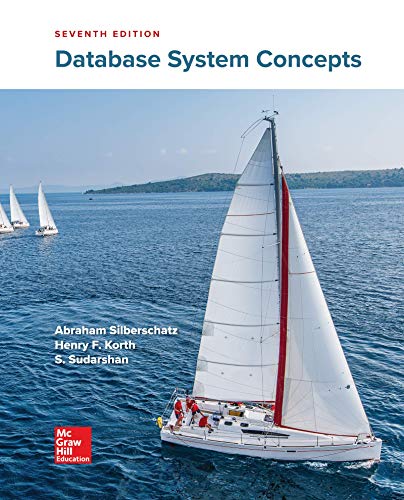
my code is working but i want to remove all the processes from main and put them into a different function.
#include <iostream>
#include <iomanip>
using namespace std;
void holdScreen();
void developerInfo();
int askmultiplyQuestion();
double isGivenSolutionCorrect(int givenAnswer, int expectedAnswer);
int numberOfCorrectResponses = 0;
int numberOfResponses = 0;
double percentageOfCorrectAnswers();
//***************************************************************
//
// Function: main
//
// Description: The main function of the program
//
// Parameters: None
//
// Returns: Zero (0)
//
//**************************************************************
int main()
{
// Do not delete this statement
developerInfo();
while (numberOfResponses < 10) {
int expectedAnswer = askmultiplyQuestion();
do {
int givenAnswer;
cin>> givenAnswer;
numberOfResponses += 1;
if (isGivenSolutionCorrect(givenAnswer, expectedAnswer)) {
numberOfCorrectResponses += 1;
break;
}
} while (numberOfResponses < 10);
}
// Terminate Program
holdScreen();
return 0;
}
int askmultiplyQuestion() {
int number1 = rand() % 10;
int number2 = rand() % 10;
cout << "How much is " << number1 << " times " << number2 << "? ";
return number1 * number2;
}
double isGivenSolutionCorrect(int givenAnswer, int expectedAnswer){
if (givenAnswer == expectedAnswer) {
char const *responses_values[] = { "Very good!", "Excellent!", "Nice work!", "Keep up the good work!" ,"Way to go!"};
int numberOfResponses = (sizeof(responses_values) / sizeof(responses_values[0]));
cout << responses_values[rand() % numberOfResponses] << endl;
return true;
}
else {
char const *responses_values[] = { "That is incorrect!", "No. Please try again!", "Wrong, Try once more!", "No. Don’t give up!" ,"Incorrect. Keep trying!"};
int numberOfResponses = (sizeof(responses_values) / sizeof(responses_values[0]));
cout << responses_values[rand() % numberOfResponses] << endl;
return false;
}
double percentageOfCorrectAnswers = double(numberOfCorrectResponses) / double(numberOfResponses);{
if (percentageOfCorrectAnswers < 0.75)
{ cout << "Please ask your teacher for extra help." << endl; }
else { cout << "Good Job!!" << endl; }
return 0;
}
}
//*********************************************************************
//
// Function: holdScreen
//
// Description: The hold screen function
//
// Parameters: None
//
// Returns: N/A
//
//*********************************************************************
void holdScreen()
{
char ch;
cout << "\nPress any key to exit... ";
ch = getchar();
}
//***************************************************************
//
// Function: developerInfo
//
// Description: The developer's information
//
// Parameters: None
//
// Returns: N/A
//
//**************************************************************
void developerInfo()
{
cout << "Name: Hussain Aldulaimi" << endl;
cout << "Course: COSC 1337
cout << "Program: Eight"
<< endl
<< endl;
} // End of developerInfo

Step by stepSolved in 3 steps with 1 images

- Choose if each of the following is true or false:Dynamically binding data to virtual functions is limited to pointers and references.arrow_forwardAdd a function to get the CPI values from the user and validate that they are greater than 0. 1. Declare and implement a void function called getCPIValues that takes two float reference parameters for the old_cpi and new_cpi. 2. Move the code that reads in the old_cpi and new_cpi into this function. 3. Add a do-while loop that validates the input, making sure that the old_cpi and new_cpi are valid values. + if there is an input error, print "Error: CPI values must be greater than 0." and try to get data again. 4. Replace the code that was moved with a call to this new function. - Add an array to accumulate the computed inflation rates 1. Declare a constant called MAX_RATES and set it to 20. 2. Declare an array of double values having size MAX_RATES that will be used to accumulate the computed inflation rates. 3. Add code to main that inserts the computed inflation rate into the next position in the array. 4. Be careful to make sure the program does not overflow the array. - Add a…arrow_forwardAssignment-3 With the help of a C program show how to typecast a void * to an int * . Attach the code and output and upload the file on classroom.arrow_forward
- How can I quickly copy a group of shared references from one array into another in C++? Create a list of potential responses to the challenge at hand. Does copying shared pointers also copy the objects they manage? Explainarrow_forwardHello, I was making a hangman simulation in C++. The code runs, but not fully. Could you identify the error and fix it? #include<iostream>#include<cstring> // for string class functions#include<fstream>#include <cctype>using namespace std; int main(){// define variable to get the response from user "Yes" or "No"string response;// Define index variableint w = 0;// define number of words that need to be guessed by the user assume 4const int WORDS = 4;// loopdo{// we will define the hangman bodyconst char body[] = "o/|\\|\\";// here we define the wordsstring words[WORDS] = {"MACAW", "SADDLE", "TOASTER", "XENOICIDE"};// fetch size or lengthstring xword(words[w].length(),'*');// define iterator to fetch the wordsstring::iterator i, ix = xword.begin();// define number of words to prompt for the userchar letters[26]={"\0"};// Now we define the variables which will be used in the simulationint n =0, xcount = xword.length();bool found = false, solved = false, hung =…arrow_forwardYou must use one other model (math/random).arrow_forward
- Writ a program with c++ language to do the following tasks : The user can input one original image and target scales . According to the user’s requirement, this image can resized to the target scale. The program can show the original image and the resized image. *The core function should be Bilinear_interpolation(original image, target height, target width) The output of this function is the resized image and then the user can save it. *You can use image processing libraries to assist your codes, such as Opencv. The functions included in the libraries which are off‐the‐shelf can be used to read images, operate images and save images.arrow_forwardStructures The circle has two data members, a Point representing the center of the circle and a float value representing the radius as shown below. typedef struct{ Point center; float radius; }Circle; Make a c project sorting it into main.c circle.h, circle.c and implement the following functions: a. float diameter(Circle circ); //computes the diameter of a circle. b. float area(Circle circ); //computes for area of a circle c. float circumference(Circle circ);//computes for the circumference of a circle. Also add the basic functiona: initCircle(), createCircle() and displayCircle()arrow_forwardIN C++ Demonstrate overriding and overloading functions using the class you built in previous activities. Explain your work by commenting your class. Submit a screenshot showing the output of a class that has overloading and overriding functions.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
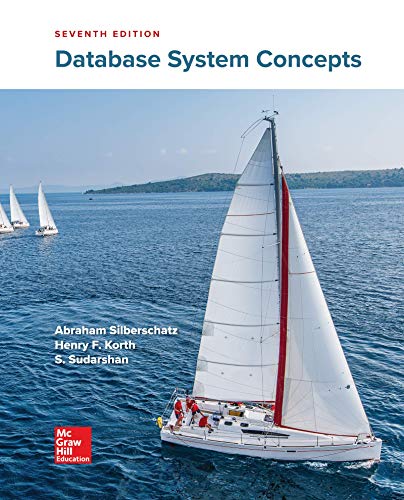
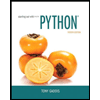
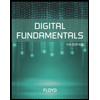
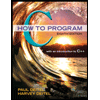
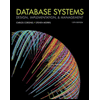
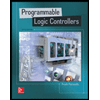