List, complete one method at the time and
uncomment the method calls in the main method.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
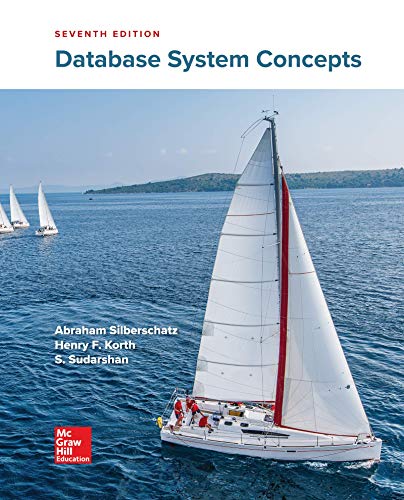
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
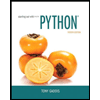
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
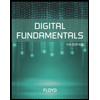
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
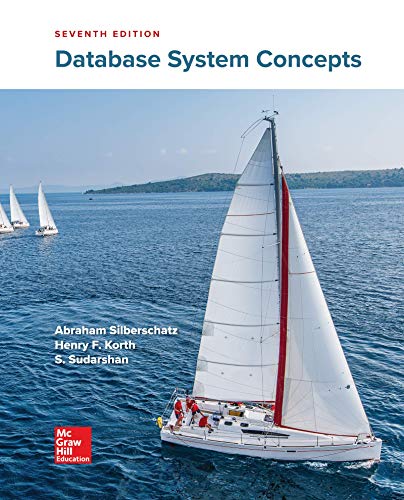
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
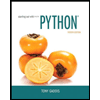
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
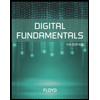
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
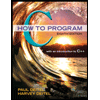
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
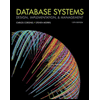
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
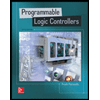
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education