Part1) Implement the interval search method in C. Read a positive integer a from the user of which square root we would like to find. Take the initial interval as (0, a) and divide this interval into 10 equal size sections at each iteration. Repeat this search until the root is found or the difference between x1 and x2 becomes less than 0.0001. Print the resulting value of the root (if one is found) or the value of x1 if the root cannot be found exactly. Hint: You need to check each section to see if the root resides within that section by evaluating f(x) at the section boundaries and looking at the signs of the evaluations. If the section contains the root, then divide the section into 10 new sections and repeat the process
Part1) Implement the interval search method in C. Read a positive integer a from the user of which square root we would like to find. Take the initial interval as (0, a) and divide this interval into 10 equal size sections at each iteration. Repeat this search until the root is found or the difference between x1 and x2 becomes less than 0.0001. Print the resulting value of the root (if one is found) or the value of x1 if the root cannot be found exactly. Hint: You need to check each section to see if the root resides within that section by evaluating f(x) at the section boundaries and looking at the signs of the evaluations. If the section contains the root, then divide the section into 10 new sections and repeat the process
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Computer science

Transcribed Image Text:If the square root of an integer number a is x, then the following equation holds: x 2 = a By moving
the constant a to the left side of the equality, we get: f(x) = x 2 - a= 0 Therefore, the roots of the
above quadratic function will be the square roots of a.
Part1) Implement the interval search method in C. Read a positive integer a from the user of which
square root we would like to find. Take the initial interval as (0, a) and divide this interval into 10
equal size sections at each iteration. Repeat this search until the root is found or the difference
between x1 and x2 becomes less than 0.0001. Print the resulting value of the root (if one is found) or
the value of x1 if the root cannot be found exactly. Hint: You need to check each section to see if the
root resides within that section by evaluating f(x) at the section boundaries and looking at the signs
of the evaluations. If the section contains the root, then divide the section into 10 new sections and
repeat the process.
Part2) Implement the Newton-Raphson formula in C. Read a positive integer a from the user of
which square root we would like to find. Start with the initial estimate of root as a (i.e., xi = a) and
apply the formula in (1) to find the next estimate of the root at each iteration. Repeat the iterations
until the difference between the current estimate and the previous estimate of the root becomes
less than 0.0001. Print the resulting value of the root (if one is found) or the value of x1 if the root
cannot be found exactly.

Transcribed Image Text:If the square root of an integer number a is x, then the following equation holds:
x² = a
By moving the constant a to the left side of the equality, we get:
f(x)=x²-a = 0
Therefore, the roots of the above quadratic function will be the square roots of a.
This function's graph is a simple parabola as show below.
X1
-a
X₂
f(x)
root of f(x)
If f(x₁) and f(x₂) have opposite signs, then there is at least one root in the interval (x1, x2) as shown above.
If the interval is small enough, it is likely to contain a single root. Thus, the roots of f(x) can be detected
by repetitively evaluating the function at shrinking intervals (x1, x2) and looking for a change in sign
between f(x1) and f(x2).
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
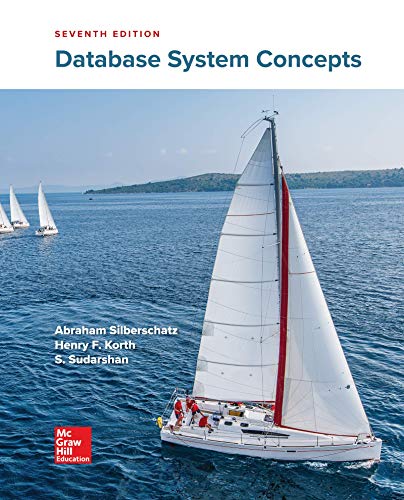
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
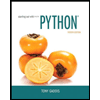
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
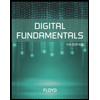
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
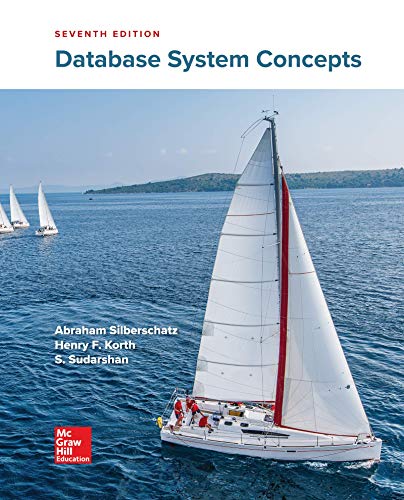
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
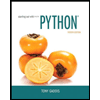
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
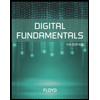
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
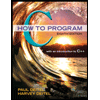
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
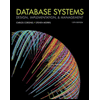
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
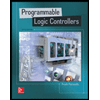
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education