// main module Module main() // Local variables Declare Integer number // Get number Call getNumber(number) // display Roman numeral Select number Case 1: Display “I” Case 2: Display “II” Case 3: Display “III” Case 4: Display “IV” Case 5: Display “V” Case 6: Display “VI” Case 7: Display “VII” Case 8: Display “VIII” Case 9: Display “IX” Case 10: Display “X” Default: Display “Error: Invalid Number” End Select End Module // The getNumber module gets wall space and stores it // in the number reference variable. Module getNumber (Integer Ref number) Display “Enter an integer from 1 to 10: ” Input number End Module I want flowchart and pseudocode please
// main module
Module main()
// Local variables
Declare Integer number
// Get number
Call getNumber(number)
// display Roman numeral
Select number
Case 1:
Display “I”
Case 2:
Display “II”
Case 3:
Display “III”
Case 4:
Display “IV”
Case 5:
Display “V”
Case 6:
Display “VI”
Case 7:
Display “VII”
Case 8:
Display “VIII”
Case 9:
Display “IX”
Case 10:
Display “X”
Default:
Display “Error: Invalid Number”
End Select
End Module
// The getNumber module gets wall space and stores it
// in the number reference variable.
Module getNumber (Integer Ref number)
Display “Enter an integer from 1 to 10: ”
Input number
End Module
I want flowchart and pseudocode please

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

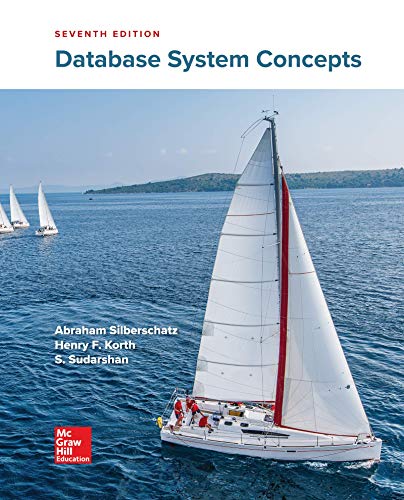
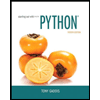
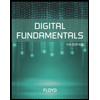
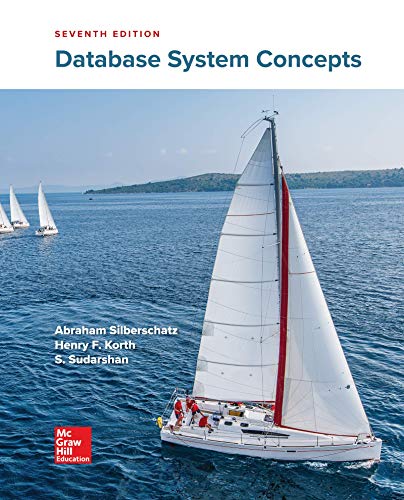
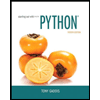
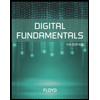
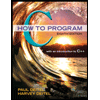
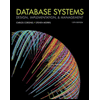
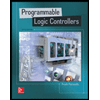