ou are said to store data of Hospitals in a city. For that purpose, you are asked to develop a structure of maxHeap using these structures in c++. You have to submit Hospital. h file only
You are said to store data of Hospitals in a city. For that purpose, you are asked to develop a structure of maxHeap using these structures in c++. You have to submit Hospital. h file only
class Hospital
{
string HospitalName;
string Address;
string HospitalID;
public:
Hospital();
Hospital(string ,string ,string);
Hospital(string HospitalID); // conversion Constructor
string getHospitalName()const;
string getHospitalId()const;
string getAddress()const;
void setHospitalName(string);
void setHospitalId(string);
void setAddress(string);
// Relational operators with respect to Hospital Id
bool operator<(Hospital)const;
bool operator<=(Hospital)const;
bool operator>(Hospital)const;
bool operator>=(Hospital)const;
bool operator==(Hospital)const;
bool operator!=(Hospital)const;
// output stream operator
friend ostream& operator<<(ostream&, const Hospital& ref); s
};
ostream& operator<<(ostream&, const Hospital& ref);
class MaxHeap
{
public:
class Node
{
public:
Hospital data;
Node* left;
Node* right;
};
private:
Node* root;
public:
MaxHeap();
bool Insert(Hospital);
bool Remove(Hospital);
bool Replace(Hospital old_Hos, Hospital new_Hos);
Hospital* getHeapArray()const;
~MaxHeap();
};
#include <iostream>
#include"Hostpital.h"
using namespace std;
int main()
{
MaxHeap a;
a.Insert(Hospital("Meo", "Lahore", "HL1"));
a.Insert(Hospital("Jinnah", "Lahore", "HL2"));
a.Insert(Hospital("Civil", "Lahore", "HL3")); a.Insert(Hospital("Cardialogy", "Lahore", "HL4")); a.Insert(Hospital("Nishtar", "Multan", "HM1"));
a.Insert(Hospital("Punjab Medical", "Faisalabad", "HF1")); a.Insert(Hospital("Allied", "Faisalabad", "HF2"));
Hospital* ptr= a.getHeapArray();
for (int i = 0; i < 7; i++)
{
cout << i[ptr]<<endl;
}
delete[] ptr;
a.Remove(Hospital("HF2"));
a.Replace(Hospital("HL4"), Hospital("THQ", "Burewala", "HB1"));
ptr = a.getHeapArray();
for (int i = 0; i < 6; i++)
{
cout << i[ptr] << endl;
}
delete[] ptr;
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

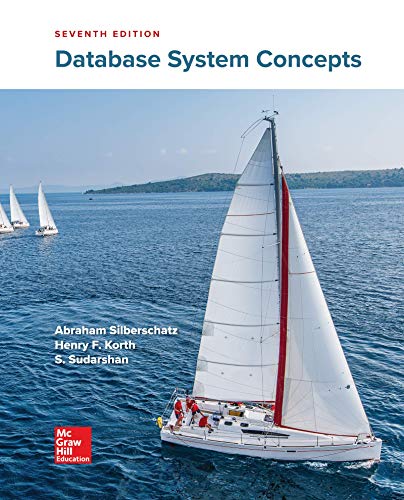
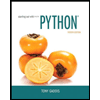
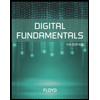
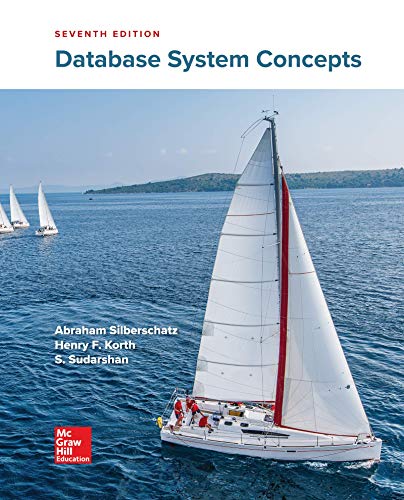
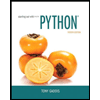
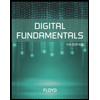
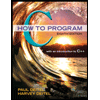
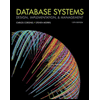
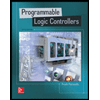