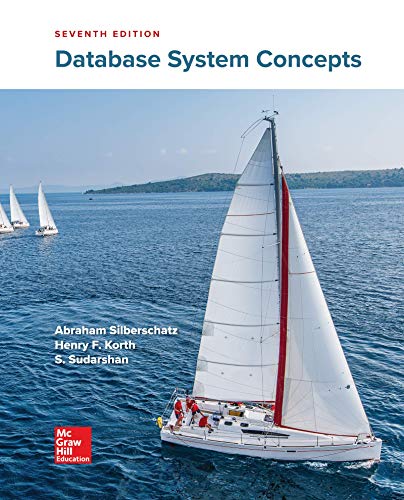
Part A
Create a FitnessTracker class that includes three data fields for a fitness activity: a String for the name of the activity as activity, an int for the number of minutes spent participating as minutes, and a LocalDate for the date as date.
Create a default constructor that automatically sets the activity to running, the minutes to 0, and the date to January 1 of the current year. Overload the constructor so it receives parameters for each of the fields and assigns them appropriately.
Includes methods to get each data field.
Part B
Create an application that instantiates two FitnessTracker objects. Prompt the user for values for one object, and pass those values to the three constructor parameters. Retain the default constructor values for the other object. Display the values for each object.
An example of the program is shown below:
Enter activity >> swimming
Enter minutes spent swimming >> 90
Enter month >> 2
Enter day >> 24
Enter year >> 2021
Activity log:
swimming 90 minutes on 2021-02-24
running 0 minutes on 2021-01-01
![O
O
STUDENT OBERGES LITERACTE SHUMPLEJ
Janda
Temban
PROJECTS
J
11
13
16
16
Ő https://literate-sniffle-x5wx9wg5x7w4fr5r.github.dev/?folder=/workspaces/9780357673423_java-programming...
28
MindTap X
import java.io.
public class testicacar
blycling
public static void main(string[] args)
Fitrackercise-ow Fitnesstracker
Compani X Q fitness tra X
Syst..tuwcis.getActivity()+"arcisa.gentinutas() +
Localitate date-Lacale.(, ,
Fiterackied-new Fitnesstrackwe("bicycling", 86, cate);
Systrici.gctivity)+ci.gontinutos() +
social et
am SO THUN FORT ⒸOM
ng-a-kardas-ok-t20-m-poundhapter VILLE DİVARIO
cp2406_f X Q testsand X
...totstan joa-programming-a-chuc-20-st sanchavarijichapterqikan pintudat (sepāre) $ ja -p "berspace
OʻGALE-HİKunciavačijichag tarquart (späte) $ja
... ja programing-me-sachuc--tam-se-raciydana (plnej š
pop-m-catzy_jna-programming-an-sandhuc-ok-tan-med-mouvenýchapter T
AN
Solved: C X C chegg.co X
☐
At
w
How to Use the Code Editor
1. Select the "Run Code' button to cute the program.
2. Select the "Calculate Grade" button to generate a score based on the completed tasks.
1. Continue to modify, run, and calculate your code unt you are happy with the grade.
4. Select the "Submi" button to turn in the assignment to your instructo
Part A
seter activity
ing
ser ut spot winning w
inter 2
etter day 2524
ter year 201
Activity log:
ne minutes 2825-02-20
ingen 221-1-1
Task Focate the treacher das
Create a Fitnesstracker class that includes three data fields for a fitness activity: a string for the name of the activity at activity, an ist for the number of minutes spent participating as minutes, and a localute for
the date.
Tsk Add the the data fields to the Fitnesstracker class.
Q
Create a default constructor that automatically sets the activity to running, the sites to 0, and the date to January 1 of the current year. Overicad the constructor so it receives parameters for each of the fields and
aigns the appropriately.
Includes methods to get each datafield.
Part B
Task Focate the detectacconc
cp2406_f X
Al
Create an application that instantiates two Fitresstracker objects. Prompt the user for values for one object, and pass those values to the three constructor parameters. Retain the default constructor values for the
other object Display the values for each object.
An example of the program is shown below
Task include get methods for each datafield.
Task Forlad the Fitnesstracker contractar to accept parameters for each data faid
Your current grade: 0.00%
Success X
RUN CODE CALCULATE GRADE
{}
וח
+
000
SUBMIT
Bo
*](https://content.bartleby.com/qna-images/question/63639615-d990-48b7-9249-2331d859943f/7d9e2f0b-22ce-4555-8c0f-d61cdde3dfb0/0r468fb_thumbnail.png)

Step by stepSolved in 4 steps with 3 images

- A field has access that is somewhere between public and private. static final Opackage Oprotectedarrow_forward1. Write a class named Coin. The Coin class should have the following field: • A String named sideUp. The sideUp field will hold either "heads" or "tails" indicating the side of the coin that is facing up. The Coin class should have the following methods: • A no-arg constructor that randomly determines the side of the coin that is facing up ("heads" or "tails") and initializes the sideUp field accordingly. • A void method named toss that simulates the tossing of the coin. When the toss method is called, it randomly determines the side of the coin that is facing up ("heads" or "tails") and sets the sideUp field accordingly. • A method named getSide Up that returns the value of the side Up field. Write a program that demonstrates the Coin class. The program should create an instance of the class and display the side that is initially facing up. Then, use a loop to toss the coin 20 times. Each time the coin is tossed, display the side that is facing up. The program should keep count of…arrow_forwardclass Duration: def __init__(self, hours, minutes): self.hours = hours self.minutes = minutes def __add__(self, other): total_hours = self.hours + other.hours total_minutes = self.minutes + other.minutes if total_minutes >= 60: total_hours += 1 total_minutes -= 60 return Duration(total_hours, total_minutes) first_trip = Duration(3, 36)second_trip = Duration(0, 47) first_time = first_trip + second_tripsecond_time = second_trip + second_trip print(first_time.hours, first_time.minutes) what is the outputarrow_forward
- 1- Design a class named Rectangle to represent a Rectangle. The class contains: • three variables integer data field named height, integer data filed named width and integer data filed named length. The default values are 1 for height, length, and width are 1.0 for each of them respectively. • A no-arg constructor that creates a default Rectangle. • A constructor that creates a Rectangle with the specified height, length and width. • A method named getVolume() that returns the volume of this Rectangle. (Volume = height * width * length) %3! 2- Write a test program called Test Rectangle that: • Creates two Rectangle objects: one object with height 11, length 5, and width 7, the other object with height 10, length 2 and width 6 • Display the volume of each Rectangle object.arrow_forward1. Create a class as described below: Class Name: Room Fields: standard room fee amenities total cost Constructors:A default constructor to initialize the fields. The standard room fee field should be set to $200 and the amenities field to zero. Another constructor with one argument. It should assign this value to the standard room fee and the amenities field to the default value of $75. Methods: getStandardFee() - This methods returns the standard room fee field value. getAmenities() - This method returns the amenities field value. getTotalCost() - This method returns the total cost field value. calcTotalCost() - This method calculated the total cost for an instance of the class setStandard Fee() - This method has one argument representing the standard room fee. setAmenities() - This method has one argument representing the amenities for the room.arrow_forwardCode should be in Pythonarrow_forward
- For your homework assignment, build a simple application for use by a local retail store. Your program should have the following classes: Item: Represents an item a customer might buy at the store. It should have two attributes: a String for the item description and a float for the price. This class should also override the __str__ method in a nicely formatted way. Customer: Represents a customer at the store. It should have three attributes: a String for the customer's name, a Boolean for the customer's preferred status, and a list of 5 indexes to hold Item objects. Include the following methods: make_purchase: accepts a String and a double as parameters to represent the name and price of an item this customer is purchasing. Create a new Item object with this info and append it to the internal list. If the customer is a preferred customer based on the Boolean attribute's value, take 10% off the total sale price. __str__: Override this method to print the customer's name and every…arrow_forwardin C#, Visual Basic Create a Car class that has at least the following properties:• Year• Make• Price• Passengers-holds the number of seat belts available in the car (presumably how many passengers the car can hold). This field must be private since users presumably can’t change this value. Have two constructors: one that initializes everything to some default value for the base price of a car and one that also accepts values for luxury upgrades of: heated seats, sun-roof, and satellite radio. The class has two methods: 1) Get Paint Color() which accepts a standard base color option but allows the user to purchase a pricier customized color 2) Upgrade Costs() which computes the cost of user-selected luxury items and adds it to the price. Create three car objects: Ford (make Explorer), Lexus (make ES) and a Clown Car which overrides the passenger value to allow 20 clowns in the car. The Get Paint Color() and Upgrade Costs() functions needs to be called for each object and data for all…arrow_forwardCreate a class of Dog, some of the differences you might have listed out maybe breed, age, size, color, etc. Then create characteristics (breed, age, size, color) can form a data member for your object. Then list out the common behaviors of these dogs like sleep, sit, eat, etc. Design the class dogs with objects and methods.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
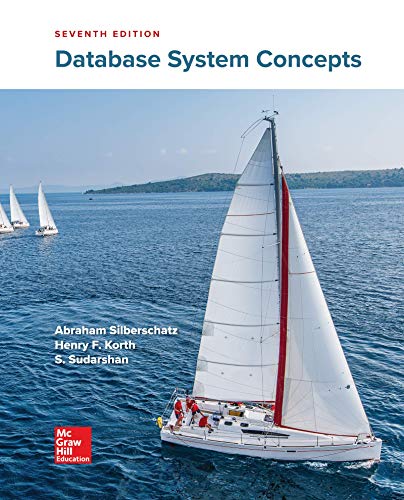
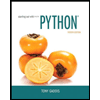
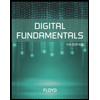
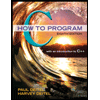
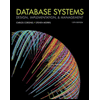
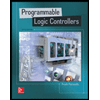