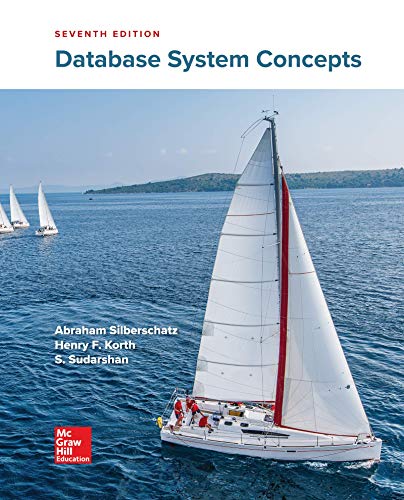
6.31 Lab training: Unit tests to evaluate your program
Auto-graded
- is named correctly and has the correct parameters and return type
- calculates and returns the correct value (or prints the correct output)
In Python labs, the line if __name__ == '__main__': is used to separate the main code from the functions' code so that each function can be unit tested.
This example lab uses multiple unit tests to test the kilo_to_pounds() function.
Complete a program that takes a weight in kilograms as input, converts the weight to pounds, and then outputs the weight in pounds. 1 kilogram = 2.204 pounds (lbs).
Ex: If the input is:
10the output is:
22.040000000000003 lbsNote: Your program must define the function
def kilo_to_pounds(kilos)
The program below has an error in the kilo_to_pounds() function.
-
Try submitting the program for grading (click "Submit mode", then "Submit for grading"). Notice that the first two test cases fail, but the third test case passes. The first test case fails because the program outputs the result from the kilo_to_pounds() function, which has an error. The second test case uses a Unit test to test the kilo_to_pounds() function, which fails.
-
Change the kilo_to_pounds() function to multiply the variable kilos by 2.204, instead of dividing. The return statement should be: return (kilos * 2.204); Submit again. Now the test cases should all pass.
Note: A common error is to mistype a function name with the incorrect capitalization. Function names are case sensitive, so if a lab program asks for a kilo_to_pounds() function, a kilo_To_Pounds() function that works for you in develop mode will result in a failed unit test (the unit test will not be able to find kilo_to_pounds()).
Use Python, please.
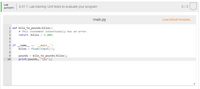

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Problem 2: Simple Interest The formula for computing the future value of an amount that is increasing due to accumulating simple interest is F=P(1+rxi) Where F is the future value, P is the present value, is the annual interest rate (decimal value not percent), and is the total time period in years. Write a function named simpleInterest that accepts values for the three variables P, and and assings them to the output variable futureValue Function Code to call your function > Save C Reset function [futureValue] =simpleInterest (presentValue, interest RatePercent, time Years) %Enter commands to perform the computation and assign the results 3 %to the output variable defined in the function command in line 1. 1 [futureValue] =simpleInterest (10000,5.25,5) My Solutions > MATLAB Documentation C Reset Run Functionarrow_forwardC++arrow_forwardWithin the tutorial casino craps game pseudocode, where should you place the pseudocode that performs the task of 'Get if the player won or lost', and how (in pseudocode) should this task be implemented? a.) In Function play, with pseudocode function play() ... set a local variable to won or lost b.) In Function play, with pseudocode function play() ... return if the player won or lost c.) In Loop until Games Played = Times to Play, with pseudocode Loop until Games Played = Times to Play ... If the player won ... Set "Get if the player won or lost" to won Else if player lost ... Set "Get if the player won or lost" to lost d.) In Loop until Games Played = Times to Play, with pseudocode Loop until Games Played = Times to Play ... If the player won ... Else if player lost ... Set "Get if the player won or lost" to won or lostarrow_forward
- Void functions do not return any value when they are called.True or falsearrow_forwarder 6 Functions Programming Exercises te 1. Rectangle Area ngle Area The area of a rectangle is calculated according to the following formula: Area = Width X Length Design a function that accepts a rectangle's width and length as arguments and returns the rectangle's area. Use the function in a program that prompts the user to enter the rectangle's width and length, and then displays the rectangle's area.arrow_forwardPython without Def function Problem Statement Define and implement a function time_delta that takes two string parameters (time1 and time2) and returns the difference between them (as a string). You may assume that the second time will always be after the first time, both times will always be on the same date, and military time will be used (no a.m. or p.m.). Examples time_delta(04:15:36, 16:35:27) ➞ (12:19:51) time_delta(02:06:05, 06:09:10) ➞ (04:03:05) Sample Input 1 "04:15:36" "16:35:27" Sample Output 1 "12:19:51"arrow_forward
- Complete function PrintPopcornTime(), with int parameter bagOunces, and void return type. If bagOunces is less than 3, print "Too small". If greater than 10, print "Too large". Otherwise, compute and print 6* bagOunces followed by seconds". End with a newline. Example output for ounces = 7: 42 seconds 1 #include NM & in 107 2 3 void PrintPopcornTime (int bagOunces) { 4 requiriturgy 5 /* Your solution goes here */ 6 7} 8 9 int main(void) { 10 int userOunces; 11 12 scanf("%d", &userOunces); 13 14 15 16} PrintPopcornTime (userOunces); return 0;arrow_forwardLogical variables: On Time or Delayed? Complete the function WhatlsIt such that: The output logical variable on Time is true only if no Traffic is true and gasEmpty is false. The output logical variable delayed is false only if no Traffic is true and gasEmpty is false. Restriction: Logical expressions must be used. Do not use if statements. Function > 1 function [onTime, delayed] = WhatIsIt (noTraffic, gasEmpty) 2 onTime = 3 delayed = 4 5 end Save C Reset MATLAB Documentationarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
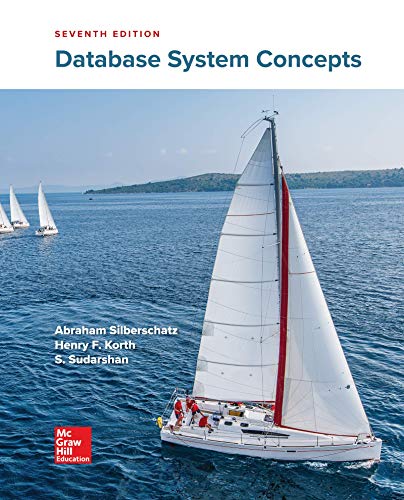
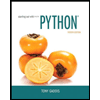
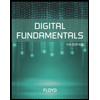
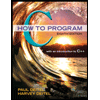
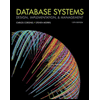
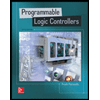