n a program, write a function that access two arguments: a list, and a number n. Assume that the list contains numbers. The function should display all of the numbers in the list that are greater than the number n Modularity – Your program should have at least these two functions: main – should accept input of a series of 10 integers from the user and an integer n that will be the search number. main should call the function display_larger and pass 2 arguments: the 10 integers stored in a list, and the number n. display_larger – should accept the 2 parameters from main. Using a loop, the function should compare all numbers in the list of number from the user to n. If the number is larger than n, it should be stored in a second list containing only the numbers that are greater than n. A third list with those less than n should also be made and printed out. The lists should be sorted and then printed, as well. Sorted lists should be done in ascending order and then is descending order. This function should display: The original list, n, and the list of numbers greater th
In a program, write a function that access two arguments: a list, and a number n. Assume that the list contains numbers. The function should display all of the numbers in the list that are greater than the number n
Modularity – Your program should have at least these two functions:
main – should accept input of a series of 10 integers from the user and an integer n that will be the search number. main should call the function display_larger and pass 2 arguments: the 10 integers stored in a list, and the number n.
display_larger – should accept the 2 parameters from main. Using a loop, the function should compare all numbers in the list of number from the user to n. If the number is larger than n, it should be stored in a second list containing only the numbers that are greater than n. A third list with those less than n should also be made and printed out. The lists should be sorted and then printed, as well. Sorted lists should be done in ascending order and then is descending order.
This function should display: The original list, n, and the list of numbers greater than n.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

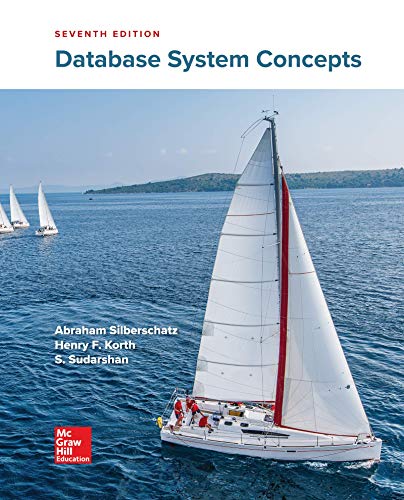
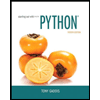
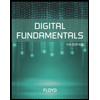
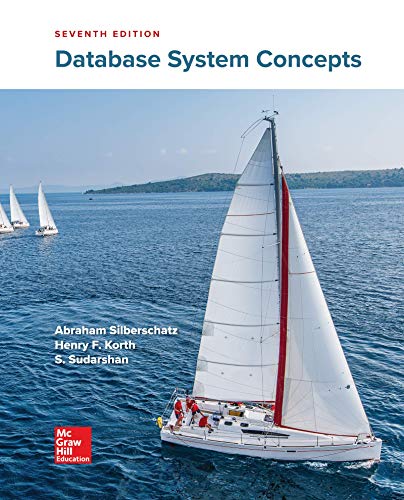
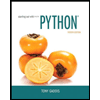
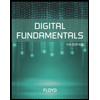
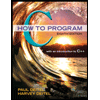
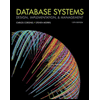
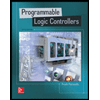