**Transcription and Explanation for Educational Website** --- **Title: Rotating Colored Cube** **URL:** `File: C:/Users/haile/hello/cube%201/index.html` **Content Description:** This webpage appears to be a local HTML file aimed at displaying a rotating colored cube. The title suggests an interactive 3D graphic element. **Visual Content:** - There is a large rectangular space outlined within the browser window. Although it is currently empty or not displaying content, this area is likely intended for the visual representation of the cube. **Functional Overview:** The webpage, when functioning correctly, is intended to demonstrate: 1. **3D Animation**: The rotating action implies the use of 3D graphics, giving viewers insights into basic animations using HTML/CSS or JavaScript. 2. **Color Dynamics**: The mention of a "Colored Cube" implies that the cube is likely to exhibit various colors, possibly changing or transitioning during rotation. **Educational Objective:** This demonstration aims to teach foundational concepts of web-based 3D graphics and interactivity, providing a practical understanding of how to implement and visualize rotating 3D objects on a webpage. --- **Note**: If you're not seeing the rotating cube in action, ensure the HTML file is correctly coded and all necessary scripts or assets are properly linked.
**Transcription and Explanation for Educational Website** --- **Title: Rotating Colored Cube** **URL:** `File: C:/Users/haile/hello/cube%201/index.html` **Content Description:** This webpage appears to be a local HTML file aimed at displaying a rotating colored cube. The title suggests an interactive 3D graphic element. **Visual Content:** - There is a large rectangular space outlined within the browser window. Although it is currently empty or not displaying content, this area is likely intended for the visual representation of the cube. **Functional Overview:** The webpage, when functioning correctly, is intended to demonstrate: 1. **3D Animation**: The rotating action implies the use of 3D graphics, giving viewers insights into basic animations using HTML/CSS or JavaScript. 2. **Color Dynamics**: The mention of a "Colored Cube" implies that the cube is likely to exhibit various colors, possibly changing or transitioning during rotation. **Educational Objective:** This demonstration aims to teach foundational concepts of web-based 3D graphics and interactivity, providing a practical understanding of how to implement and visualize rotating 3D objects on a webpage. --- **Note**: If you're not seeing the rotating cube in action, ensure the HTML file is correctly coded and all necessary scripts or assets are properly linked.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The below code is suppose to make a rotating colored 3d cube using html (canvas) and JavaScript but I cannot get it to work properly. What am I doing wrong? What is the problem? This is a WebGL project if that helps. All that is rendered is what is seen in the attatched image. Thank you for your help.
Source code HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Rotating Colored Cube</title>
<script type="text/javascript" src="glMatrix-1.0.1.min.js">
</script>
<script type="text/javascript" src="WebGL.js">
</script>
</head>
<body>
<canvas id="myCanvas" width="600" height="250"
style="border:1px solid black;">
</canvas>
</body>
</html>
JavaScript source code:
function initBuffers(gl){
var cubeBuffers = {}
cubeBuffers.positionBuffer = gl.createArrayBuffer([
// Front face
-1, -1, 1,
1, -1, 1,
1, 1, 1,
-1, 1, 1,
// Back face
-1, -1, -1,
-1, 1, -1,
1, 1, -1,
1, -1, -1,
// Top face
-1, 1, -1,
-1, 1, 1,
1, 1, 1,
1, 1, -1,
// Bottom face
-1, -1, -1,
1, -1, -1,
1, -1, 1,
-1, -1, 1,
// Right face
1, -1, -1,
1, 1, -1,
1, 1, 1,
1, -1, 1,
// Left face
-1, -1, -1,
-1, -1, 1,
-1, 1, 1,
-1, 1, -1
]);
// build color Vertices
var colors = [
[1, 0, 1, 1], // Front face - Pink
[0, 1, 0, 1], // Back face - Green
[0, 0, 1, 1], // Top face - Blue
[0, 1, 1, 1], // Bottom face - Turquoise
[1, 1, 0, 1], // Right face - Yellow
[1, 0, 0, 1] // Left face - Red
];
var colorVertices = [];
for (var n in colors) {
var color = colors[n];
for (var i=0; i < 4; i++) {
colorVertices = colorVertices.concat(color);
}
}
cubeBuffers.colorBuffer = gl.createArrayBuffer(colorVertices);
cubeBuffers.indexBuffer = gl.createElementArrayBuffer([
0, 1, 2, 0, 2, 3, // Front face
4, 5, 6, 4, 6, 7, // Back face
8, 9, 10, 8, 10, 11, // Top face
12, 13, 14, 12, 14, 15, // Bottom face
16, 17, 18, 16, 18, 19, // Right face
20, 21, 22, 20, 22, 23 // Left face
]);
return cubeBuffers;
}
function stage(gl, cubeBuffers, angle){
// set field of view at 45 degrees
// set viewing range between 0.1 and 100.0 units away.
gl.perspective(45, 0.1, 100);
gl.identity();
// translate model-view matrix
gl.translate(0, 0, -5);
// rotate model-view matrix about x-axis (tilt box downwards)
gl.rotate(Math.PI * 0.15, 1, 0, 0);
// rotate model-view matrix about y-axis
gl.rotate(angle, 0, 1, 0);
gl.pushPositionBuffer(cubeBuffers);
gl.pushColorBuffer(cubeBuffers);
gl.pushIndexBuffer(cubeBuffers);
gl.drawElements(cubeBuffers);
}
window.onload = function(){
var gl = new WebGL("myCanvas", "experimental-webgl");
gl.setShaderProgram("VARYING_COLOR");
var cubeBuffers = initBuffers(gl);
var angle = 0;
gl.setStage(function(){
// update angle
var angularVelocity = Math.PI / 4; // radians / second
var angleEachFrame = angularVelocity * this.getTimeInterval() / 1000;
angle += angleEachFrame;
this.clear();
stage(this, cubeBuffers, angle);
});
gl.start();
};

Transcribed Image Text:**Transcription and Explanation for Educational Website**
---
**Title: Rotating Colored Cube**
**URL:**
`File: C:/Users/haile/hello/cube%201/index.html`
**Content Description:**
This webpage appears to be a local HTML file aimed at displaying a rotating colored cube. The title suggests an interactive 3D graphic element.
**Visual Content:**
- There is a large rectangular space outlined within the browser window. Although it is currently empty or not displaying content, this area is likely intended for the visual representation of the cube.
**Functional Overview:**
The webpage, when functioning correctly, is intended to demonstrate:
1. **3D Animation**: The rotating action implies the use of 3D graphics, giving viewers insights into basic animations using HTML/CSS or JavaScript.
2. **Color Dynamics**: The mention of a "Colored Cube" implies that the cube is likely to exhibit various colors, possibly changing or transitioning during rotation.
**Educational Objective:**
This demonstration aims to teach foundational concepts of web-based 3D graphics and interactivity, providing a practical understanding of how to implement and visualize rotating 3D objects on a webpage.
---
**Note**: If you're not seeing the rotating cube in action, ensure the HTML file is correctly coded and all necessary scripts or assets are properly linked.
AI-Generated Solution
Unlock instant AI solutions
Tap the button
to generate a solution
Recommended textbooks for you
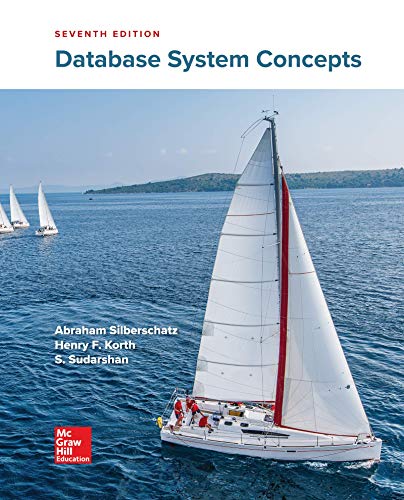
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
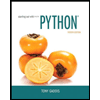
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
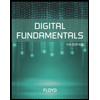
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
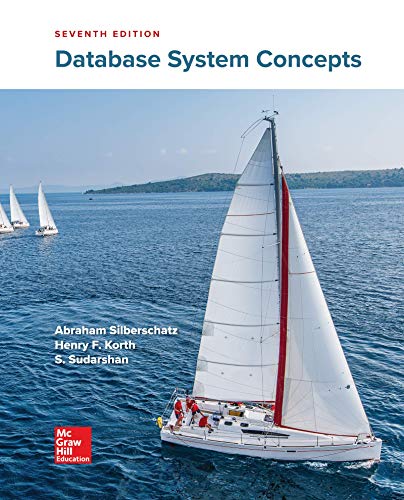
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
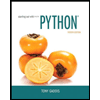
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
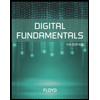
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
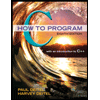
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
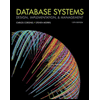
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
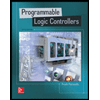
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education