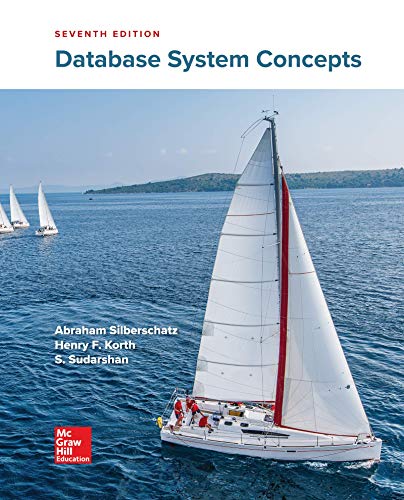
Concept explainers
Laboratory Exercise Understanding
Objective:
At the end of the exercise, the students should be able to:
▪ Construct a Python script based on the given algorithms.
Software Requirement:
▪ Python 3.7 or higher
Procedure:
1. Study the following sample Python syntaxes:
Task Sample Syntax Remarks Variable declaration x = 5 No need to indicate the data type. Do not add semi-colon. Comment #This is a comment. User input name = input(“Enter your name”) Convert string to int or float. int(x) float(x) Convert to compare or compute values. Convert int or float to string. str(x) Convert if the output must show a number and a string. Combine conditional statements. x > 5 and x <10 x > 5 or x < 10 not(x < 5) if statement if x == 0: print(“Enter a higher number”) Use four (4) spaces per indentation level. else-if statement elif x == 0: print(“Enter a higher number”) else statement else: print(“Try again”) Create a list of items. my_list = [“red”, “blue”, “green”] Python recommends the use of lists than arrays. Display all items in a list. print(my_list) Create an empty list. my_list = [] Add an item to the end of a list. my_list.append(“yellow”)
2. Open Notepad++.
3. Create a Python script that will compare two (2) numbers entered by the user. Refer to the sample syntaxes and the algorithm below. 3.1. User enters the first number. 3.2. User enters the second number. 3.3. If the first number is less than, greater than, or equal the second number, a message is displayed.
4. Save the script as algo1.py.
5. To test and run the script, open Command Prompt. Navigate to your file’s location then type python algo1.py. IT1815 01 Laboratory Exercise 1 *Property of STI Page 2 of 2
6. Create another Python script that will display the names of your three (3) classmates. Refer to the sample syntaxes and algorithm below. 6.1. User enters the name of the first classmate. 6.2. User enters the name of the second classmate. 6.3. User enters the name of the third classmate. 6.4. The name of the three (3) classmates are displayed.
7. Save the script as algo2.py.
8. Create a folder named LastName_FirstName_MI_LE1 (ex. Reyes_Nika_P_LE1) in your local drive.
9. Move the two (2) scripts to your folder.

Step by stepSolved in 2 steps with 2 images

- Course Level Programming Assignment - Programming a Calculator using Python In this assignment you will write a computer program from scratch using the Python programming language. This program will function as a simple calculator. Objectives Write a simple Python program that performs arithmetic operations based on the user input Stage 1: A simple calculator Your calculator should provide the following arithmetic and control operations. Arithmetic Operations Addition (+) add(a,b) Subtraction (-) subtract(a,b) Multiplication (*) multiply(a,b) Division (/) divide(a,b) Power (^) power(a,b) Remainder (%) remainder(a,b) Control Operations Terminate (#) Reset ($) Write a function select_op(choice) to select the appropriate mathematics function based on the users selection. The behavior of the program should be as follows: The program should ask the user to specify the desired operation…arrow_forwardC Language thanksarrow_forwardC PROGRAM / C LANGUAGE Make a C program language. Input the number of players then input the number of goals scored for each. Print "Not Messi" if the highest goals exceed 10 and "Okay, fine, it's Messi" if it doesn't INPUT 1. Number of players 2. Goals scored by each player Constraints All of those goals are whole numbers The first line will contain a message prompt to input the number of players • The succeeding lines will contain a message to input the goals scored by each player • The last line contains appropriate resulting message EXPECTED OUTPUT A EXPECTED OUTPUT B Enter the number of players: 4 Goals score by player # 1: 7 Goals score by player #2: 2 Goals score by player #3: 5 Goals score by player #4: 1 Okay, fine it's Messi Enter the number of players: 5 Goals score by player # 1: 10 Goals score by player #2: 2 Goals score by player #3: 20 Goals score by player # 4: 1 Goals score by player # 4: 0 Not Messiarrow_forward
- Although Algol60's flexible style permits statements to start and stop anywhere, the majority of programming languages today demand that statements explicitly end with an end symbol, such as a semicolon or a colon. In contrast, Python and a select few other programming languages have a predefined structure whereby statements start in a certain column and conclude at the end of a line of code unless continuation marks are included for each statement. You will discover how fixed or free formats affect readability, writability, and security in this section.arrow_forwardWhat are the stages in building a program function?arrow_forward"Programming style" conjures up images of what? How do you know whether you've done your job well as a programmer?arrow_forward
- Although Algol60's flexible style permits statements to start and stop anywhere, the majority of programming languages today demand that statements explicitly end with an end symbol, such as a semicolon or a colon. In contrast, Python and a select few other programming languages have a predefined structure whereby statements start in a certain column and conclude at the end of a line of code unless continuation marks are included for each statement. You will discover how fixed or free formats affect readability, writability, and security in this section.arrow_forwardAssignment Name JavaScript Concepts III Assignment Filename lastname-circleArea.html (replace lastname with your last name). area.js Assignment Description This assignment demonstrates the following concepts: JavaScript Functions Assignment Instructions Write a JavaScript program that calculates the area of a circle: Write a function that calculates the area of a circle given the radius. Formula: Math.PI * (radius * radius) Your program code should contain appropriately named variables. Use documentation to identify various components of your program. Call the function once and pass a radius value of 10.75 to it. Use the starter files and complete the logic to satisfy the instructions. To access the files, download and save the circleArea.zip folder attached to this assignment. Then unzip the compressed folder to reveal the included files: circleArea.html and area.js. Be sure that the two files are in the same folder. Then write your code in the area.js…arrow_forwardProblem Solving Technique Also known as Software Development Methodology. Involved six essential steps in program development. Helps in producing the output needed within the given timeline. The first three steps are very critical in problem development. PROBLEM You are a System Developer in a hospital. You are to help the front desk in developing a program that reads and displays the age of 20 people (one after another) in sequence. Hint: You need a way to count how many people whose age have been read and displayed. Therefore, you can used a concept of counter, a variable used to count the number of people whose age have been processed by the program. From the problem below, Analyse STEP 1 until STEP 3. Follow the Problem above. For STEP 3 Please write both Flow Chart and Pseudo Code. STEP 1: Specify the problem requirement STEP 2: Analyze the problem STEP 3: Design the algorithm to solve the problem Flowchart Pseudo Codearrow_forward
- A(n) on its own but can be used with other software products. _is a small application that cannot runarrow_forwardC Programming Language Assignment #4Introduction to C Programming – COP 3223Objectives1. To learn how to use loops for repeated execution2. To reinforce knowledge of If-Else statements for conditional executionIntroduction: Programmers for a Better TomorrowProgrammers for a Better Tomorrow is an organization dedicated to helping charities, medical societies, and scholarship organizations manage various tasks so that they can focus on making the world a better place! They have asked you and your classmates to help them develop some new programs to benefit their organizations.Problem: Scholarship Endowment Fund Part 2 (fund2.c)One division of Programmers for a Better Tomorrow is their Scholarship Endowment Fund. They provide yearly scholarships to students who need a hand in amounts of 1000, 500, and 250 dollars.The money for these scholarships comes from interest made on previous donations and investments. You will create a program to track the amount in the Fund as various donations…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
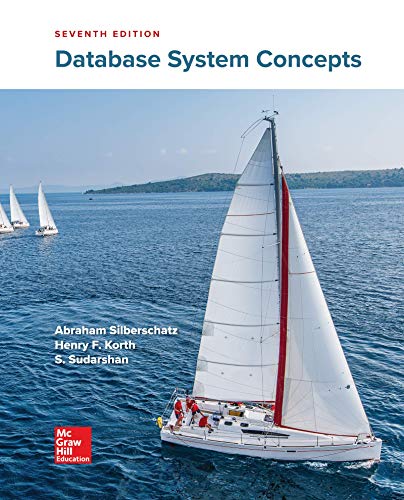
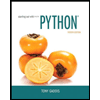
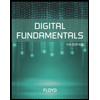
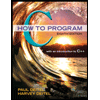
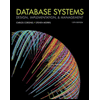
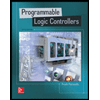