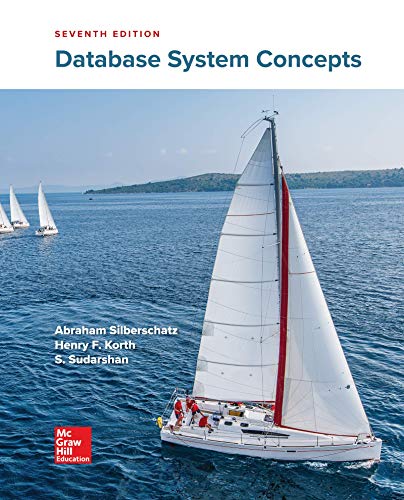
Instructions from my Introduction to
#1) Write code in C++ to read a file and produce on the console a "Monthly Sales Report."
#2) Open a file called Sales_2020_08.txt. Using the following:
#include <iostream>
#include <fstream>
int main()
{
ifstream myInFileStream ();
myInFileStream.open ("Sales_2020_08.txt");
#3) Read each record that has variables such as FirstName, LastName, SalesAmount, and etc.
#4) Produce a nice looking professional report (an example of the professional report is in the picture attached) that includes:
A) Headings
B) One line for each salesperson; each line includes a line number, salespersons names, gross sales, and calculated sales commission
C) total line with a total of the gross sales and the commissions.
#5) CODE REQUIREMENTS:
- Code to open the file
- a loop that stops on EOF or other
mechanisms. - a line counter for each line.
- Commission rates are based on gross sales amount:
- 5.5% for sales in the range of 0 - 10000.00
- 6.7% for sales in the range of 10000.01 - 22500.00
- 11.2% for sales greater than 22500.00
#6) DO NOT DO THE FOLLOWING:
A) Do not manually count the number of records and put the reading of the records in a for loop or while loop with the number of records controlling the loop.
B) There are no user inputs so do not manually code any of the data. Once the program executes, we are simply just reading the date from the file.
#7) The attached photo is an example of how your file must output when executed
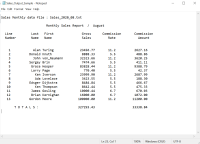

Step by stepSolved in 3 steps with 2 images

- How do you write a code in c++ that gives the user an option to save(write) a program(example the program arranges numbers in ascending order) onto a text file.arrow_forwardFileAttributes.c 1. Create a new C source code file named FileAttributes.c preprocessor 2. Include the following C libraries a. stdio.h b. stdlib.h c. time.h d. string.h e. dirent.h f. sys/stat.h 3. Function prototype for function printAttributes() main() 4. Write the main function to do the following a. Return type int b. Empty parameter list c. Declare a variable of data type struct stat to store the attribute structure (i.e. statBuff) d. Declare a variable of data type int to store an error code (i.e. err) e. Declare a variable of data type struct dirent as a pointer (i.e. de) f. Declare a variable of data type DIR as a pointer set equal to function call opendir() passing explicit text “.” as an argument to indicate the current directory (i.e. dr) g. If the DIR variable is equal to NULL do the following i. Output to the…arrow_forwardI am confused as to how reading files work in C++, and would like to know what is a way to read and to also display files in C++?arrow_forward
- write a pseudo code for the C++ program below: The C++ code is given below : //C++ Code #include<iostream>#include<fstream>using namespace std;/*Create a global variable of type ofstream for the output file*/ofstream outfile; /** This function asks the user for the number of employees in the company. This value should be returned as an int. The function accepts no arguments (No parameter/input).*/int NumOfEmployees();/** accepts an argument of type int for the number of employees in the company and returns the total of missed days as an int. This function should do the following:Asks the user to enter the following information for each employee:The employee number (ID) (Assume the employee number is 4 digits or fewer, but don't validate it).The number of days that employee missed during the past year.Writes each employee number (ID) and the number of days missed to the output file */int TotDaysAbsent(int numberOfEmployees);/* calculates the average number of days…arrow_forwardpseudo code for the code //C++ Code #include<iostream>#include<fstream>using namespace std;/*Create a global variable of type ofstream for the output file*/ofstream outfile; /** This function asks the user for the number of employees in the company. This value should be returned as an int. The function accepts no arguments (No parameter/input).*/int NumOfEmployees();/** accepts an argument of type int for the number of employees in the company and returns the total of missed days as an int. This function should do the following:Asks the user to enter the following information for each employee:The employee number (ID) (Assume the employee number is 4 digits or fewer, but don't validate it).The number of days that employee missed during the past year.Writes each employee number (ID) and the number of days missed to the output file */int TotDaysAbsent(int numberOfEmployees);/* calculates the average number of days absent.The function takes two arguments:the number of…arrow_forwardThe Payroll Department keeps a list of employee information for each pay period in a text file. The format of each line of the file is the following: <last name> <hours worked> <hourly wage> Write a program that inputs a filename from the user and prints to the terminal a report of the wages paid to the employees for the given period. The report should be in tabular format with the appropriate header. Each line should contain: An employee’s name The hours worked The wages paid for that period. An example of the program input and output is shown below: Enter the file name: data.txt Name Hours Total Pay Lambert 34 357.00 Osborne 22 137.50 Giacometti 5 503.50arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
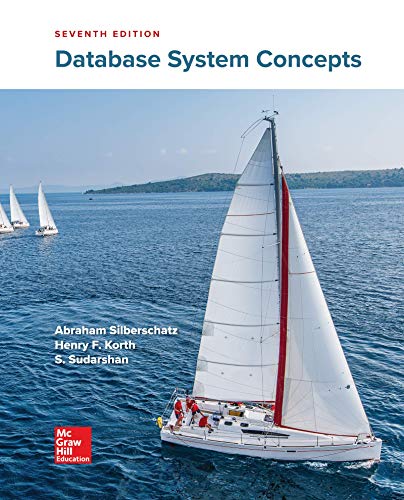
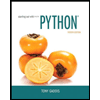
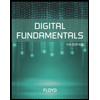
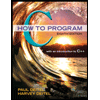
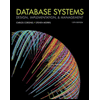
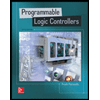