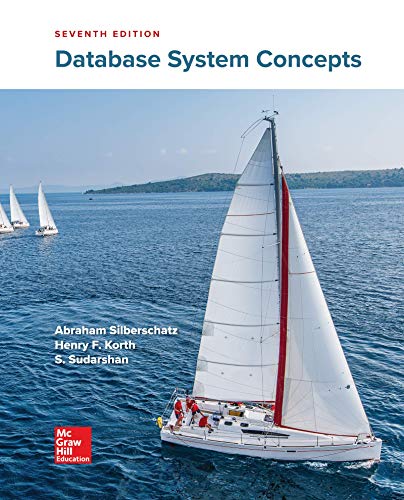
please code in python
Write a function called sooner_date that has 4 integer parameters. The first is a number
between 1 and 12 (inclusive) that represents a month. 1 is January, 2 is February, etc. The
second is a number between 1 and 31 (inclusive) that represents a day. The third parameter is
another integer representing a month and the fourth is another integer parameter representing
a day. So essentially you have 2 dates (the first 2 parameters and the second 2 parameters).
Figure out which date would come sooner, then print out that date in the format month / day.
Here are some examples of calling the function with different arguments. (The code executed is
in blue, the output produced is in green):
sooner_date(1, 1, 1, 2)
1 / 1
sooner_date(2, 5, 1, 3)
1 / 3
sooner_date(8, 25, 7, 30)
7 / 30

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps

- - Provide these 5 (screenshots)sample outputs and tell if each is a palindrome or not.Too bad--I hid a bootSome men interpret eight memos"Go Hang a Salami! I'm a Lasagna Hog"(title of a book on palindromes by Jon Agee, 1991)A man, a plan, a canal—PanamaGateman sees my name, garageman sees name tag LinkedStackADT.javapublic interface LinkedStackADT<T> {boolean isEmptyStack();void push(T val);T peek() throws StackUnderflowException;void pop() throws StackUnderflowException;}=============StackUnderflowException.javapublic class StackUnderflowException extends Exception {public StackUnderflowException(){}public StackUnderflowException(String msg){super(msg);}}=========LinkedStackDS.javapublic class LinkedStackDS<T> implements LinkedStackADT<T>{private class StackNode{T data;StackNode next;StackNode(){}StackNode(T data, StackNode next){this.data = data;this.next = next;}public String toString(){return data.toString();}}private StackNode top;public LinkedStackDS()…arrow_forwardThe owner of a venue for events needs software to keep track of reservations. The venue can handle two events in a day, one scheduled for the afternoon and another scheduled for the evening. Each event consists of the type (wedding, bar mitzvah, etc.), the date of the event, and whether or not the event will be held in the evening. A programmer writes the code given on the handout. Rewrite the above code so that It has all of the correct access modifiers for good encapsulation. Your code obeys the principle of encapsulation that obliges you to put each method in the proper class. Your code uses NO getters and NO additional classes or methods. You are welcome to modify any existing methods (including signatures and bodies) and/or move them between the existing classes. Your code DOES NOT modify any of the constructors. Important notes: You DO NOT have to write a main method. You DO NOT have to write tests. You DO NOT have to write Javadocs. You DO NOT have to write interfaces to…arrow_forwardWrite a function named “endingWithSlashSlash” that accepts the same parameters as command line arguments (argc and argv). It returns true if at least one of the arguments have a “//” at the end and false otherwise. This function also returns the number of arguments that have “//” at the end. For example, “//” will return true and 1 “one// two three// four” will return true and 2 “one” will return false and 0 “one two” will return false and 0 Please note that for question #5, you cannot refer or use string class, string methods such length() or size() or any string function such as strlen(), strcmp or strstr(). Please use only array notation, pointer to character, and/or pointer arithmetic and make use of the fact that this is a C-string.arrow_forward
- I need help solving this in PYTHONarrow_forwardHere is the main function in a program int main () { int x = 30; //more code goes here someFunction (x) ; cout « x; return 0; } Assume the heading for someFunction is written correctly with an integer parameter named x, and that this is the body of that function: { x = x * 20; //more code goes here } What will be the output? Just enter a number. If there would be an error, enter -999arrow_forwardI can't seem to figure this out.. for python You have a business that cleans floors in commercial space and offices. You charge customers $0.45 per square yard, and some customers require multiple cleanings every month. You need two custom functions for your business. The first function takes a floor's length and width in feet and returns the area in square yards. The second void function takes the area, charge per square yard, and number of monthly cleanings as arguments and prints the cleaning cost. This latter function uses selection logic to print different output for single and multiple monthly cleanings (see Sample Outputs). Use a properly named constant for the charge per square yard, too.arrow_forward
- Write a program that has three functions. The first ask the user to enter a temperature in degrees celcius. The second takes a single parameter that is a temperature in degrees Celsius and returns the corresponding temperature in Fahrenheit. The thirsd function takes one parameter, a temperture in celsius and print the temperature in Celsius along with the corresponding temperature in Fahrenhiet. This function will use the second function. Then write a complete program that asks the user for a temperatre in Celsius and prints the corresponding temperature in Farenheit.arrow_forwardGiven the function of def happyBirthday(name, age = 10): print("Happy Birthday", name, "I hear your", age, "today") What is the output with a function call of: happyBirthday(age = 7, "Sarah") Happy Birthday Sarah I hear your 10 today Happy Birthday Sarah I hear your 7 today Happy Birthday 7 I hear your Sarah today Error Message Parameters are __________ Variables defined in a function header. Variables or constants used in a function call. Both variable defined in a function header, and a local variable for the function Local variables to a function. from graphics import * def drawDoor(x1, y1, x2, y2): '''draws a door with opposite corners at x1, y1 and x2, y2''' door = Rectangle(Point(x1, y1), Point(x2, y2)) door.setFill('red') door.draw(win) knob = Circle(Point(x2 - 10, (y1 + y2)//2), 3) knob.setFill('yellow') knob.draw(win) True or False: the function call is…arrow_forwardA group of statisticians at a local college has asked you to create a set of functions using python programming that compute the median and mode of a set of numbers, as defined in Section 5.4. Define these functions in a module named stats.py. Also include a function named mean, which computes the average of a set of numbers. Each function should expect a list of numbers as an argument and return a single number. Each function should return 0 if the list is empty. Include a main function that tests the three statistical functions with a given list.arrow_forward
- Write a program called gcd.java that repeatedly asks the user to input two integer numbers and print out those numbers' greatest common divisor. Computing and printing the GCD should be done in a function named calculate_gcd that takes the two numbers received from the user as input parameters. Your program should ask the user if they want to end; otherwise, continue asking for the two numbers and printing the results. Here is a part of the program below. Complete the code where the comment states to. The output is also included.arrow_forwardUSE PYTHON TANK YOUarrow_forwardCreate a program in python with a main function and a custom module named rect that defines three custom functions about rectangles as follows: a value-returning function that takes a rectangle's dimensions as parameters and returns the perimeter. a void function that takes a rectangle's dimensions as parameters and prints the area. a value-returning function that takes a rectangle's dimensions as parameters and returns the diagonal length. In the main function, import your custom module, prompt the user for the dimensions of a rectangle, and then use all three module functions. Duplicate the display of decimals shown in the Sample Output.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
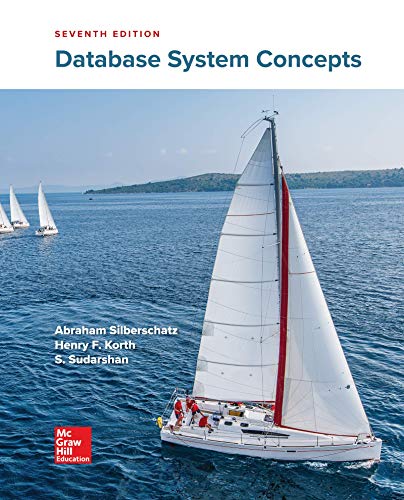
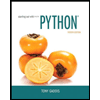
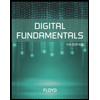
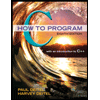
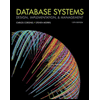
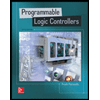