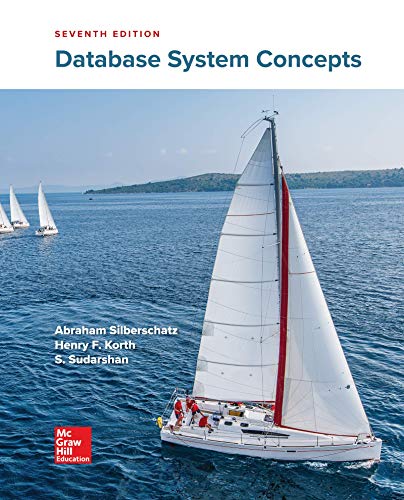
Concept explainers
Java Program
Requirements: Create a DodecahedronList class that stores the name of the list and an ArrayList of Dodecahedron objects. It also includes methods that return the name of the list, number of Dodecahedron objects in the DodecahedronList, total surface area, total volume, average surface area, average volume, and average surface to volume ratio for all Dodecahedron objects in the DodecahedronList. The toString method returns a String containing the name of the list followed by each Dodecahedron in the ArrayList, and a summaryInfo method returns summary information about the list.
Design: The DodecahedronList class has two fields, a constructor, and methods as outlined below.
(1) Fields (instance variables): a String representing the name of the list and an ArrayList of Dodecahedron objects. These are the only fields (instance variables) that this class should have.
(2) Constructor: Your DodecahedronList class must contain a constructor that accepts a parameter of type String representing the name of the list and a parameter of type ArrayList<Dodecahedron> representing the list of Dodecahedron objects. These parameters should be used to assign the fields described above (instance variables).
(3) Methods: The methods for DodecahedronList are described below.
o getName: Returns a String representing the name of the list.
o numberOfDodecahedrons: Returns an int representing the number of Dodecahedron objects in the DodecahedronList.
o totalSurfaceArea: Returns a double representing the total surface areas for all Dodecahedron objects in the list.
o totalVolume: Returns a double representing the total volumes for all Dodecahedron objects in the list.
o averageSurfaceArea: Returns a double representing the average surface area for all Dodecahedron objects in the list.
o averageVolume: Returns a double representing the average volume for all Dodecahedron objects in the list.
o averageSurfaceToVolumeRatio: Returns a double representing the average surface to volume ratio for all Dodecahedron objects in the list.
o toString: Returns a String (does not begin with \n) containing the name of the list followed by each Dodecahedron in the ArrayList. In the process of creating the return result, this toString() method should include a while loop that calls the toString() method for each Dodecahedron object in the list (adding a \n before and after each). Be sure to include appropriate newline escape sequences.
o summaryInfo: Returns a String (does not begin with \n) containing the name of the list (which can change depending of the value read from the file) followed by various summary items: number of Dodecahedrons, total surface area, total volume, average surface area, average volume, and average surface to volume ratio. Use "#,##0.0##" as the pattern to format the double values.
This is the code I have written for the first part of the program called Dodecahedron.java, I am requesting help with the second part that is described above.
import java.text.DecimalFormat;
public class Dodecahedron
{
private String label = "";
private String color = "";
private double edge = 0;
public Dodecahedron(String labelIn, String colorIn, double edgeIn)
{
setLabel(labelIn);
setColor(colorIn);
setEdge(edgeIn);
}
public String getLabel()
{
return label;
}
public boolean setLabel(String labelIn)
{
boolean isSetLabel = false;
if (labelIn != null)
{
label = labelIn.trim();
isSetLabel = true;
}
return isSetLabel;
}
public String getColor()
{
return color;
}
public boolean setColor(String colorIn)
{
boolean isSetColor = false;
if (colorIn != null)
{
color = colorIn.trim();
isSetColor = true;
}
return isSetColor;
}
public double getEdge()
{
return edge;
}
public boolean setEdge(double edgeIn)
{
boolean isSetEdge = false;
if (edgeIn > 0)
{
edge = edgeIn;
isSetEdge = true;
}
return isSetEdge;
}
public double surfaceArea()
{
double a = (3 * Math.sqrt(25 + 10 * Math.sqrt(5)) * Math.pow(edge, 2));
return a;
}
public double volume()
{
double v = (15 + 7 * Math.sqrt(5)) / 4 * Math.pow(edge, 3);
return v;
}
public double surfaceToVolumeRatio()
{
double s = surfaceArea() / volume();
return s;
}
public String toString()
{
DecimalFormat fmt = new DecimalFormat("#,##0.0##");
String output = "Dodecahedron \"" + label + "\" is \"" + color
+ "\" with 30 edges of length " + edge + " units.\n";
output += "\tsurface area = " + fmt.format(surfaceArea())
+ " square units \n";
output += "\tvolume = " + fmt.format(volume()) + " cubic units\n";
output += "\tsurface/volume ratio = "
+ fmt.format(surfaceToVolumeRatio());
return output;
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 6 steps with 4 images

- 1) What is one main disadvantage of an ArrayList? 2) Write a Java statement to create an ArrayList called list to hold 25 integers. 3) What is the type of the ArrayList defined in question number 2? 4) Write a for loop to initialize the objects in the ArrayList created in question number 2 above to -1.arrow_forward11:02 all 10% + Create 1 1000.01 Create 2 2000.02 Create 3 3000.03 Deposit 111.11 Deposit 2 22.22 Withdraw 4 5000.00 Create 4 4000.04 Withdraw 1 0.10 Balance 2 Withdraw 2 0.20 Deposit 3 33.33 Withdraw 4 0.40 Bad Command 65 Balance 1 Balance 2 Balance 3 Balance 4arrow_forwardJava code 8. Given an existing ArrayList named friendList, find the first index of a friend named Sasha and store it in a new variable named index. 9. Given that an ArrayList of Integers named numberList has already been created and several values have already been inserted, write the statement necessary to insert the value 25 at the end of the list. 10. Declare an integer array named evens and fill it with even numbers from 2 through 10 in one statement. 11. Given an existing array named pets, find the size of the array and store it in a new variable named numPets. 12. Given an existing ArrayList named contactList, find the number of contacts in the ArrayList and store it in the existing variable named numContacts.arrow_forward
- In C#, Make any necessary modifications to the RushJob class so that it can be sorted by job number. Modify the JobDemo3 application so the displayed orders have been sorted. Save the application as JobDemo4. An example of the program is shown below: Enter job number 22 Enter customer name Joe Enter description Powerwashing Enter estimated hours 4 Enter job number 6 Enter customer name Joey Enter description Painting Enter estimated hours 8 Enter job number 12 Enter customer name Joseph Enter description Carpet cleaning Enter estimated hours 5 Enter job number 9 Enter customer name Josefine Enter description Moving Enter estimated hours 12 Enter job number 21 Enter customer name Josefina Enter description Dog walking Enter estimated hours 2 Summary: RushJob 6 Joey Painting 8 hours @$45.00 per hour. Rush job adds 150 premium. Total price is $510.00 RushJob 9 Josefine Moving 12 hours @$45.00 per hour. Rush job adds 150 premium. Total price is $690.00 RushJob 12 Joseph Carpet cleaning 5…arrow_forwardshapes = [{'type': 'circle', 'x': 300, 'y': 300, 'radius': 100, 'color': 'cyan'},{'type': 'circle', 'x': 300, 'y': 300, 'radius': 10, 'color': 'white'},{'type': 'rectangle', 'x1': 500, 'y1': 500, 'x2': 550, 'y2': 580, 'color': 'green'},{'type': 'line', 'x': 0, 'y': 0, 'a': 100, 'b': 300, 'color': "black", 'width': 7},{'type': 'point', 'x': 200, 'y': 50, 'color': 'black'},{'type': 'point', 'x': 205, 'y': 50, 'color': 'black'},{'type': 'point', 'x': 210, 'y': 50, 'color': 'black'},{'type': 'triangle', 'x': 500, 'y': 100, 'a': 600, 'b': 100, 'c': 550, 'd': 200, 'color': 'yellow'},{'type': 'oval', 'x': 100, 'y': 100, 'a': 400, 'b': 400, 'color': 'red'},{'type': 'text', 'x': 500, 'y': 50, 'message': 'hello world!', 'color': 'blue'}] I'd like to take this list and write it to a new txt file. Ignoring the ':' , {}, and words within quotation marks. so the txt file created would look like this: circle, 300,300, 100, cyancircle, 300,300, 10, whiterectangle, 500,500, 550,580, greenline, 0,0,…arrow_forwardDesign an application that declares an array of 10 StockTransactionobjects. Prompt the user for data for each object, and then display all the values. Design an application that declares an array of 10 StockTransactionobjects. Prompt the user for data for each object, and then pass the array to a method that determines and displays the two stocks with the highest and lowest price per share.arrow_forward
- Data structure & Algrothium java program Create the three following classes: 1. class containing two strings attributes first name and last name.2. class containing the name object and an ArrayList of string to store the list gifts. This class would extend the attached NicePersonInterface.java3. class containing one ArrayList of Names to store the naughty names. Another ArrayList of NicePerson to store the nice names and gifts. Add atleast 4 names in each list and display all information.arrow_forward•is Valid Phone Number(): ReturnsTrue if the provided phone number (represented as a string) is cor-rectly formatted, otherwiseFalse. To be considered correctly formatted, phone numbers must bewritten as###-###-####, where#is a digit between 0 and 9 .•validatePhoneBook(): A phone book is a list where each entry is a phone book record (represented asa dictionary; see below for more details). This function checks each phone book record in the phonebook for correctly formatted phone numbers.A phone book record is a dictionary which initially has two keys: the key"name"mapped to thecontact’s name (string) and the key"phone number"mapped to that contact’s phone number (alsoa string).validatePhoneBook()adds a new key"valid"to the record with the valueTrueif the phonenumber is formatted correctly, otherwiseFalse. 1. Write white-box and black-box tests for the function is ValidPhoneNumber(). You should do this with-out Python, either on paper, or in a simple document. Don’t worry about…arrow_forwardCreate an array of three string values that the fullName variable will use as a point of reference. Make an initialization list for the array using string values for the first, middle, and last names.arrow_forward
- Create an array of three string values that the fullName variable will use as a point of reference. Make an initialization list for the array using string values for the first, middle, and last names.arrow_forwardMicrosoft Visual C# 7th edition Create a program for Smalltown Regional Airport Flights that accepts either an integer flight number or string airport code from the options in Figure 8-33. Pass the user’s entry to one of two overloaded GetFlightInfo() methods, and then display a returned string with all the flight details. For example, if 201 was input, the output would be: Flight #201 AUS Austin Scheduled at: 0710 (note that there should be two spaces between 'Austin' and 'Scheduled'). The method version that accepts an integer looks up the airport code, name, and time of flight; the version that accepts a string description looks up the flight number, airport name, and time. The methods return a message if the flight is not found. For example, if 100 was input, the output should be Flight #100 was not found. If no flights were scheduled for the airport code entered, for example MCO, the message displayed should be Flight to MCO was not found. I am having these errors 1-…arrow_forwardmy_games = ['Zelda', 'Pokemon', 'Splatoon'] my_games[1] = 'Minecraft' Draw (or describe) the objects and labels that the Python interpreter creates in response to the first assignment. Then draw (or describe) the objects and labels that results from carrying out the second assignment immediately after the first assignment. Include your drawings or text descriptions in the solution document.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
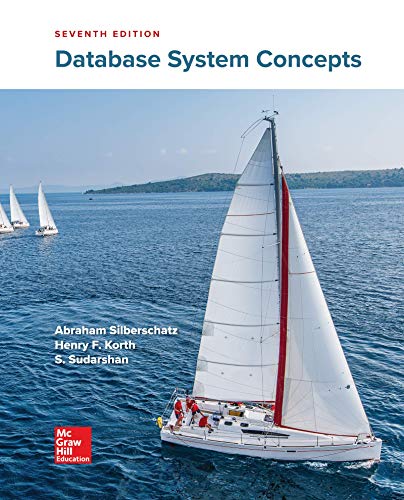
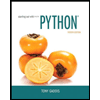
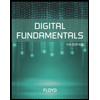
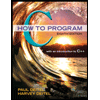
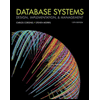
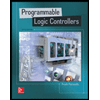