Make a python class called CellPhone. The init() method for CellPhone needs to store two attributes: brand, and model. Make two attribute called call_time (representing total calls duration) and txt_count (representing total text message count)which both start at 0 for each new CellPhone instance. Define a method named call() that gets a call duration and updates the call_time attribute, then prints out the total calls duration so far. Define another method named txt() that each time we call it just updates the txt_count attribute by adding 1 message to that, then prints the total message count so far. In the end, create a new instance of CellPhone and run each method once (use any number for call duration argument).
Make a python class called CellPhone. The init() method for CellPhone needs to store two attributes: brand, and model. Make two attribute called call_time (representing total calls duration) and txt_count (representing total text message count)which both start at 0 for each new CellPhone instance.
Define a method named call() that gets a call duration and updates the call_time attribute, then prints out the total calls duration so far.
Define another method named txt() that each time we call it just updates the txt_count attribute by adding 1 message to that, then prints the total message count so far.
In the end, create a new instance of CellPhone and run each method once (use any number for call duration argument).

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

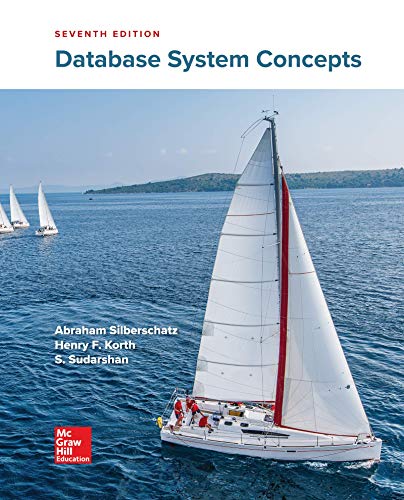
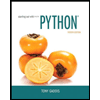
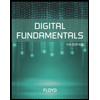
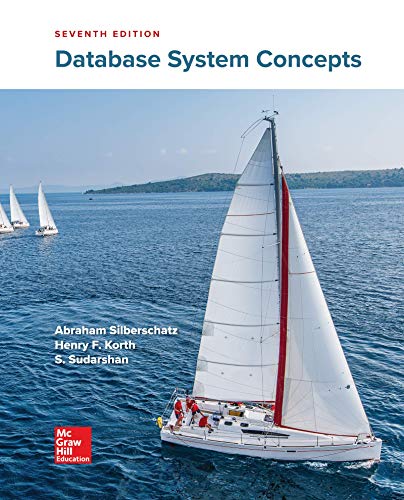
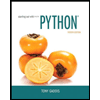
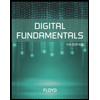
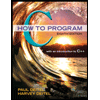
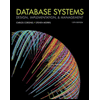
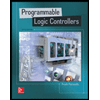