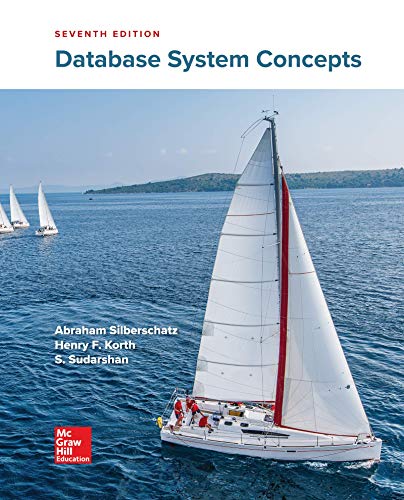
Write a program that takes any word, like SUSHI, and builds a binary search tree in alphabetical order,
allowing duplicate letters, which will be inserted to the left when the duplicate is encountered. Then output
the string in a vertical tree-like form. Input will be a one-word string (all caps) greater than or equal to 1
letter(s) long. Assume proper input. The vertical tree should consist of a line of nodes, followed by a line of
/ and \ characters indicating relationships, followed by a line of nodes, etc. Assume all nodes are
representable as a single character. Adjacent nodes on the lowest level should be separated by at least one
space, nodes further up should be separated as appropriate. Nodes with two children should be placed
precisely in the middle of their direct children. Relationship slashes should be halfway between the parent
and the appropriate child (round whichever way you want). The user should be able to visualize the levels
lining up with each other. In C++, use the C++ string class for input. A tree data structure must be used.
Refer to the sample output below.
Sample Runs:
Enter a word: JAMES
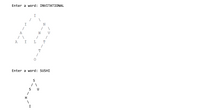
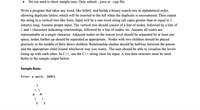

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Decode Binary String Assignment Using the Huffman tree depicted below, decode the following binary string. Use alternate colors to represent the individual letters and corresponding sub-string, separate sub-string by a blank space. You can do it by hand, scan, and upload. For example, 100010 1001 010 g o e 000001001110010100001000011101010001011100111010100011001111101111001arrow_forwardWrite a method isBST() that takes a Node as argument and returns true if the argument node is the root of a binary search tree, false otherwise.Hint : This task is also more difficult than it might seem, because the order in which youcall the methods in the previous three exercises is importantarrow_forwardIn the binary search tree, write a function that takes in a root, p, and checks whether the tree rooted in p is a binary search tree or not. What is the time complexity of your function? def is_bst(self, p: Node):arrow_forward
- Rewrite the definition of the function searchNode of the class B-tree provided (bTree.h) by using a binary search. Write a C++ code to ask the user to enter a list of positive integers ending with -999, build a b- tree of order 5 using the positive integers, and display the tree contents. Also, ask the user to enter a number to search and display if the number is found in the tree. bTree.harrow_forwardWrite a method isBST() that takes a Node as argument and returns true if the argument node is the root of a binary search tree, false otherwise.Hint : This task is also more difficult than it might seem, because the order in which youcall the methods in the previous three exercises is importantarrow_forwardYou have an empty binary search tree. After that, you do the following sequence of insertions:20, 30, 10, 50, 40, 60. Draw the status of the binary search tree after the first 3 insertions, and at the endof all 6 insertions (you only need to show 2 trees).arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
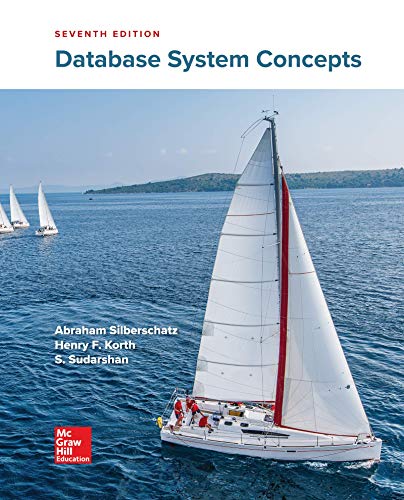
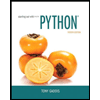
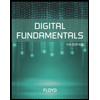
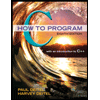
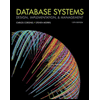
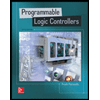