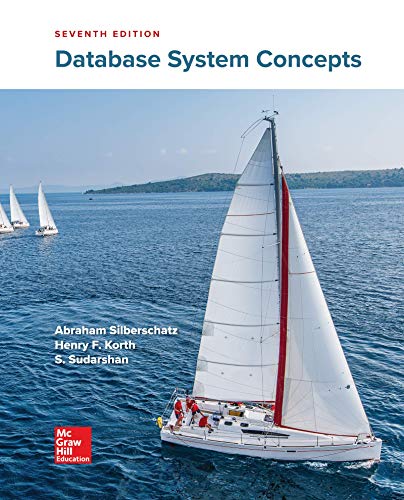
JAVA PROGRAM
import java.io.*;
import java.util.Scanner;
public class FileTotalAndAverage {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("Please enter the file name: ");
while (true) {
String fileName = scanner.nextLine();
File file = new File(fileName);
if (file.exists() && file.isFile()) {
processFile(file);
break; // Exit loop if file is found and processed
} else {
System.out.println("File '" + fileName + "' does not exist.\nPlease enter the file name again: ");
}
}
scanner.close();
}
private static void processFile(File file) {
try {
Scanner fileScanner = new Scanner(file);
double total = 0;
int count = 0;
while (fileScanner.hasNextDouble()) {
double number = fileScanner.nextDouble();
total += number;
count++;
}
fileScanner.close();
double average = (count == 0) ? 0 : total / count;
System.out.printf("Total: %.3f%n", total);
System.out.printf("Average: %.3f%n", average);
} catch (FileNotFoundException e) {
// This block should never be reached as we checked if the file exists before
System.err.println("Unexpected error: " + e.getMessage());
}
}
}
-456.167
19.815
-322.301
344.949
-850.533
-672.360
-188.767
646.462
-118.775
808.613
-746.865
-370.432
219.607
-166.298
-508.636
-989.128
-205.020
-928.165
-180.699
300.753
316.036
709.371
-886.860
-585.388
-554.302
-394.801
-970.233
905.941
787.854
-181.240
74.665
802.453
-951.292
-510.656
-203.999
-276.199
-350.575
398.501
-519.799
469.199
592.120
713.424
155.967
585.481
-780.846
387.700
457.806
560.933
-343.916
486.806
-43.184
237.494
191.488
309.275
-64.415
-206.333
-377.696
-409.553
-734.282
-777.221
-318.800
-695.745
40.631
-384.036
-937.134
-380.501
-77.083
756.524
-720.959
-579.412
-215.301
542.097
-402.541
468.721
151.050
573.296
-342.210
758.038
200.691
882.294
8.042
-87.760
634.811
-777.953
767.795
570.716
594.012
596.648
900.010
-370.754
985.007
785.562
-838.952
-71.182
72.845
-500.759
698.946
527.167
1.082
Test Case 1
double_input1.txtENTER
Total: -5,748.583\n
Average: -57.486\n
Test Case 2
double_input2.txtENTER
Total: 112,546.485\n
Average: 56.273\n
Test Case 3
double_input3.txtENTER
File 'double_input3.txt' does not exist.\n
Please enter the file name again: \n
double_input1.txtENTER
Total: -5,748.583\n
Average: -57.486\n

Step by stepSolved in 4 steps with 3 images

- **** In Java Please **** Task : Please write a program or a code to calculate the frequency percentage of the occurrences of the characters in a text file.arrow_forwardJava code File IO ApplicationA shop collects the following information from its customers:1. Full name (first name, last name, and optional middle initial) 2. Address (street name and number, city, zip code, and state)3. Phone number 4. Email 5. First visit/service date6. Most recent visit/service date7. Total amount charged8. Current balance and description of the last service You are to write a program that allow the shop to manage their customers. The shop needs the following functionalities:1. Search for a customer by:a. Nameb. Phone numberc. Email (The result of the search would have similar option as in the “List all customers” option below)2. List all customersa. Select and edit a customer b. Delete a customer3. Add a new customera. Prompt for appropriate data fields4. Get account consolidation sheet that shows total charges and total balance of all customers5. Get the records of the biggest 5 spenders (spend the most)6. Exit and save all changes (all changes to the customers…arrow_forwardJAVA PROGRAM Chapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage I need a "," (comma) to the number format here (after the first %):System.out.printf(Locale.US, "Total: %.3f%n", total); Also, I don't need to set the Locale. PLEASE FIX AND MODIFY THIS JAVA SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL TEST CASSES PLEASES. RIGHT NOW IT SAYS 0 OUT 3 PASSED. THE PICTURES THAT I PROVIDED PROOF THAT WHEN I UPLOAD IT TO HYPERGRADES IT FAILS TEST CASSES. THANK YOU import java.io.*;import java.util.Scanner;import java.util.Locale;public class FileTotalAndAverage { public static void main(String[] args) { Scanner scanner = new Scanner(System.in);…arrow_forward
- pythonarrow_forwardCan this be done in Java and not C++arrow_forwardJava Program ASAP ************This program must work in hypergrade and pass all the test cases.********** The text files are located in Hypergrade. Also for test case 1 first display Please enter a string to convert to Morse code:\n then you press Enter it should print out \n. Then for test case 2 it should display Please enter a string to convert to Morse code:\n then you type abc it should print out .- -... -.-. \n For test case 3 first display Please enter a string to convert to Morse code:\n then you type This is a sample string 1234.ENTER it should print put - .... .. ... .. ... .- ... .- -- .--. .-.. . ... - .-. .. -. --. .---- ..--- ...-- ....- .-.-.- \n. This program down below does not pass the test cases as shown in the screenshot I have provided the correct test case as a screenshot too. Please modify it or create a new program so it paases the test cases. Thank you! For test case 1 it wants only Please enter a string to convert to Morse…arrow_forward
- Java codearrow_forwardJAVA PROGRAM Chapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage PLEASE FIX AND MODIFY THIS JAVA SO WHEN I UPLOAD IT TO HYPERGRADE IT PASSES ALL TEST CASSES PLEASES. RIGHT NOW IT SAYS 0 OUT 3 PASSED. THE PICTURES THAT I PROVIDED PROOF THAT WHEN I UPLOAD IT TO HYPERGRADES IT FAILS TEST CASSES. THANK YOU. import java.io.*;import java.util.Scanner;public class FileTotalAndAverage { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Please enter the file name: "); while (true) { String fileName = scanner.nextLine(); File file = new File(fileName); if (file.exists() &&…arrow_forwardJAVA PROGRAMMINGIf Statements - Program #1Ms. Monroe is an Income Tax Practitioner who operated a service that prepares income taxforms. Her fees are based on the type of form filed and yearly income.Program Specifications:a. Create a folder named If Program 01. Save the Java file as TaxPrep.b. Input consists of the customer's name, the form type (1 - short form; 2 - long form), andthe yearly income. Sample input is illustrated on page 2. You will enter the input from thekeyboard. Input prompts should be very specific. When inputting the form type makesure that you display 1 – Short Form or 2 – Long Form. The user needs to know what toenter. This program will process one record and only one record at a time.c. The basic fee is $100 for a short form (form type of 1) or $200 for a long form (form typeof 2). The income fee is based on the yearly income. If the yearly income is less the$50,000, the income fee is the same as the basic fee. If the yearly income is $50,000 ormore, the income…arrow_forward
- java Program Chapter 4. Homework Assignment (read instructions carefully) Write a program that asks the user for the name of a file. The program should read all the numbers from the given file and display the total and average of all numbers in the following format (three decimal digits): Total: nnnnn.nnn Average: nnnnn.nnn Class name: FileTotalAndAverage I do not want a goodbye system out in the program and it has to pass all the test cases. Test Case 1 Please enter the file name or type QUIT to exit:\ninput1.txtENTERTotal: 11.800\nAverage: 2.950\n Test Case 2 Please enter the file name or type QUIT to exit:\ninput2.txtENTERTotal: 17.300\nAverage: 3.460\n Test Case 3 Please enter the file name or type QUIT to exit:\ninput3.txtENTERTotal: 1.124\nAverage: 1.124\n Test Case 4 Please enter the file name or type QUIT to exit:\ninput4.txtENTERFile input4.txt is empty.\n Test Case 5 Please enter the file name or type QUIT…arrow_forwardJava programming : I can’t figure question 9 out. Help would be appreciated of any input or output. Thanks!arrow_forwardUsing Java PrintWriter, write a program that prompts the user for information and saves it to a text file File name is musicians.txt Use this information below: George,Thoroughgood,54321 Ludwig,Van Beethoven,90111 Edward,Van Halen,12345arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
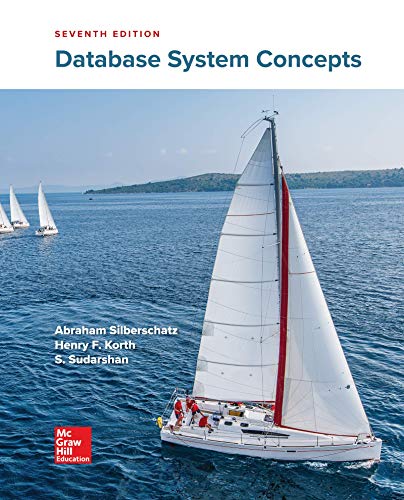
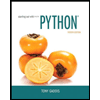
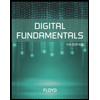
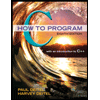
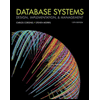
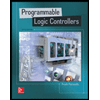