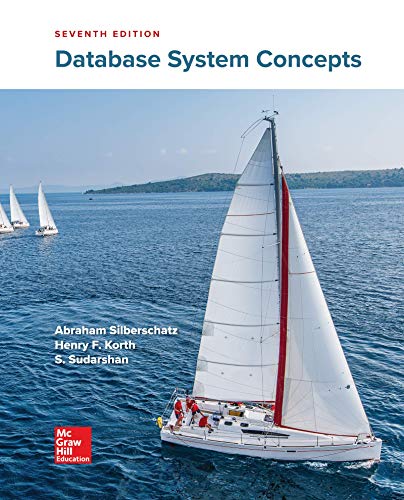
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
C
In this unit, you will create 3 functions:
- main(): Call print_stack() and then call f1(10).
- f1(unsigned int): Call print_stack(). Define a local string containing "ABCD". Call f2() passing the string variable as its parameter.
- f2(char *): Call print_stack().
Furthermore, define a macro OFFSET=32 in this unit and use that macro in place of the offset parameter whenever you call print_stack(). This helps you test your code using different offsets without having to change it throughout your code.
Make sure the code compiles and there must be no errors. I want to see the full output in the terminal running, so I know that the code compiles.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
There is an error on line 12. I need that error to be fixed. You can see that error on the image attached.
![### C Programming: Basic Stack Operations
#### Source Code
The following code snippet demonstrates basic stack operations using a C program:
```c
#include<stdio.h>
#include<stdlib.h>
#define OFFSET 32
int Top=-1, inp_array[OFFSET];
void push();
void pop();
void print_stack();
int main() {
int choice;
while(true) {
printf("\nOperations performed by Stack");
printf("\n1.push the element\n2.pop the element\n3.Display Stack\n4.End");
printf("\nInput the choice:");
scanf("%d",&choice);
switch(choice) {
case 1: push();
break;
case 2: pop();
break;
case 3: print_stack();
break;
case 4: exit(0);
default: printf("\nInvalid choice!!!");
}
}
}
```
#### Explanation and Error
- **Header Files and Constants**: Includes the standard input-output and standard library header files. Defines a constant `OFFSET` with a value of 32.
- **Global Variables**: Declares an integer `Top` initialized to -1 and an integer array `inp_array` sized by `OFFSET`.
- **Function Declarations**:
- `push()`: To add elements to the stack.
- `pop()`: To remove elements from the stack.
- `print_stack()`: To display elements in the stack.
- **Main Function**:
- Uses a `while` loop to continually perform stack operations based on user input.
- Prompts the user for a choice and executes corresponding functions.
- **Error Notice**: The code contains an error due to the use of `true` without declaration. C does not inherently recognize `true`. To resolve this, replace `while(true)` with `while(1)` for infinite loop functionality.
#### Compiler Output
On the right, the panel shows the compiler output:
- An error indicating `'true' undeclared` in the main function at line 12.
- Suggests declaring the identifier or using a valid alternative.
By correcting the undeclared identifier, the program can compile and execute without this specific error. Remember that C requires explicit declarations and does not implicitly recognize boolean values such as `true` or `false`.](https://content.bartleby.com/qna-images/question/e7ddc10c-4670-40fd-b02c-6a60c5fcc2f2/2b81bc29-a744-428b-9c9c-8cdb62842a13/pnivz6d_thumbnail.png)
Transcribed Image Text:### C Programming: Basic Stack Operations
#### Source Code
The following code snippet demonstrates basic stack operations using a C program:
```c
#include<stdio.h>
#include<stdlib.h>
#define OFFSET 32
int Top=-1, inp_array[OFFSET];
void push();
void pop();
void print_stack();
int main() {
int choice;
while(true) {
printf("\nOperations performed by Stack");
printf("\n1.push the element\n2.pop the element\n3.Display Stack\n4.End");
printf("\nInput the choice:");
scanf("%d",&choice);
switch(choice) {
case 1: push();
break;
case 2: pop();
break;
case 3: print_stack();
break;
case 4: exit(0);
default: printf("\nInvalid choice!!!");
}
}
}
```
#### Explanation and Error
- **Header Files and Constants**: Includes the standard input-output and standard library header files. Defines a constant `OFFSET` with a value of 32.
- **Global Variables**: Declares an integer `Top` initialized to -1 and an integer array `inp_array` sized by `OFFSET`.
- **Function Declarations**:
- `push()`: To add elements to the stack.
- `pop()`: To remove elements from the stack.
- `print_stack()`: To display elements in the stack.
- **Main Function**:
- Uses a `while` loop to continually perform stack operations based on user input.
- Prompts the user for a choice and executes corresponding functions.
- **Error Notice**: The code contains an error due to the use of `true` without declaration. C does not inherently recognize `true`. To resolve this, replace `while(true)` with `while(1)` for infinite loop functionality.
#### Compiler Output
On the right, the panel shows the compiler output:
- An error indicating `'true' undeclared` in the main function at line 12.
- Suggests declaring the identifier or using a valid alternative.
By correcting the undeclared identifier, the program can compile and execute without this specific error. Remember that C requires explicit declarations and does not implicitly recognize boolean values such as `true` or `false`.
Solution
by Bartleby Expert
Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
There is an error on line 12. I need that error to be fixed. You can see that error on the image attached.
![### C Programming: Basic Stack Operations
#### Source Code
The following code snippet demonstrates basic stack operations using a C program:
```c
#include<stdio.h>
#include<stdlib.h>
#define OFFSET 32
int Top=-1, inp_array[OFFSET];
void push();
void pop();
void print_stack();
int main() {
int choice;
while(true) {
printf("\nOperations performed by Stack");
printf("\n1.push the element\n2.pop the element\n3.Display Stack\n4.End");
printf("\nInput the choice:");
scanf("%d",&choice);
switch(choice) {
case 1: push();
break;
case 2: pop();
break;
case 3: print_stack();
break;
case 4: exit(0);
default: printf("\nInvalid choice!!!");
}
}
}
```
#### Explanation and Error
- **Header Files and Constants**: Includes the standard input-output and standard library header files. Defines a constant `OFFSET` with a value of 32.
- **Global Variables**: Declares an integer `Top` initialized to -1 and an integer array `inp_array` sized by `OFFSET`.
- **Function Declarations**:
- `push()`: To add elements to the stack.
- `pop()`: To remove elements from the stack.
- `print_stack()`: To display elements in the stack.
- **Main Function**:
- Uses a `while` loop to continually perform stack operations based on user input.
- Prompts the user for a choice and executes corresponding functions.
- **Error Notice**: The code contains an error due to the use of `true` without declaration. C does not inherently recognize `true`. To resolve this, replace `while(true)` with `while(1)` for infinite loop functionality.
#### Compiler Output
On the right, the panel shows the compiler output:
- An error indicating `'true' undeclared` in the main function at line 12.
- Suggests declaring the identifier or using a valid alternative.
By correcting the undeclared identifier, the program can compile and execute without this specific error. Remember that C requires explicit declarations and does not implicitly recognize boolean values such as `true` or `false`.](https://content.bartleby.com/qna-images/question/e7ddc10c-4670-40fd-b02c-6a60c5fcc2f2/2b81bc29-a744-428b-9c9c-8cdb62842a13/pnivz6d_thumbnail.png)
Transcribed Image Text:### C Programming: Basic Stack Operations
#### Source Code
The following code snippet demonstrates basic stack operations using a C program:
```c
#include<stdio.h>
#include<stdlib.h>
#define OFFSET 32
int Top=-1, inp_array[OFFSET];
void push();
void pop();
void print_stack();
int main() {
int choice;
while(true) {
printf("\nOperations performed by Stack");
printf("\n1.push the element\n2.pop the element\n3.Display Stack\n4.End");
printf("\nInput the choice:");
scanf("%d",&choice);
switch(choice) {
case 1: push();
break;
case 2: pop();
break;
case 3: print_stack();
break;
case 4: exit(0);
default: printf("\nInvalid choice!!!");
}
}
}
```
#### Explanation and Error
- **Header Files and Constants**: Includes the standard input-output and standard library header files. Defines a constant `OFFSET` with a value of 32.
- **Global Variables**: Declares an integer `Top` initialized to -1 and an integer array `inp_array` sized by `OFFSET`.
- **Function Declarations**:
- `push()`: To add elements to the stack.
- `pop()`: To remove elements from the stack.
- `print_stack()`: To display elements in the stack.
- **Main Function**:
- Uses a `while` loop to continually perform stack operations based on user input.
- Prompts the user for a choice and executes corresponding functions.
- **Error Notice**: The code contains an error due to the use of `true` without declaration. C does not inherently recognize `true`. To resolve this, replace `while(true)` with `while(1)` for infinite loop functionality.
#### Compiler Output
On the right, the panel shows the compiler output:
- An error indicating `'true' undeclared` in the main function at line 12.
- Suggests declaring the identifier or using a valid alternative.
By correcting the undeclared identifier, the program can compile and execute without this specific error. Remember that C requires explicit declarations and does not implicitly recognize boolean values such as `true` or `false`.
Solution
by Bartleby Expert
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- In Visual Studio using Console App (.NET Core) (4) In Visual Studio, create and test a console application that does the following: (1) Create a variable of the Integer data type, named marks; (2) Create a variable of the String data type, named grade; (3) Use the built-in function Console.ReadLine() to get the user's input for marks as follows (it is assumed that the user always inputs integers when testing this program): Console.WriteLine("Input the marks:") marks CType(Console.ReadLine(), Integer) (4) Convert marks into grade as follows using an If-Then-Elself statement: marks 90-100 85-89 80-84 77-79 73-76 70-72 67-69 63-66 60-62 50-59 0-49 grade A+ A A- B+ B B- C+ с C- D F (5) Display the value of grade in the console window using the following statement: Console.WriteLine("The grade is "& grade) Hint: The If-Then-Elself statement is a lengthy one and is similar to the following: If ((marks >=90) And (marks = 85) And (marks =0) And (marks<-49) Then grade "F" Elsearrow_forwardAssignment Name JavaScript Concepts III Assignment Filename lastname-circleArea.html (replace lastname with your last name). area.js Assignment Description This assignment demonstrates the following concepts: JavaScript Functions Assignment Instructions Write a JavaScript program that calculates the area of a circle: Write a function that calculates the area of a circle given the radius. Formula: Math.PI * (radius * radius) Your program code should contain appropriately named variables. Use documentation to identify various components of your program. Call the function once and pass a radius value of 10.75 to it. Use the starter files and complete the logic to satisfy the instructions. To access the files, download and save the circleArea.zip folder attached to this assignment. Then unzip the compressed folder to reveal the included files: circleArea.html and area.js. Be sure that the two files are in the same folder. Then write your code in the area.js…arrow_forwardWrite in c language please: write a statement that calls the function IncreaseItemQty with parameters computerInfo and addStock. Assign computerInfo with the returned value. #include <stdio.h> typedef struct ProductInfo_struct { char itemName[50]; int itemQty;} ProductInfo; ProductInfo IncreaseItemQty(ProductInfo productToStock, int increaseValue) { productToStock.itemQty = productToStock.itemQty + increaseValue; return productToStock;} int main(void) { ProductInfo computerInfo; int addStock; scanf("%s", computerInfo.itemName); scanf("%d", &computerInfo.itemQty); scanf("%d", &addStock); /* Your code goes here */ printf("Name: %s, stock: %d\n", computerInfo.itemName, computerInfo.itemQty); return 0;} thank you in advancearrow_forward
- C++ formatting please Write a program (not a function) that reads from a file named "data.csv". This file consists of a list of countries and gold medals they have won in various Olympic events. Write to standard out, the top 5 countries with the most gold medals. You can assume there will be no ties. Expected Output: 1: United States with 1022 gold medals 2: Soviet Union with 440 gold medals 3: Germany with 275 gold medals 4: Great Britain with 263 gold medals 5: China with 227 gold medalsarrow_forwardin C code not c++arrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forward
- USING ARROW FUNCTIONS IN JAVASCRIPT WRITE THE FOLLOWING CODEarrow_forwardpython programing: You are going to create a race class, and inside that class it has a list of entrants to the race. You are going to create the daily schedule of three or so races and populate the races with the jockey/horse entries and then print out the schedule. Create a race class that has The name of the race as a character string, ie “RACE 1” or “RACE 2”, The time of the race A list of entries for that race Create a list of of 3 (or 4) of those race objects(races) for the day For the list of entries for a particular race, make a entrant class (the building block of your entrants list) you should have The horse name The jockey name Now you need to Populate the structure (ie set up three races on the schedule, add 2-3 horses entries to each race. You can hardcode the entries if you want to, rather than getting them from the user, just to save the time of making a menu interface and whatnot. Be able to print the days schedule So example output would be…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
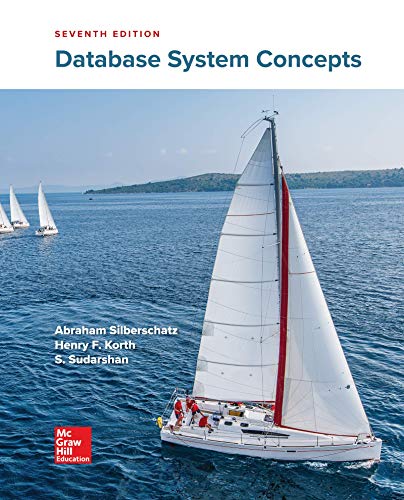
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
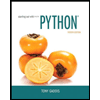
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
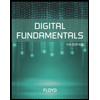
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
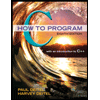
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
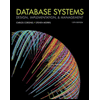
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
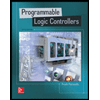
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education