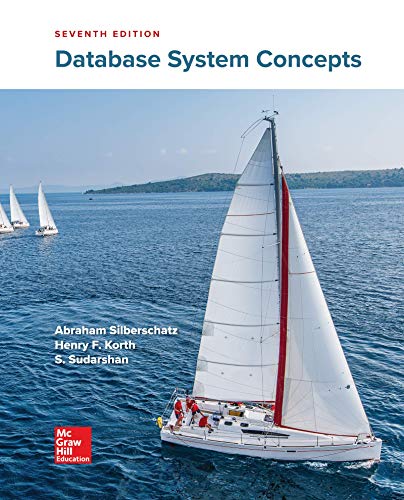
Concept explainers
Lab 10 Using an interface to share methods
It is often the case that two or more classes share a common set of methods. For programming purposes we might wish to treat the objects of those classes in a similar way by invoking some of their common routines.
For example, the Dog and Cat classes listed below agree on the void method speak. Because Dog and Cat objects have the ability to “speak,” it is natural to think of putting both types of objects in an ArrayList and invoking speak on every object in the list. Is this possible? Certainly we could create an ArrayList of Dog that would hold all the Dog objects, but can we then add a Cat object to an ArrayList of Dog?
Try running the main program below as it is written. Run it a second time after uncommenting the line that instantiates a Cat object and tries to add it to the ArrayList.
import java.util.*;
public class AnimalRunner
{
public static void main(String[] args)
{
ArrayList<Dog> dogcatList = new ArrayList<Dog>();
dogcatList.add(new Dog("Fred"));
// dogcatList.add(new Cat("Wanda"));
}
}
-------------------
public class Dog
{
private String name;
public Dog(String name)
{
this.name = name;
}
public void speak()
{
System.out.println("Woof! Woof!");
}
public String toString()
{
return "Dog: " + name;
}
}
-------------------
public class Cat
{
private String name;
public Cat(String name)
{
this.name = name;
}
public void speak()
{
System.out.println("Meow! Meow!");
}
public String toString()
{
return "Cat: " + name;
}
}
Our experiment to add Cat objects to an ArrayList of Dog objects failed. Perhaps we should try using the original Java ArrayList without generics? Try running the code below as it is written along with the Dog andCat classes defined above. Run it a second time after uncommenting the line that invokes speak.
import java.util.*;
public class AnimalRunner
{
public static void main(String[] args)
{
ArrayList dogcatList = new ArrayList();
dogcatList.add(new Dog("Fred"));
// dogList.add(new Cat("Wanda"));
for (Object obj : dogcatList)
{
// obj.speak();
}
}
}
The experiment shows that we are now able to add Dog and Cat objects to the ArrayList, but there is a compile error on the line obj.speak because obj is an Object reference variable and the class Object doesn’t contain a speak method. We need a reference variable that can refer to Dog and Cat objects and which also allows us to invoke speak. The solution to the problem uses interfaces.
First create an interface called Speakable that specifies a void speak() method. Be sure to modify the Dog and Cat classes to indicate that they implement the Speakable interface. For example, in the case of theDog class, we will code public class Dog implements Speakable. Be sure to make a similar change in the declaration of the Cat class.
The term Speakable can be used to create Speakable references. Using generics, create an ArrayList of Speakable objects in the main method. Modify the for loop so that it iterates over Speakable objects. Try adding the Dog and Cat objects and invoking the speak method on each object. Does this work?

Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 5 images

- Assume the existence of a Widget class that implements the Comparable interface and thus has a compareTo method that accepts an Object parameter and returns an int. Write an efficient static method, getWidgetMatch, that has two parameters. The first parameter is a reference to a Widget object. The second parameter is a potentially very large array of Widget objects that has been sorted in ascending order based on the Widget compareTo method. The getWidgetMatch searches for an element in the array that matches the first parameter on the basis of the equals method and returns true if found and false otherwise.arrow_forwardConsider the following class hierarchy: class Animal { ... } class Mammal extends Animal { ... } class Cat extends Mammal { ... } Write a generic method printArray that takes an array of any type and prints each element of the array. If the element is an instance of Animal or its subclass, the method should call the toString method of the object to print its details. Otherwise, the method should simply print the element. Your method should have the following signature: public static <T> void printArray(T[] array)arrow_forwardNeed help on java homework assignment, It says Create a class Vehicle. A Vehicle has a single instance variable of type String named "vehicleType" that describes the vehicle. It has an abstract method getTires that takes no parameters and returns an ArrayList<Tire> object that describes each Tire of the vehicle. A Tire class has been provided. Vehicle has a single constructor that takes the vehicle type as a parameter.Create a class Bicycle that extends Vehicle that has two Tires, both of type "skinny". Bicycle should have a single default constructor.Create a class Dragster that extends Vehicle and has four Tires, two of type "slick" and two of type "medium". Dragster should have a single default constructor.Write a tester program to test Bicycle and Dragster objects. Use declarations such as Vehicle b = new Bicycle();in your tester. Here is the code provided : public class Tire { private String size; public Tire(String siz) { size = siz; } public String…arrow_forward
- The following is the specification for the constructor of a EmailFolder class: /*** Creates a new EmailFolder with the given label** @precondition label != null AND !label.isEmpty()* @postcondition getLabel()==label*/public EmailFolder(String label) Assume the variable folders is an array list of EmailFolder objects. Write code to add a new EmailFolder to the list.arrow_forwardWrite a C#program that uses a class called ClassRegistration as outlined below: The ClassRegistration class is responsible for keeping track of the student id numbers for students that register for a particular class. Each class has a maximum number of students that it can accommodate. Responsibilities of the ClassRegistration class: It is responsible for storing the student id numbers for a particular class (in an array of integers) It is responsible for adding new student id numbers to this list (returns boolean) It is responsible for checking if a student id is in the list (returns a boolean) It is responsible for getting a list of all students in the class (returns a string). It is responsible for returning the number of students registered for the class (returns an integer) It is responsible for returning the maximum number of students the class is allowed (returns an integer) It is responsible for returning the name of the class. (returns a string) ClassRegistration -…arrow_forwardComplete the Course class by implementing the courseSize() method, which returns the total number of students in the course. Given classes: Class LabProgram contains the main method for testing the program. Class Course represents a course, which contains an ArrayList of Student objects as a course roster. (Type your code in here.) Class Student represents a classroom student, which has three fields: first name, last name, and GPA. Ex. For the following students: Henry Bendel 3.6 Johnny Min 2.9 the output is: Course size: 2 public class LabProgram { public static void main (String [] args) { Course course = new Course(); // Example students for testing course.addStudent(new Student("Henry", "Bendel", 3.6)); course.addStudent(new Student("Johnny", "Min", 2.9)); System.out.println("Course size: " + course.courseSize()); } } public class Student { private String first; // first name private String last; // last name private…arrow_forward
- Use this exercise to test your code that you wrote in the previous exercise. CookBook Class In this exercise, you are going to complete the CookBook class. public class CookBook { private ArrayList<Recipe> recipes; private String name; public CookBook(String name){ this.name = name; recipes = new ArrayList<Recipe>(); } /** * This method adds a recipe to the ArrayList of Recipes * @param recipe a recipe object to be added to the list */ public void addRecipe(Recipe recipe){ /* Implement this method */ } /** * This method removes all recipes from the * ArrayList of Recipes where the title matches the input * string * * @param title the title of the recipe to remove * @return the number of recipes removed */ public int removeRecipe(String title){ /* Implement this method */ } /** * This method lists the title of all the recipes in the * cook book * */ public void listAll(){ /* Implement this method */ } } The addRecipe method should take a recipe and add it to the end of the…arrow_forwardWrite a method for the farmer class that allows a farmer object to pet all cows on the farm. Do not use arrays.arrow_forwardUSING JAVA: Part A) Implement a superclass Appointment and subclasses Onetime, Daily, and Monthly. An Appointment has a description (for example, “See the dentist”) and a date. Write a method, occuresOn(int year, int month, int day) The check whether the appointment occurs on that date. For example, for appointment, you must check whether the day of the month matches. This fill an array of Appointment objects with a mixture of appointments. Have the user enter a date and print out all appointments that occur on that date. Part B) Improve the appointment book by giving the user the option to add new appointments. The user must specify the type of the appointment, the description, and the date.arrow_forward
- Q1: Write the Java code corresponding to the following UML diagram. The class School implements the interface Building. The method computeArea returns the area of the building as width * length. After that, add a testing class with a main method which defines an arrayList that contains three objects. From the main method, print the area of all the three objects. Building > + computeArea() : double + toString() : String School classroomNum: int width: double - length: double + School(classroomNum: int, width: double, length: double) + computeArea() : double + toString() : Stringarrow_forwardhow would you do this? this is a non graded practice labarrow_forwardInheritance, Polymorphism, ArrayLists, Throwing Exceptions The UML diagram below shows a set of classes designed to represent a music collection from 1995. The constructors and methods all function in the standard way, except: The equipmentRequired method should return “Record Player” or “CD Player” as appropriate.The getAlbum method of the NinetiesMusicCollection class accepts an index and returns the corresponding Album object. This method throws an IllegalArgumentException if the index is out of range. (This is the only exception you have to throw anywhere in your code.)In the NinetiesMusicCollection constructor, you can assume the ArrayList<Album> object passed as an argument is not null . Don’t worry about privacy leaks.Implement this set of classes in Java. Note the italics on the Class name “Album” and the method name “equipmentRequired” in the Album class.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
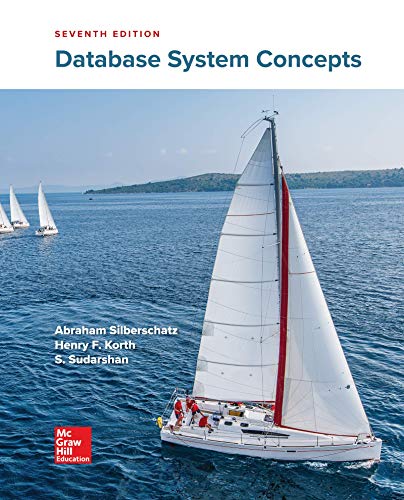
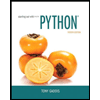
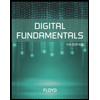
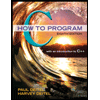
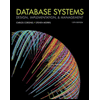
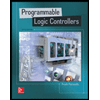