Need help fixing my code!!only modify under TODO!! Food_wastage_tracker_backend.cc #include "food_wastage_tracker_backend.h" #include #include "food_wastage_record.h" #include "food_wastage_report.h" #include "server_utils/rapidjson/document.h" // rapidjson's DOM-style API #include "server_utils/rapidjson/prettywriter.h" // for stringify JSON #include "server_utils/rapidjson/rapidjson.h" #include "server_utils/rapidjson/stringbuffer.h" // wrapper of C stream for prettywriter as output #include "server_utils/rapidjson/writer.h" // Util function to convert a FoodWastageRecord class object into a serialized // JSON object. void SerializeFoodWastageRecordToJSON( const FoodWastageRecord &record, rapidjson::Writer *writer) { writer->StartObject(); writer->String("date_"); // DO NOT MODIFY std::string date; // TODO 1. Use the accessor/getter function for date from the // FoodWastageRecord class object to get the date and store it in the date // string declared above. FoodWastageRecord record; date = record.Date(); writer->String(date.c_str()); writer->String("meal_"); // DO NOT MODIFY std::string meal; // TODO 2. Use the accessor/getter function for meal from the // FoodWastageRecord class object to get the meal and store it in the meal // string declared above. meal = record.Meal(); writer->String(meal.c_str()); writer->String("food_name_"); // DO NOT MODIFY std::string food_name; // TODO 3. Use the accessor/getter function for food name from the // FoodWastageRecord class object to get the food name and store it in the // food_name string declared above. food_name = record.FoodName(); writer->String(food_name.c_str()); writer->String("qty_in_oz_"); // DO NOT MODIFY double quantity; // TODO 4. Use the accessor/getter function for quantity from the // FoodWastageRecord class object to get the quantity and store it in the // quantity variable declared above. quantity = record.QuantityInOz(); writer->Double(quantity); writer->String("wastage_reason_"); // DO NOT MODIFY std::string wastage_reason; // TODO 5. Use the accessor/getter function for wastage reason from the // FoodWastageRecord class object to get the wastage reason and store it in // the wastage_reason string declared above. wastage_reason = record.WastageReason(); writer->String(wastage_reason.c_str()); writer->String("disposal_mechanism_"); // DO NOT MODIFY std::string disposal_mechanism; // TODO 6. Use the accessor/getter function for disposal mechanism from the // FoodWastageRecord class object to get the disposal mechanism and store it // in the disposal_mechanism string declared above. disposal_mechanism = record.DisposalMechanism(); writer->String(disposal_mechanism.c_str()); writer->String("cost_"); // DO NOT MODIFY double cost; // TODO 7. Use the accessor/getter function for cost from the // FoodWastageRecord class object to get the cost and store it in the cost // variable declared above. cost = record.Cost(); writer->Double(cost); writer->EndObject();
Need help fixing my code!!only modify under TODO!!
Food_wastage_tracker_backend.cc
#include "food_wastage_tracker_backend.h"
#include <filesystem>
#include "food_wastage_record.h"
#include "food_wastage_report.h"
#include "server_utils/rapidjson/document.h" // rapidjson's DOM-style API
#include "server_utils/rapidjson/prettywriter.h" // for stringify JSON
#include "server_utils/rapidjson/rapidjson.h"
#include "server_utils/rapidjson/stringbuffer.h" // wrapper of C stream for prettywriter as output
#include "server_utils/rapidjson/writer.h"
// Util function to convert a FoodWastageRecord class object into a serialized
// JSON object.
void SerializeFoodWastageRecordToJSON(
const FoodWastageRecord &record,
rapidjson::Writer<rapidjson::StringBuffer> *writer) {
writer->StartObject();
writer->String("date_"); // DO NOT MODIFY
std::string date;
// TODO 1. Use the accessor/getter function for date from the
// FoodWastageRecord class object to get the date and store it in the date
// string declared above.
FoodWastageRecord record;
date = record.Date();
writer->String(date.c_str());
writer->String("meal_"); // DO NOT MODIFY
std::string meal;
// TODO 2. Use the accessor/getter function for meal from the
// FoodWastageRecord class object to get the meal and store it in the meal
// string declared above.
meal = record.Meal();
writer->String(meal.c_str());
writer->String("food_name_"); // DO NOT MODIFY
std::string food_name;
// TODO 3. Use the accessor/getter function for food name from the
// FoodWastageRecord class object to get the food name and store it in the
// food_name string declared above.
food_name = record.FoodName();
writer->String(food_name.c_str());
writer->String("qty_in_oz_"); // DO NOT MODIFY
double quantity;
// TODO 4. Use the accessor/getter function for quantity from the
// FoodWastageRecord class object to get the quantity and store it in the
// quantity variable declared above.
quantity = record.QuantityInOz();
writer->Double(quantity);
writer->String("wastage_reason_"); // DO NOT MODIFY
std::string wastage_reason;
// TODO 5. Use the accessor/getter function for wastage reason from the
// FoodWastageRecord class object to get the wastage reason and store it in
// the wastage_reason string declared above.
wastage_reason = record.WastageReason();
writer->String(wastage_reason.c_str());
writer->String("disposal_mechanism_"); // DO NOT MODIFY
std::string disposal_mechanism;
// TODO 6. Use the accessor/getter function for disposal
// FoodWastageRecord class object to get the disposal mechanism and store it
// in the disposal_mechanism string declared above.
disposal_mechanism = record.DisposalMechanism();
writer->String(disposal_mechanism.c_str());
writer->String("cost_"); // DO NOT MODIFY
double cost;
// TODO 7. Use the accessor/getter function for cost from the
// FoodWastageRecord class object to get the cost and store it in the cost
// variable declared above.
cost = record.Cost();
writer->Double(cost);
writer->EndObject();
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

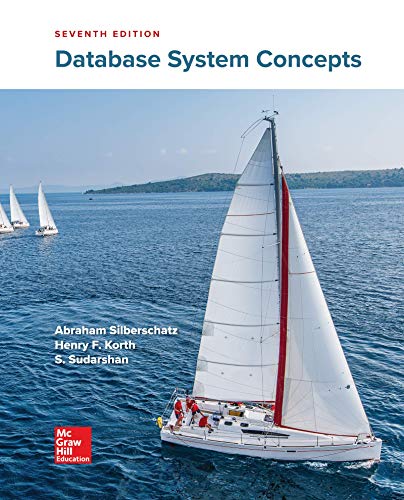
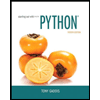
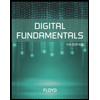
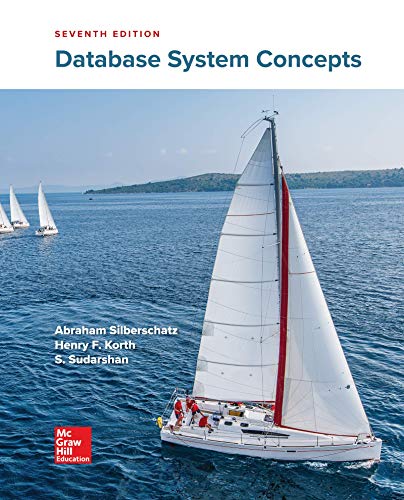
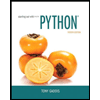
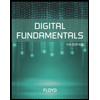
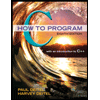
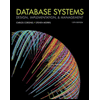
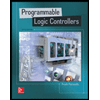