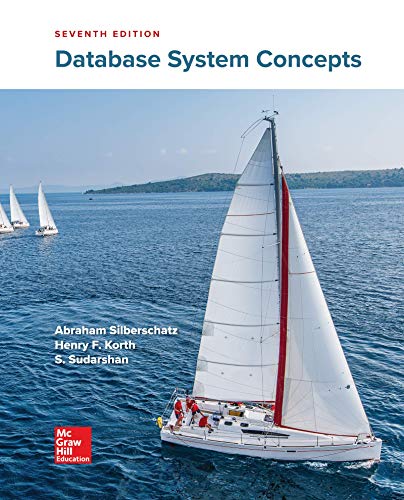
Concept explainers
Implement in C
7.11.1: LAB: Student struct
Given main(), build a struct called Student that represents a student that has two data members: the student's name (string) and the student's GPA (double). Assume student's name has a maximum length of 20 characters.
Implement the Student struct and related function declarations in Student.h, and implement the related function definitions in Student.c as listed below:
- Student InitStudent() - initializes name to "Louie" and gpa to 1.0
- Student SetName(char *name, Student s) - sets the student's name
- Student SetGPA(double gpa, Student s) - sets the student's GPA
- void GetName(char* studentName, Student s) - return the student's name in studentName
- double GetGPA(Student s) - returns the students GPA
Ex. If a new Student object is created, the default output is:
Louie/1.0
Ex. If the student's name is set to "Felix" and the GPA is set to 3.7, the output becomes:
Felix/3.7
main.c
#include <stdio.h>
#include <string.h>
#include "Student.h"
/* Type your code here */
Student.h
#ifndef STUDENT_H_
#define STUDENT_H_
/* Type your code here */
#endif

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Develop an algorithm and write a C++ program that counts the letter occurrence in the string; make sure you use call by reference to the array of a class with letter and count as private member attributes when return from parse function. For the class member methods, provide the public accessor and modifier as well as print functions for the private member attributes. Write a test program that reads a C-string and displays the number of letters [a-z] and number of numbers [0-9] in the string. In addition to that, you need to output the histogram of the letter and count array. Here is a sample run of the program: <Output> Enter a string: 2023 is coming The number of letters in 2023 is coming is 8 The number of numbers in 2023 is coming is 4 ---- Histogram ---- Char Count 0 1 2 2 3 1 c 1 g 1 i 2 m 1 n 1 /* not a completed output */ <End Output>arrow_forwardWrite a structure called Point that has two member integer variables called x and y. Write a function that accepts a point and displays it in the form (x, y). For example if the x coordinate is 5 and y coordinate is 7, it should display the point as (5, 7) on the monitor. Write a function called readPointValues that gets the address of a point and use pointer notations to read values x and y into the point. Write a function to find the distance between two points. Write the main program to test all these functions. C languagearrow_forwardThis is in c++. Create a class of function objects called StartsWith that satisfies the following specification: when initialized with character c, an object of this class behaves as a unary predicate that determines if its string argument starts with c. For example, StartsWith(’a’) is a function object that can be used as a unary predicate to determine if a string starts with an a. So, StartsWith(’a’)("alice") would return true but StartsWith(’a’)("bob") would return false. The function objects should return false when called on an empty string. Test your function objects by using one of them together with the STL algorithm count_if to count the number of strings that start with some letter in some vector of strings. So basically, I want a class that returns true or false based on if the name that is typed starts with the character that I put down.arrow_forward
- Implement in C Programing 7.10.1: LAB: Product struct Given main(), build a struct called Product that will manage product inventory. Product struct has three data members: a product code (string), the product's price (double), and the number count of product in inventory (int). Assume product code has a maximum length of 20. Implement the Product struct and related function declarations in Product.h, and implement the related function definitions in Product.c as listed below: Product InitProduct(char *code, double price, int count) - set the data members using the three parameters Product SetCode(char *code, Product product) - set the product code (i.e. SKU234) to parameter code void GetCode(char *productCode, Product product) - return the product code in productCode Product SetPrice(double price, Product product) - set the price to parameter product double GetPrice(Product product) - return the price Product SetCount(int count, Product product) - set the number of items in inventory…arrow_forwardCode in c++arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
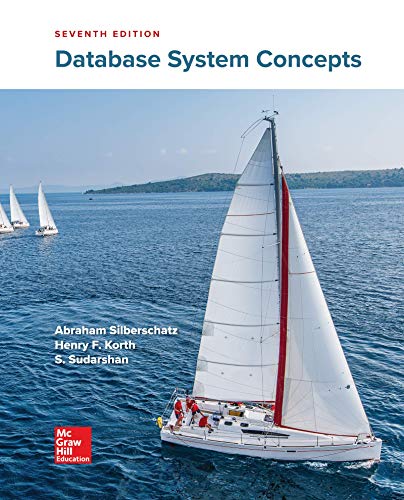
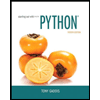
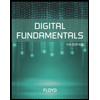
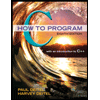
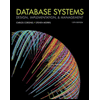
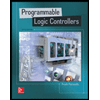