OCaml Code: Below is streams.ml and it needs the value "head". Please fix the code and make sure to test the code using various test cases to make sure the code works. streams.ml let rec even n = if n = 0 then true else odd (n - 1) and odd n = if n = 0 then false else even (n - 1) let rec squares n = let rec squares_aux m acc = if m = 0 then List.rev acc else squares_aux (m - 1) (acc @ [m * m]) in squares_aux n [] let rec fibs n = let rec fibs_aux a b count acc = if count = 0 then List.rev acc else fibs_aux b (a + b) (count - 1) (a :: acc) in
OCaml Code: Below is streams.ml and it needs the value "head". Please fix the code and make sure to test the code using various test cases to make sure the code works.
streams.ml
let rec even n =
if n = 0 then true
else odd (n - 1)
and odd n =
if n = 0 then false
else even (n - 1)
let rec squares n =
let rec squares_aux m acc =
if m = 0 then List.rev acc
else squares_aux (m - 1) (acc @ [m * m])
in
squares_aux n []
let rec fibs n =
let rec fibs_aux a b count acc =
if count = 0 then List.rev acc
else fibs_aux b (a + b) (count - 1) (a :: acc)
in
fibs_aux 0 1 n []
let rec evenFibs n =
let rec fibs_aux a b count acc =
if count = 0 then List.rev acc
else if a mod 2 = 0 then fibs_aux b (a + b) (count - 1) (a :: acc)
else fibs_aux b (a + b) count acc
in
fibs_aux 0 1 n []
let rec oddFibs n =
let rec fibs_aux a b count acc =
if count = 0 then List.rev acc
else if a mod 2 = 1 then fibs_aux b (a + b) (count - 1) (a :: acc)
else fibs_aux b (a + b) count acc
in
fibs_aux 1 1 n []
let rec is_prime n =
let rec is_not_divisible k =
k * k > n || (n mod k <> 0 && is_not_divisible (k + 1))
in
n > 1 && is_not_divisible 2
let rec primes n =
let rec find_primes k count acc =
if count = 0 then List.rev acc
else if is_prime k then find_primes (k + 1) (count - 1) (k :: acc)
else find_primes (k + 1) count acc
in
find_primes 2 n []
let rec rev_zip_diff l1 l2 f =
match l1, l2 with
| [], _ | _, [] -> []
| x :: xs, y :: ys -> (x, y, f (x, y)) :: rev_zip_diff xs ys f
let rec printGenList lst f =
match lst with
| [] -> ()
| x :: xs -> f x; printGenList xs f
let rec printList lst filename =
let oc = open_out filename in
let rec print_list_helper lst =
match lst with
| [] -> close_out oc
| x :: xs -> output_string oc (string_of_int x ^ " "); print_list_helper xs
in
print_list_helper lst
let rec printPairList lst filename =
let oc = open_out filename in
let rec print_pair_list_helper lst =
match lst with
| [] -> close_out oc
| (x, y) :: xs ->
output_string oc ("(" ^ string_of_int x ^ ", " ^ string_of_int y ^ ") ");
print_pair_list_helper xs
in
print_pair_list_helper lst
let rec take n lst =
if n <= 0 then []
else
match lst with
| [] -> []
| x :: xs -> x :: take (n - 1) xs
let string_of_tuple_list lst =
"[" ^ String.concat "; " (List.map (fun (x, y, z) -> "(" ^ string_of_int x ^ ", " ^ string_of_int y ^ ", " ^
string_of_int z ^ ")") lst) ^ "]"
let () =
let string_of_int_list lst = "[" ^ String.concat "; " (List.map string_of_int lst) ^ "]" in
print_endline ("even 2;;\n- : bool = " ^ string_of_bool (even 2));
print_endline ("even 3;;\n- : bool = " ^ string_of_bool (even 3));
print_endline ("odd 2;;\n- : bool = " ^ string_of_bool (odd 2));
print_endline ("odd 3;;\n- : bool = " ^ string_of_bool (odd 3));
print_endline ("take 10 squares;;\n- : int list = " ^ string_of_int_list (take 10 (squares 10)));
print_endline ("take 10 fibs;;\n- : int list = " ^ string_of_int_list (take 10 (fibs 10)));
print_endline ("take 10 evenFibs;;\n- : int list = " ^ string_of_int_list (take 10 (evenFibs 10)));
print_endline ("take 10 oddFibs;;\n- : int list = " ^ string_of_int_list (take 10 (oddFibs 10)));
print_endline ("take 10 primes;;\n- : int list = " ^ string_of_int_list (take 10 (primes 10)));
print_endline ("take 5 (rev_zip_diff evenFibs oddFibs (fun (x,y)->x-y));;\n- : (int * int * int) list = " ^
string_of_tuple_list (take 5 (rev_zip_diff (evenFibs 10) (oddFibs 10) (fun (x, y) -> x - y))));
print_endline "printGenList [\"how\"; \"the\"; \"turntables\"] (fun s -> print_string (s ^ \" \"));;";
printGenList ["how"; "the"; "turntables"] (fun s -> print_string (s ^ " "));
print_endline "\n-: unit = ()";
print_endline "printList [2; 4; 6; 8] \"printList.txt\";; (* \"2 4 6 8 \" *)";
printList [2; 4; 6; 8] "printList.txt";
print_endline "printPairList [(2, 1); (3, 2); (4, 3)] \"printPairList.txt\";; (* \"(2, 1) (3, 2) (4, 3) \" *)";
printPairList [(2, 1); (3, 2); (4, 3)] "printPairList.txt"

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

I ran the code and still got the same error. The code still needs the value head. Please fix the code and show screenshots of the correct code with the output. Make sure to use various test cases to test the code to make sure it works. Attached are images of the directions.
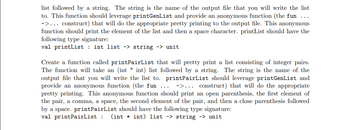
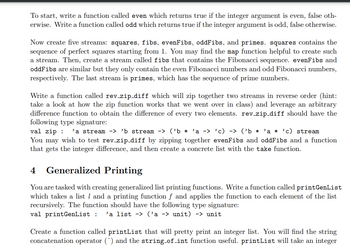
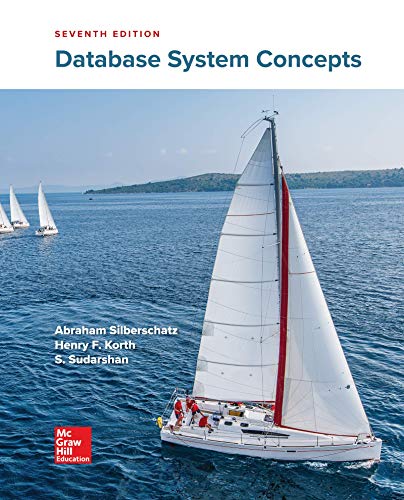
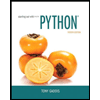
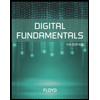
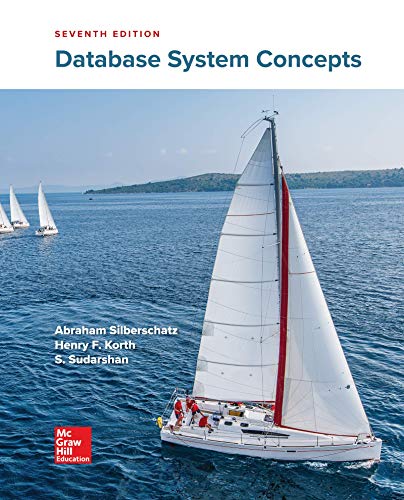
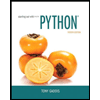
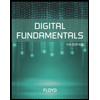
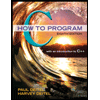
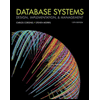
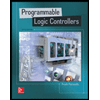