need help explaining each and every line of the code functionality ========================================================== import React, { useEffect, useContext, useState } from "react"; import { AppContext } from "../../context/applicationContext"; import LoadingIndicator from "../../components/LoadingIndicator"; // import the API from apiUtil.js import { getOthersFeedsApi } from "../../util/ApiUtil"; import MyProfile from "../../components/MyProfile"; import AddFeed from "../../components/AddFeed"; import InfiniteScroll from "react-infinite-scroll-component"; import FeedCard from "../../components/FeedCard"; const Dashboard = () => { // name spacing for the child component using UseContext hook const appContext = useContext(AppContext); // access the token which is present inside our cookies const token = appContext.getSession(); // access the user data by calling getUserData() function from AppContext const userData = appContext.getUserData(); // all the other user's feed are captured and stored inside an Array const [feedsData, setFeedsData] = useState([]); const [pageNumber, setPageNumber] = useState(0); //State variable that holds a boolean indicating whether thee are more feeds to be displayed const [hasMore, setHasMore] = useState(true); // Function is going to load the feeds of other user's const getOthersFeeds = async (loadPageNumber) => { if (loadPageNumber === 0) { setFeedsData([]); } const apiResponse = await getOthersFeedsApi(token, loadPageNumber); console.log(apiResponse); if (apiResponse.status === 1) { let feedsDataNew = []; if (loadPageNumber !== 0) { feedsDataNew = feedsData; } feedsDataNew.push(...apiResponse.payLoad.content); setFeedsData(feedsDataNew); setPageNumber(loadPageNumber + 1); if (loadPageNumber + 1 === apiResponse.payLoad.totalPages) { setHasMore(false); } else { setHasMore(true); } } }; //set the page title and fetch the feeds for the Dashboard useEffect(() => { document.title = "Home | Feed App"; getOthersFeeds(0); }, []); // if the data is being fetched from the backend, it displays loading indicator if (!userData) { return ; } return ( {/* {#MyProfile Component} */} {/* {#AddFeed Component} */} {/* {#FeedCard Component} */} getOthersFeeds(pageNumber)} hasMore={hasMore} endMessage={ Yay! You have seen it all. } refreshFunction={() => getOthersFeeds(0)} pullDownToRefresh pullDownToRefreshThreshold={50} pullDownToRefreshContent={ ↓ Pull down to refresh } releaseToRefreshContent={ ↑ Release to refresh } > {feedsData.map( ({ feedId, picture, content, createdOn, feedMetaData, user }) => ( ) )} ) } export default Dashboard
need help explaining each and every line of the code functionality ========================================================== import React, { useEffect, useContext, useState } from "react"; import { AppContext } from "../../context/applicationContext"; import LoadingIndicator from "../../components/LoadingIndicator"; // import the API from apiUtil.js import { getOthersFeedsApi } from "../../util/ApiUtil"; import MyProfile from "../../components/MyProfile"; import AddFeed from "../../components/AddFeed"; import InfiniteScroll from "react-infinite-scroll-component"; import FeedCard from "../../components/FeedCard"; const Dashboard = () => { // name spacing for the child component using UseContext hook const appContext = useContext(AppContext); // access the token which is present inside our cookies const token = appContext.getSession(); // access the user data by calling getUserData() function from AppContext const userData = appContext.getUserData(); // all the other user's feed are captured and stored inside an Array const [feedsData, setFeedsData] = useState([]); const [pageNumber, setPageNumber] = useState(0); //State variable that holds a boolean indicating whether thee are more feeds to be displayed const [hasMore, setHasMore] = useState(true); // Function is going to load the feeds of other user's const getOthersFeeds = async (loadPageNumber) => { if (loadPageNumber === 0) { setFeedsData([]); } const apiResponse = await getOthersFeedsApi(token, loadPageNumber); console.log(apiResponse); if (apiResponse.status === 1) { let feedsDataNew = []; if (loadPageNumber !== 0) { feedsDataNew = feedsData; } feedsDataNew.push(...apiResponse.payLoad.content); setFeedsData(feedsDataNew); setPageNumber(loadPageNumber + 1); if (loadPageNumber + 1 === apiResponse.payLoad.totalPages) { setHasMore(false); } else { setHasMore(true); } } }; //set the page title and fetch the feeds for the Dashboard useEffect(() => { document.title = "Home | Feed App"; getOthersFeeds(0); }, []); // if the data is being fetched from the backend, it displays loading indicator if (!userData) { return ; } return ( {/* {#MyProfile Component} */} {/* {#AddFeed Component} */} {/* {#FeedCard Component} */} getOthersFeeds(pageNumber)} hasMore={hasMore} endMessage={ Yay! You have seen it all. } refreshFunction={() => getOthersFeeds(0)} pullDownToRefresh pullDownToRefreshThreshold={50} pullDownToRefreshContent={ ↓ Pull down to refresh } releaseToRefreshContent={ ↑ Release to refresh } > {feedsData.map( ({ feedId, picture, content, createdOn, feedMetaData, user }) => ( ) )} ) } export default Dashboard
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
need help explaining each and every line of the code functionality
==========================================================
import React, { useEffect, useContext, useState } from "react";
import { AppContext } from "../../context/applicationContext";
import LoadingIndicator from "../../components/LoadingIndicator";
// import the API from apiUtil.js
import { getOthersFeedsApi } from "../../util/ApiUtil";
import MyProfile from "../../components/MyProfile";
import AddFeed from "../../components/AddFeed";
import InfiniteScroll from "react-infinite-scroll-component";
import FeedCard from "../../components/FeedCard";
const Dashboard = () => {
// name spacing for the child component using UseContext hook
const appContext = useContext(AppContext);
// access the token which is present inside our cookies
const token = appContext.getSession();
// access the user data by calling getUserData() function from AppContext
const userData = appContext.getUserData();
// all the other user's feed are captured and stored inside an Array
const [feedsData, setFeedsData] = useState([]);
const [pageNumber, setPageNumber] = useState(0);
//State variable that holds a boolean indicating whether thee are more feeds to be displayed
const [hasMore, setHasMore] = useState(true);
// Function is going to load the feeds of other user's
const getOthersFeeds = async (loadPageNumber) => {
if (loadPageNumber === 0) {
setFeedsData([]);
}
const apiResponse = await getOthersFeedsApi(token, loadPageNumber);
console.log(apiResponse);
if (apiResponse.status === 1) {
let feedsDataNew = [];
if (loadPageNumber !== 0) {
feedsDataNew = feedsData;
}
feedsDataNew.push(...apiResponse.payLoad.content);
setFeedsData(feedsDataNew);
setPageNumber(loadPageNumber + 1);
if (loadPageNumber + 1 === apiResponse.payLoad.totalPages) {
setHasMore(false);
} else {
setHasMore(true);
}
}
};
//set the page title and fetch the feeds for the Dashboard
useEffect(() => {
document.title = "Home | Feed App";
getOthersFeeds(0);
}, []);
// if the data is being fetched from the backend, it displays loading indicator
if (!userData) {
return <LoadingIndicator />;
}
return (
<main className="grid grid-cols-1 lg:grid-cols-2 gap-6 my-12 mx-0 md:mx-12 w-2xl container px-2 mx-auto">
{/* {#MyProfile Component} */}
<MyProfile />
<article>
{/* {#AddFeed Component} */}
<AddFeed />
{/* {#FeedCard Component} */}
<InfiniteScroll
dataLength={feedsData.length}
next={() => getOthersFeeds(pageNumber)}
hasMore={hasMore}
endMessage={
<p className="text-center">
<b>Yay! You have seen it all.</b>
</p>
}
refreshFunction={() => getOthersFeeds(0)}
pullDownToRefresh
pullDownToRefreshThreshold={50}
pullDownToRefreshContent={
<h3 className="text-center">↓ Pull down to refresh</h3>
}
releaseToRefreshContent={
<h3 className="text-center">↑ Release to refresh</h3>
}
>
<div className="mt-3">
{feedsData.map(
({ feedId, picture, content, createdOn, feedMetaData, user }) => (
<FeedCard
key={feedId}
feedId={feedId}
picture={picture}
content={content}
createdOn={createdOn}
username={user.username}
firstName={user.firstName}
lastName={user.lastName}
profilePicture={user.profile.picture}
feedMetaData={feedMetaData}
/>
)
)}
</div>
</InfiniteScroll>
</article>
</main>
)
}
export default Dashboard
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
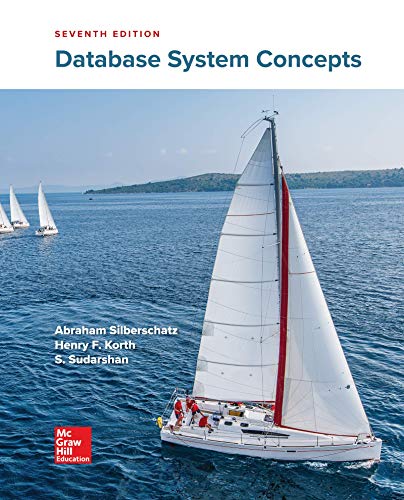
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
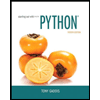
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
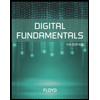
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
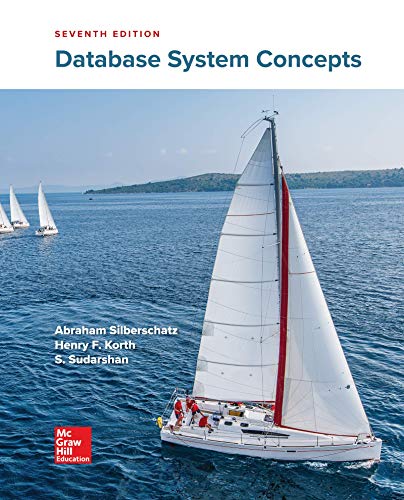
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
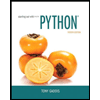
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
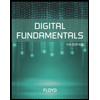
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
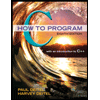
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
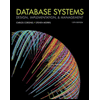
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
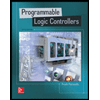
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education