Output each floating-point value with two digits after the decimal point, which can be achieved by executing cout << fixed << setprecision(2); once before all other cout statements. 1) Prompt the user to enter five numbers, being five people's weights. Store the numbers in a vector of doubles. Output the vector's numbers on one line, each number followed by one space. 2) Also output the total weight, by summing the vector's elements. 3) Also output the average of the vector's elements. 4) Also output the max vector element.
I'm doing something wrong but I'm not sure what. This is in C++.
Question
Output each floating-point value with two digits after the decimal point, which can be achieved by executing
cout << fixed << setprecision(2); once before all other cout statements.
1) Prompt the user to enter five numbers, being five people's weights. Store the numbers in a
2) Also output the total weight, by summing the vector's elements.
3) Also output the average of the vector's elements.
4) Also output the max vector element.
I've attached images of what I did and the output full of errors.
Default template:
#include <iostream>
#include <iomanip> // For setprecision
// FIXME include vector library
using namespace std;
int main() {
/* Type your code here. */
return 0;
}
Thank you



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

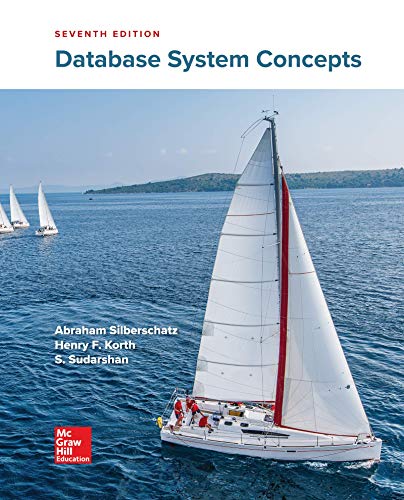
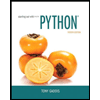
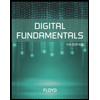
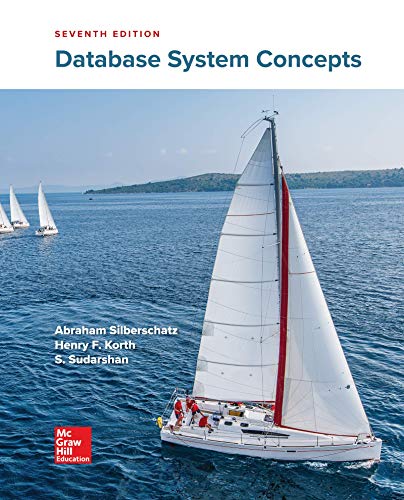
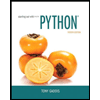
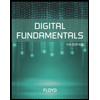
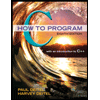
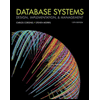
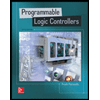