Module/Week 7 ASSIGNMENT (ARRAYS) Use functional decomposition to write a C++ program that asks the user to enter his or her weight and the name of a planet. Use an enumerated type called planetTyps to represent the planets and use a switch statement that takes as its condition a planetType variable. The program, via the switch statement, then outputs how much the user would weigh on that planet. The following table gives the factor by which the weight must be multiplied for each planet. Your program must have the following functions in it: GetUserlnput0, ConvertinputTOPlanetType0, and QutputWeightO your switch statement must be in QutputWeight0). I will let you determine what each function's parameters and return types should be. The program will output an error message if the user does not type a correct planet name. The prompt and the error message will make it clear to the user how a planet name must be entered. Use proper formatting and appropriate comments in your code. The output must be labeled clearly and formatted neatly. The following table gives the factor by which the weight must be multiplied for each planet: Mercury 0.4155 Venus 0.8975 Earth 1.0 Мoon 0.166 Mars 0.3507 Jupiter 2.5374 Saturn 1.0677 Uranus 0.8947 Neptune 1.1794 Pluto 0.0899
Module/Week 7 ASSIGNMENT (ARRAYS)
Use functional decomposition to write a C++
Your program must have the following functions in it: GetUserInput(), ConvertInputToPlanetType(), and OutputWeight() (your switch statement must be in OutputWeight()). I will let you determine what each function’s parameters and return types should be. The program will output an error message if the user does not type a correct planet name. The prompt and the error message will make it clear to the user how a planet name must be entered.
Use proper formatting and appropriate comments in your code. The output must be labeled clearly and formatted neatly.
The following table gives the factor by which the weight must be multiplied for each planet:


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

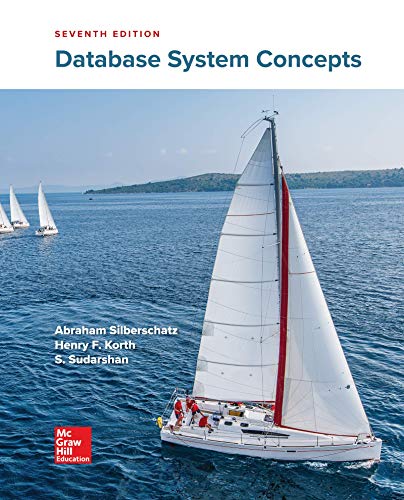
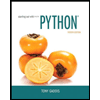
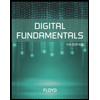
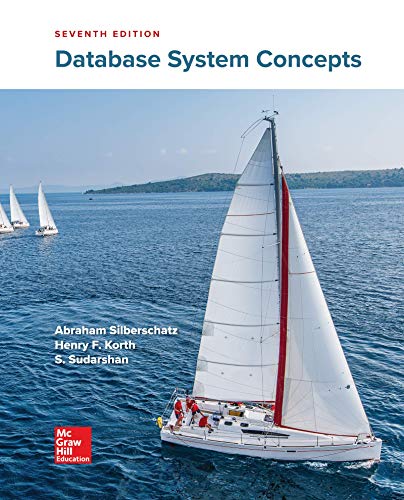
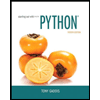
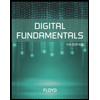
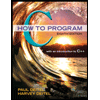
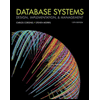
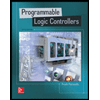