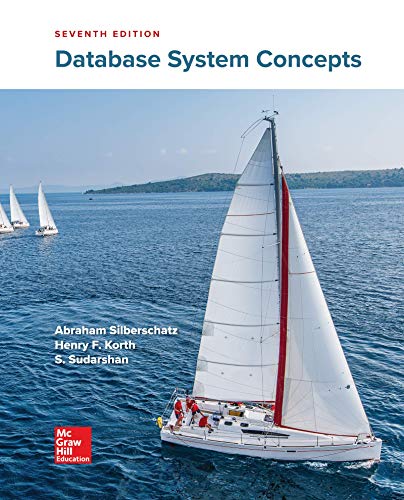
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
thumb_up100%
// JumpinJava.cpp - This program looks up and prints the names and prices of coffee orders.
// Input: Interactive
// Output: Name and price of coffee orders or error message if add-in is not found
#include <iostream>
#include <string>
using namespace std;
int main()
{
// Declare variables.
string addIn; // Add-in ordered
const int NUM_ITEMS = 5; // Named constant
// Initialized array of add-ins
string addIns[] = {"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"};
// Initialized array of add-in prices
double addInPrices[] = {.89, .25, .59, 1.50, 1.75};
bool foundIt = false; // Flag variable
int x; // Loop control variable
double orderTotal = 2.00; // All orders start with a 2.00 charge
// Get user input
cout << "Enter coffee add-in or XXX to quit: ";
cin >> addIn;
// Write the rest of the program here.
return 0;
} // End of main()
![Parallel Arrays
JumpinJive.cpp
Co a n you wilele to wite your ɔlateiiemLS.
1 // JumpinJava.cpp
21/ Input:
3 // Output: Name and price of coffee orders or error message if add-in is no
This program looks up and prints the names and prices a
dy Tools
Interactive
Instructions
4.
5 #include <iostream>
1. Study the prewritten code to make sure you
6 #include <string>
understand it.
7 using namespace std;
5 Tips
8.
2. Write the code that searches the array for the name
9 int mainO
Tips
of the add-in ordered by the customer.
10 {
11
// Declare variables.
3. Write the code that prints the name and price of the
12
string addIn;
const int NUM ITEMS = 5; // Named constant
no.
W Add-in ordered
add-in or the error message, and then write the
13
code that prints the cost of the total order.
14
// Initialized array of add-ins
string addIns[]
V/ Initialized array of add-in prices
15
{"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"};
4. Execute the program by clicking the Run button at
16
17
double addInPrices[]
bool foundIt = false;
the bottom of the screen. Use the following data:
= {,89, .25, .59, 1.50, 1.75};
18
// Flag variable
// Loop control variable
boxes
Cream
19
int X;
20
double orderTotal
2.00; // All orders start with a 2.00 charge
21
Caramel
22
// Get user input
Whiskey
23
cout << "Enter coffee add-in or XXX to quit: ";
ck
24
cin >> addIn;
chocolate
25
26
// Write the rest of the program here.
Chocolate
27
return O;
28 }// End of main()
Cinnamon
29
Vanilla
!!](https://content.bartleby.com/qna-images/question/4bfbb203-4399-4804-a6b8-bbfebafe4401/04d9555a-c5d5-434a-99f6-c277fd50bbca/ifypi35_thumbnail.jpeg)
Transcribed Image Text:Parallel Arrays
JumpinJive.cpp
Co a n you wilele to wite your ɔlateiiemLS.
1 // JumpinJava.cpp
21/ Input:
3 // Output: Name and price of coffee orders or error message if add-in is no
This program looks up and prints the names and prices a
dy Tools
Interactive
Instructions
4.
5 #include <iostream>
1. Study the prewritten code to make sure you
6 #include <string>
understand it.
7 using namespace std;
5 Tips
8.
2. Write the code that searches the array for the name
9 int mainO
Tips
of the add-in ordered by the customer.
10 {
11
// Declare variables.
3. Write the code that prints the name and price of the
12
string addIn;
const int NUM ITEMS = 5; // Named constant
no.
W Add-in ordered
add-in or the error message, and then write the
13
code that prints the cost of the total order.
14
// Initialized array of add-ins
string addIns[]
V/ Initialized array of add-in prices
15
{"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"};
4. Execute the program by clicking the Run button at
16
17
double addInPrices[]
bool foundIt = false;
the bottom of the screen. Use the following data:
= {,89, .25, .59, 1.50, 1.75};
18
// Flag variable
// Loop control variable
boxes
Cream
19
int X;
20
double orderTotal
2.00; // All orders start with a 2.00 charge
21
Caramel
22
// Get user input
Whiskey
23
cout << "Enter coffee add-in or XXX to quit: ";
ck
24
cin >> addIn;
chocolate
25
26
// Write the rest of the program here.
Chocolate
27
return O;
28 }// End of main()
Cinnamon
29
Vanilla
!!
![ays
JumpinJive.cpP
1 // JumpinJava.cpp - This program looks up and prints the n
Summary
2 // Input:
Interactive
3//Output: Name and price of coffee orders or error messag
4.
In this lab, you use what you have learned about parallel
5. #include <iostream>
</>
arrays to complete a partially completed C++ program.
6 #include <string>
The program should:
7 using namespace std;
8)
• Either print the name and price for a coffee add-in
9 int main()
10 {
from the Jumpin' Jive Coffee Shop
|11
/ Declare variables
• Or it should print the message
12
string addIn;
/ Add-in ordered
13
const int NUM ITEMS
5; // Named constant
Sorry, we do not carry that.
14
WInitialized array of add-ins
string addIns[]
//Initiaized array of add-in prices
15
{"Cream", "Cinnamon", "Chocolate", "Amar
Read the problem description carefully before you
16
17
double addInPrices[]
{.89, .25, . 59, 1.50, 1.75};
/ Flag variable
begin. The file provided for this lab includes the
18
bool foundIt = false;
necessary variable declarations and input statements.
19
int x
//Loop control variable
double orderTotal = 2.00; // All orders start with a 2.00 ch
You need to write the part of the program that searches
20
21
22
for the name of the coffee add-in(s) and either prints
the name and price of the add-in or prints the error
// Get user input
23
cout << "Enter coffee add-in or XXX to quit:
message if the add-in is not found. Comments in the
24
cin >> addIn;
code tell you where to write your statements.
25
26
7/ Write the rest of the program here](https://content.bartleby.com/qna-images/question/4bfbb203-4399-4804-a6b8-bbfebafe4401/04d9555a-c5d5-434a-99f6-c277fd50bbca/hakq2us_thumbnail.jpeg)
Transcribed Image Text:ays
JumpinJive.cpP
1 // JumpinJava.cpp - This program looks up and prints the n
Summary
2 // Input:
Interactive
3//Output: Name and price of coffee orders or error messag
4.
In this lab, you use what you have learned about parallel
5. #include <iostream>
</>
arrays to complete a partially completed C++ program.
6 #include <string>
The program should:
7 using namespace std;
8)
• Either print the name and price for a coffee add-in
9 int main()
10 {
from the Jumpin' Jive Coffee Shop
|11
/ Declare variables
• Or it should print the message
12
string addIn;
/ Add-in ordered
13
const int NUM ITEMS
5; // Named constant
Sorry, we do not carry that.
14
WInitialized array of add-ins
string addIns[]
//Initiaized array of add-in prices
15
{"Cream", "Cinnamon", "Chocolate", "Amar
Read the problem description carefully before you
16
17
double addInPrices[]
{.89, .25, . 59, 1.50, 1.75};
/ Flag variable
begin. The file provided for this lab includes the
18
bool foundIt = false;
necessary variable declarations and input statements.
19
int x
//Loop control variable
double orderTotal = 2.00; // All orders start with a 2.00 ch
You need to write the part of the program that searches
20
21
22
for the name of the coffee add-in(s) and either prints
the name and price of the add-in or prints the error
// Get user input
23
cout << "Enter coffee add-in or XXX to quit:
message if the add-in is not found. Comments in the
24
cin >> addIn;
code tell you where to write your statements.
25
26
7/ Write the rest of the program here
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- / Swap.cpp - This program determines the minimum and maximum of three values inputby // the user and performs necessary swaps. // Input: Three int values. // Output: The numbers in ascending numerical order. #include <iostream>using namespace std;int main(){ // Declare variables int first = 0;// First number int second = 0;// Second number int third = 0;// Third number int temp; // Used to swap numbers // Get user input cout << "Enter first number: "; cin >> first; cout << "Enter second number: "; cin >> second; cout << "Enter third number: "; cin >> third; // Test to see if the first number is greater than the second number if it is swap the numbers // Test to see if the second number is greater than the third number if it is swap the numbers // Test to see if the first number is greater than the second number again if itis swap the numbers // Print numbers in numerical order cout << "Smallest: " << first << endl; cout…arrow_forward// Cornwall.cpp - This program computes hotel guest rates.// Input: None// Output: Hotel guest rate#include <iostream>#include <string>using namespace std;double computeRate(int);double computeRate(int, string);int main() { int days; string mealPlan; string question; double rate = 0.0; cout << "How many days do you plan to stay? " << endl; cin >> days; cout << "Do you want a meal plan? Y or N: " << endl; cin >> question; // Figure out which arguments to pass to the computeRate() function and // then call the computeRate() function cout << "The rate for your stay is $" << rate << endl; return 0;} // End of main() function// Write computeRate functions here.arrow_forwardC++ I have code: #include <bitset>#include <fstream>#include <iostream>#include <string> using namespace std;const std::string MORSE_CODE[] = {".-", "-...", "-.-.", "-..", ".", "..-.", "--.", "....", "..", ".---", "-.-", ".-..", "--", "-.", "---", ".--.", "--.-", ".-.", "...", "-", "..-", "...-", ".--", "-..-", "-.--", "--..", "-----", ".----", "..---", "...--", "....-", ".....", "-....", "--...", "---..", "----."};const char MORSE_DELIM = ' '; string decodeMorse(const string& morse) { string message; string word; bitset<8> bits; int bitIndex = 0; for (char c : morse) { if (c == MORSE_DELIM) { int letterIndex = -1; for (int i = 0; i < 26; ++i) { if (word == MORSE_CODE[i]) { letterIndex = i; break; } } if (letterIndex != -1) { bits.set(bitIndex, letterIndex & 1); ++bitIndex;…arrow_forward
- // JumpinJava.cpp - This program looks up and prints the names and prices of coffee orders.// Input: Interactive// Output: Name and price of coffee orders or error message if add-in is not found #include <iostream>#include <string>using namespace std; int main(){ // Declare variables. string addIn; // Add-in ordered const int NUM_ITEMS = 5; // Named constant // Initialized array of add-ins string addIns[] = {"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"}; // Initialized array of add-in prices double addInPrices[] = {.89, .25, .59, 1.50, 1.75}; bool foundIt = false; // Flag variable int x; // Loop control variable double orderTotal = 2.00; // All orders start with a 2.00 charge int findItem(); // Get user input cout << "Enter coffee add-in or XXX to quit: "; cin >> addIn; // Write the rest of the program here. findItem(); foundIt = false x = 0 while(x < NUM_ITEMS) if…arrow_forward// ChangeCase.cpp - This program converts characters to lowercase and uppercase.// Input: Interactive// Output: Uppercase and lowercase versions of the user-entered string#include <iostream>#include <string>using namespace std;int main() { const int LEN = 9; char sample1[LEN]; char sample2[LEN]; char result1; char result2; int i; cout << "Enter 9 lowercase characters: "; for(i = 0; i < LEN; i++) { cin >> sample1[i]; // Convert sample1[i] to uppercase and assign it to result1 cout << result1 << endl; } cin.ignore(256,'\n'); cout << "Enter 9 uppercase characters: "; for(i = 0; i < LEN; i++) { cin >> sample2[i]; // Convert sample2[i] to lowercase and assign it to result2 cout << result2 << endl; } cin.ignore(256,'\n'); return 0;} // End of main() functionarrow_forward// MovieGuide.cpp - This program allows each theater patron to enter a value from 0 to 4 // indicating the number of stars that the patron awards to the Guide's featured movie of the // week. The program executes continuously until the theater manager enters a negative number to // quit. At the end of the program, the average star rating for the movie is displayed. #include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables. double numStars; // star rating. double averageStars; // average star rating. double totalStars = 0; // total of star ratings. int numPatrons = 0; // keep track of number of patrons // This is the work done in the housekeeping() function // Get input. cout << "Enter rating for featured movie: "; cin >> numStars; // This is the work done in the detailLoop() function // Write while loop here //…arrow_forward
- // MichiganCities.cpp - This program prints a message for invalid cities in Michigan. // Input: Interactive // Output: Error message or nothing #include <iostream> #include <string> using namespace std; int main() { // Declare variables string inCity; // name of city to look up in array const int NUM_CITIES = 10; // Initialized array of cities string citiesInMichigan[] = {"Acme", "Albion", "Detroit", "Watervliet", "Coloma", "Saginaw", "Richland", "Glenn", "Midland", "Brooklyn"}; bool foundIt = false; // Flag variable int x; // Loop control variable // Get user input cout << "Enter name of city: "; cin >> inCity; // Write your loop here // Write your test statement here to see if there is // a match. Set the flag to true if city is found. // Test to see if city was not found to determine if // "Not a city in Michigan" message should be printed.…arrow_forwardQuestion 1: break phrase Problem statement Breaking a string into two parts based on a delimiter has applications. For example, given an email address, breaking it based on the delimiter "@" gives you the two parts, the mail server's domain name and email username. Another example would be separating a phone number into the area code and the rest. Given a phrase of string and a delimiter string (shorter than the phrase but may be longer than length 1), write a C++ function named break_string to break the phrase into two parts and return the parts as a C++ std::pair object (left part goes to the "first" and right part goes to the "second").arrow_forward// JumpinJava.cpp - This program looks up and prints the names and prices of coffee orders. // Input: Interactive // Output: Name and price of coffee orders or error message if add-in is not found #include <iostream> #include <string> using namespace std; int main() { // Declare variables. string addIn; // Add-in ordered const int NUM_ITEMS = 5; // Named constant // Initialized array of add-ins string addIns[] = {"Cream", "Cinnamon", "Chocolate", "Amaretto", "Whiskey"}; // Initialized array of add-in prices double addInPrices[] = {.89, .25, .59, 1.50, 1.75}; bool foundIt = false; // Flag variable int x; // Loop control variable double orderTotal = 2.00; // All orders start with a 2.00 charge // Get user input cout << "Enter coffee add-in or XXX to quit: "; cin >> addIn; // Write the rest of the program here. return 0; } // End of main()arrow_forward
- c++arrow_forward//main.cpp #include "Person.h"#include "Seller.h"#include "Powerseller.h"#include <iostream>#include <list>#include <fstream>#include <string> using namespace std; void printMenu();void printSellers(list<Seller*> sellers);void checkSeller(list<Seller*> sellers);void addSeller(list<Seller*> sellers);void deleteSeller(list<Seller*> &sellers); int main() { list<Seller*> sellers; ifstream infile; infile.open("sellers.dat"); if (!infile) { cerr << "File could not be opened." << endl; exit(1); } char type; while (!infile.eof()) { infile >> type; if (type == 'S') { Seller* newseller = new Seller; newseller -> read(infile); sellers.push_back(newseller); } if (type == 'P') { Powerseller* newpowerseller = new Powerseller; newpowerseller -> read(infile); sellers.push_back(newpowerseller); } } infile.close();…arrow_forward#include#include#includeusing namespace std;// outputHtmlTitle// parameters// This function...void outputHtmlTitle(ofstream & fout, string title){fout << "" << endl;fout << "" << endl;fout << "" << endl;fout << "" << endl;fout << title << endl;fout << "" << endl;}void outputHtmlFooter(ofstream & fout){fout << "" << endl;fout << "" << endl;}void outputHtmlList(ostream & fout, string first, string second, string third){fout << "" << endl;fout << "\t" << first << "" << endl;fout << "\t" << second << "" << endl;fout << "\t" << third << "" << endl;fout << "\t" << endl;}void main(int argc, char * *argv){ofstream htmlFile("myIntro.html");string title;cout << "Please enter the title: ";getline(cin, title);outputHtmlTitle(htmlFile, title);string name;string course1, course2, course3;cout…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
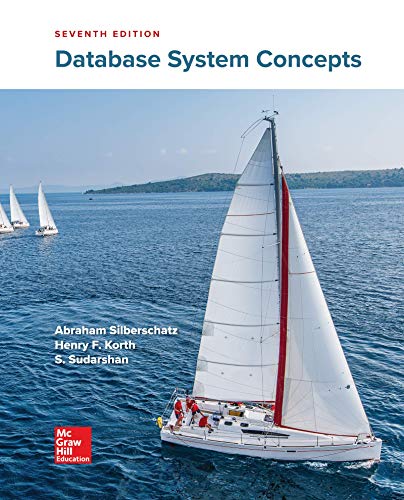
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
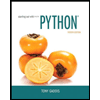
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
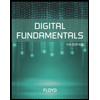
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
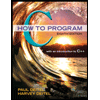
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
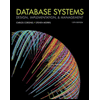
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
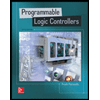
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education