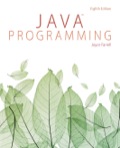
Concept explainers
Overview
In this assignment, the student will write a C++ program that identifies a non-negative integer as prime, composite, or neither and provides the unique prime factorization for the composite case.
When completing this assignment, the student should demonstrate mastery of the following concepts:
· Decision Making (if, else)
· Iteration (for, while)
· Number Theory (Prime Factors)
· Advanced Loop Variants
Assignment
Write a C++ program that prompts the user for a single integer value. After receiving the value, perform an analysis to determine if the integer is prime, composite or neither. If you are not sure what this means, you need to research the appropriate topics in number theory and consider what the
In the event that you determine the provided integer is composite, provide a listing of the unique prime factorization for that integer as illustrated in the below examples.
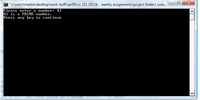

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- 18. Tom and Jerry opened a new lawn service. They provide three types of services: mowing, fertilizing, and planting trees. The cost of mowing is $35.00 per 5,000 square yards, fertilizing is $30.00 per application, and planting a tree is $50.00. Write an algorithm that prompts the user to enter the area of the lawn, the number of fertilizing applications, and the number of trees to be planted. The algorithm then determines the billing amount. (Assume that the user orders all three services.) (9)arrow_forward(Simulation) Write a program to simulate the roll of two dice. If the total of the two dice is 7 or 11, you win; otherwise, you lose. Embellish this program as much as you like, with betting, different odds, different combinations for win or lose, stopping play when you have no money left or reach the house limit, displaying the dice, and so forth. (Hint: Calculate the dots showing on each die with the expression dots=(int)(6.0randomnumber+1), where the random number is between 0 and 1.)arrow_forwardAccount numbers sometimes contain a check digit that is the result of a mathematical calculation. The inclusion of the digit in an account number helps ascertain whether the number is a valid one. Write an application named CheckDigit that asks a user to enter a four-digit account number and determines whether it is a valid number. The number is valid if the fourth digit is the remainder when the number represented by the first three digits of the four-digit number is divided by 7. For example, 7770 is valid, because 0 is the remainder when 777 is divided by 7. The next problems rely on the generation of a random number. You can create a random number that is at least mi n but less than max using the following statements: Random ranNumberCenerator = new Random(); int randomNumber; randomNumber = ranNumberGenerator .Next(min, max);arrow_forward
- (Misc. application) a. Write a program that continuously requests a grade to be entered. If the grade is less than 0 or greater than 100, your program should print an appropriate message informing the user that an invalid grade has been entered; else, the grade should be added to a total. When a grade of 999 is entered, the program should exit the repetition loop and compute and display the average of the valid grades entered. b. Run the program written in Exercise 2a and verify the program by using appropriate test data.arrow_forward(Numerical) Write an assignment statement to calculate the nth term in an arithmetic sequence. This is the formula for calculating the value, v, of the nth term: v=a+(n1)d a is the first number in the sequence. d is the difference between any two numbers in the sequence.arrow_forwardFor each of the following exercises, you may choose to write a console-based or GUI application, or both. Write a program named TestScoreList that accepts eight int values representing student test scores. Display each of the values along with a message that indicates how far it is from the average.arrow_forward
- Malcolm Movers charges a base rate of $200 per move plus $150 per hour and $2 per mile. Write a program named MoveEstimator that prompts a user for and accepts estimates for the number of hours for a job and the number of miles involved in the move and displays the total moving fee.arrow_forward(Computation) A magic square is a square of numbers with N rows and N columns, in which each integer value from 1 to (N * N) appears exactly once, and the sum of each column, each row, and each diagonal is the same value. For example, Figure 7.21 shows a magic square in which N=3, and the sum of the rows, columns, and diagonals is 15. Write a program that constructs and displays a magic square for a given odd number N. This is the algorithm:arrow_forward26. A room has one door, two windows, and a built-in bookshelf and it needs to be painted. Suppose that one gallon of paint can paint 120 square feet. Write a program that prompts the user to input the lengths and widths of the door, each window, the bookshelf; and the length, width, and height of the room (in feet). The program outputs the amount of paint needed to paint the walls of the room.arrow_forward
- a. Write a program named CheckMonth that prompts a user to enter a birth month. If the value entered is greater than 12 or less than 1, display an error message; otherwise, display the valid month with a message such as 3 is a valid month. b. Write a program named CheckM0nth2 that prompts a user to enter a birth month and day. Display an error message if the month is invalid (not I through 12) or the day is invalid for the month (for example, not between 1 and 31 for January or between I and 29 for February). If the month and day are valid, display them with a message.arrow_forward4. During each summer, John and Jessica grow vegetables in their backyard and buy seeds and fertilizer from a local nursery. The nursery carries different types of vegetable fertilizers in various bag sizes. When buying a particular fertilizer, they want to know the price of the fertilizer per pound and the cost of fertilizing per square foot. The following program prompts the user to enter the size of the fertilizer bag, in pounds, the cost of the bag, and the area, in square feet, that can be covered by the bag. The program should output the desired result. However, the program contains logic errors. Find and correct the logic errors so that the program works properly. // Logic errors. #include #include using namespace std; int main() { double costs double area; double bagsize; cout > bagsize; cout > cost; cout > area; cout << endl; cout << "The cost of the fertilizer per pound is: $" << bagsize / cost << endl; cout << "The cost of fertilizing per square foot is: $" << area / cost << endl; return 0; }arrow_forwardA structure that allows repeated execution of a block of statements is a(n) _____________. sequence selection array looparrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
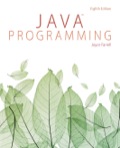
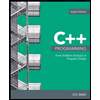
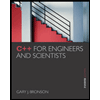
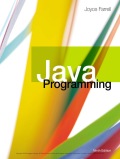
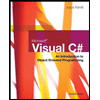