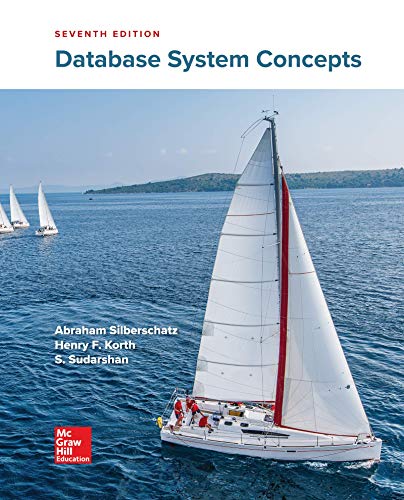
- Observe the following code and answer the following questions.
import java.io.*;
import java.net.*;
import java.util.Date;
public class TimeServer{
public static void main(String args[]) throws Exception{
ServerSocket server = new ServerSocket(9876);
while (true){
Socket client = server.accept(); //blocks until a client connects
PrintStream ps = new PrintStream( client.getOutputStream() );
ps.println("Time from server:" + new Date());
client.close();
}
}
}
- What type of server this is? Is it single or multi-threaded?
2. What should be done according to you if the number of clients to this server increases? Modify the server code so that the increases number of clients can be handled by this server.
3. Explain where in your code you ensured that for every incoming request from a client, a new thread is created to serve that client

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 3 images

- import org.firmata4j.IODevice;import org.firmata4j.Pin;import org.firmata4j.firmata.FirmataDevice;import org.firmata4j.ssd1306.SSD1306;import java.io.IOException;import java.util.HashMap;import java.util.TimerTask;public class minorproj extends TimerTask {static String recLog = "\"/dev/cu.usbserial-0001\"";static IODevice myGroveBoard;private final SSD1306 theOledObject;private Pin MoistureSensor;private Pin WaterPump;private Pin Button;private int sampleCount;public minorproj(SSD1306 theOledObject, Pin Button, Pin MoistureSensor, Pin WaterPump) {this.theOledObject = theOledObject;this.Button = Button;this.MoistureSensor = MoistureSensor;this.WaterPump = WaterPump;this.sampleCount = 0;}double startTime = System.currentTimeMillis();@Overridepublic void run() {while (true) {{// check if button is pressedif (this.Button.getValue() == 1) {break; // exit loop and stop program}String VolValue = String.valueOf(MoistureSensor.getValue());System.out.println("Moisture Sensor Value:" +…arrow_forwardPlease answer the follwoing regarding the java code: When looking at the code below, assume that the MyThread and MyRunnable classes are correctly implemented in other files. How many threads are there in the program shown below? (Be careful to consider ALL the threads!)arrow_forwardJava provides a mechanism that enables a program to be executed from the command line, as an application. Java runtime execution works on the basis that the class executed must have a: (constructor? inner class? method?) named `main’ that is: (final? static? local?) , public and void. This should take an : (StringBuffer? ArrayList? Array?) of Strings as its only argument. Execution is begun by (calling this method? clicking the BlueJ Run button? selecting this method from the dropdown menu?) . Select the correct answer.arrow_forward
- For the following code I get an error: Exception in thread "main" java.lang.Error: Unresolved compilation problems: The type LinkedListIn is not generic; it cannot be parameterized with arguments <String> The type LinkedListIn is not generic; it cannot be parameterized with arguments <> at linkedList.LinkedListTester.main(LinkedListTester.java:5) How can I fix it? package linkedList; public class LinkedListTester { public static void main(String[] args) { LinkedListIn<String> list = new LinkedListIn<>(); list.display(); list.add("Station 1"); list.add("Station 2"); list.add("Station 3"); list.add("Station 4"); // Print the linked list System.out.println("All Stations: " + list); // Accessing the first item System.out.println("First stop: " + list.getFirst()); // Accessing the last item System.out.println("Last stop: " + list.getLast()); // Removing the first item System.out.println("Left this station: " + list.removeFirst()); //…arrow_forwardSolution question number 2arrow_forwardConsider the below Java code. import public class Semaphore Demo java.util.concurrent.Semaphore; Semaphore binarySema= new Semaphore (1); public static void main (String args[]) { final SemaphoreDemo sema DemoObj= new Semaphore Demo (); Thread td1= new Thread() ( public void run () { } }} } }; Thread td2= new Thread () { @Override public void run () { sema DemoObj.testMutualExclusion (); } sema DemoObj.testMutualExclusion (); tdl.start(); td2.start (); private void testMutualExclusion () { try { binarySema.acquire(); System.out.println (Thread.currentThread().getName()+ "inside the region of mutual exclusive."); Thread.sleep (1000); } catch (InterruptedException e) { ie.printStackTrace(); } finally ( System.out.println (Thread.currentThread().getName() + "outside the region of mutual exclusive."); What would be the output of the program?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
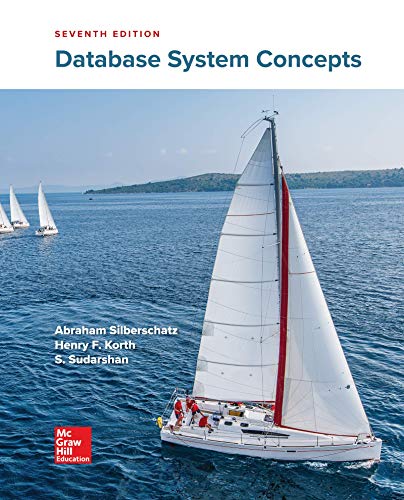
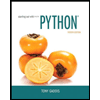
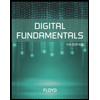
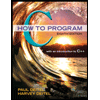
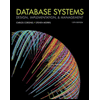
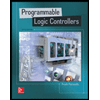