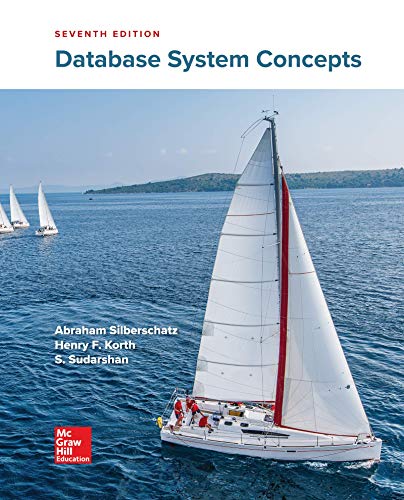
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Java help, can you please explain this I'm a beginner and I'm lost:(
Implement a nested class DoubleNode for building doubly-linked lists, where each node contains
a reference to the item preceding it and the item following it in the list (null if there is no such
item). Then implement methods for the following tasks:
• Print the contents of the list
• Insert at the beginning
• Insert at the end
• Remove from the beginning
• Remove from the end
• Insert before a give node (Insert before the first occurrence of the node, if the
node exists; else insert at the end)
• Insert after a given node (Insert after the first occurrence of the node, if the node
exists; else insert at the end)
• Remove a given node (Remove the first occurrence of the node, remove nothing if
node not found)
• Move to front (move the first occurrence of the node to the front)
• Move to end (moved and first occurrence of the node to the end)
Write a tester program and test your implementation. You must test each of these cases and print
the list after each test and verify the correctness of the code.
Examples:
Suppose the link list contains the following elements:
C-> O -> M -> P -> U -> T -> E
1. Print the contents of the list:
C-> O -> M -> P -> U -> T -> E
2. Insert M at the beginning of the list:
M -> C-> O -> M -> P -> U -> T -> E
3. Insert R at the end:
M -> C-> O -> M -> P -> U -> T -> E -> R
4. Remove from the beginning:
C-> O -> M -> P -> U -> T -> E -> R
a reference to the item preceding it and the item following it in the list (null if there is no such
item). Then implement methods for the following tasks:
• Print the contents of the list
• Insert at the beginning
• Insert at the end
• Remove from the beginning
• Remove from the end
• Insert before a give node (Insert before the first occurrence of the node, if the
node exists; else insert at the end)
• Insert after a given node (Insert after the first occurrence of the node, if the node
exists; else insert at the end)
• Remove a given node (Remove the first occurrence of the node, remove nothing if
node not found)
• Move to front (move the first occurrence of the node to the front)
• Move to end (moved and first occurrence of the node to the end)
Write a tester program and test your implementation. You must test each of these cases and print
the list after each test and verify the correctness of the code.
Examples:
Suppose the link list contains the following elements:
C-> O -> M -> P -> U -> T -> E
1. Print the contents of the list:
C-> O -> M -> P -> U -> T -> E
2. Insert M at the beginning of the list:
M -> C-> O -> M -> P -> U -> T -> E
3. Insert R at the end:
M -> C-> O -> M -> P -> U -> T -> E -> R
4. Remove from the beginning:
C-> O -> M -> P -> U -> T -> E -> R
5. Remove from the end:
C-> O -> M -> P -> U -> T -> E
6. Insert M before P:
C-> O -> M -> M -> P -> U -> T -> E
7. Insert H before M:
C-> O -> H -> M -> M -> P -> U -> T -> E -> B
8. Insert B before A:
C-> O -> M -> M -> P -> U -> T -> E -> B
9. Insert C after P:
C-> O -> M -> M -> P-> C -> U -> T -> E -> B
10.Insert L after M:
C-> O -> M -> L -> M -> P -> C-> -> U -> T -> E -> B
11.Remove M
C-> O -> L -> M -> P -> C-> -> U -> T -> E -> B
12.Remove G
C-> O -> L -> M -> P -> C-> -> U -> T -> E -> B
13.Move P to the front of the list:
P -> C-> O -> L -> M -> C-> -> U -> T -> E -> B
14.Move L to the end of the list:
P -> C-> O -> M -> C-> -> U -> T -> E -> B -> L
C-> O -> M -> P -> U -> T -> E
6. Insert M before P:
C-> O -> M -> M -> P -> U -> T -> E
7. Insert H before M:
C-> O -> H -> M -> M -> P -> U -> T -> E -> B
8. Insert B before A:
C-> O -> M -> M -> P -> U -> T -> E -> B
9. Insert C after P:
C-> O -> M -> M -> P-> C -> U -> T -> E -> B
10.Insert L after M:
C-> O -> M -> L -> M -> P -> C-> -> U -> T -> E -> B
11.Remove M
C-> O -> L -> M -> P -> C-> -> U -> T -> E -> B
12.Remove G
C-> O -> L -> M -> P -> C-> -> U -> T -> E -> B
13.Move P to the front of the list:
P -> C-> O -> L -> M -> C-> -> U -> T -> E -> B
14.Move L to the end of the list:
P -> C-> O -> M -> C-> -> U -> T -> E -> B -> L
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 5 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Create a nested class called DoubleNode that allows you to create doubly-linked lists with each node containing a reference to the item before it and the item after it (or null if neither of those items exist). Then implement static methods for the following operations: insert before a given node, insert after a given node, remove a given node, remove from a given node, insert at the beginning, insert at the end, remove from a given node, and remove a given node.arrow_forwardUsing Java, modify the doubly linked list class presented below so it works with generic types. Add the following methods drawn from the java.util.List interface:• void clear(): remove all elements from the list.• E get(int index): return the element at position index in the list.• E set(int index, E element): replace the element at the specified position with the specified element and return the previous element. Test your generic linked list class by processing a list of numbers of type double. ====================================/**The DLinkedList class implements a doubly Linked list. */class DLinkedList {/**The Node class stores a list element and a reference to the next node.*/private class Node{String value; // Value of a list elementNode next; // Next node in the listNode prev; // Previous element in the list/**Constructor. @param val The element to be stored in the node.@param n The reference to the successor node.@param p The reference to the predecessor node.*/ Node(String…arrow_forwardIn python. Write a LinkedList class that has recursive implementations of the add and remove methods. It should also have recursive implementations of the contains, insert, and reverse methods. The reverse method should not change the data value each node holds - it must rearrange the order of the nodes in the linked list (by changing the next value each node holds). It should have a recursive method named to_plain_list that takes no parameters (unless they have default arguments) and returns a regular Python list that has the same values (from the data attribute of the Node objects), in the same order, as the current state of the linked list. The head data member of the LinkedList class must be private and have a get method defined (named get_head). It should return the first Node in the list (not the value inside it). As in the iterative LinkedList in the exploration, the data members of the Node class don't have to be private. The reason for that is because Node is a trivial class…arrow_forward
- Implement a simple linked list in Python (Write source code and show output) with basic linked list operations like: (a) create a sequence of nodes and construct a linear linked list. (b) insert a new node in the linked list. (b) delete a particular node in the linked list. (c) modify the linear linked list into a circular linked list. Use this template: class Node:def __init__(self, val=None):self.val = valself.next = Noneclass LinkedList:"""TODO: Remove the "pass" statements and implement each methodAdd any methods if necessaryDON'T use a builtin list to keep all your nodes."""def __init__(self):self.head = None # The head of your list, don't change its name. It shouldbe "None" when the list is empty.def append(self, num): # append num to the tail of the listpassdef insert(self, index, num): # insert num into the given indexpassdef delete(self, index): # remove the node at the given index and return the deleted value as an integerpassdef circularize(self): # Make your list circular.…arrow_forwardTrace insertion sort for list ={18,57,8,89,7}arrow_forwardOnly 2arrow_forward
- JAVA please Given main() in the ShoppingList class, define an insertAtEnd() method in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts Code provided in the assignment ItemNode.java:arrow_forwardJava Programming language Please help me with this. Thanks in advance.arrow_forwardImplement a Single linked list to store a set of Integer numbers (no duplicate) • Instance variable• Constructor• Accessor and Update methods 3. Define TestSLinkedList Classa. Declare an instance of the Single List class.b. Test all the methods of the Single List class.arrow_forward
- Use Java : Delete N Nodes After M Nodes of a Linked List You are given the head of a linked list and two integers m and n.Traverse the linked list and remove some nodes in the following way:- Start with the head as the current node.- Keep the first m nodes starting with the current node.- Remove the next n nodes- Keep repeating steps 2 and 3 until you reach the end of the list.Return the head of the modified list after removing the mentioned nodesarrow_forwardJava Design and draw a method called check() to check if characters in a linked list is a palindrome or not e.g "mom" or "radar" or "racecar. spaces are ignored, we can call the spaces the “separator”. The method should receive the separator as a variable which should be equal to “null” when no separator is used.arrow_forwardDon't give me AI generated answer or plagiarised answer. If I see these things I'll give you multiple downvotes and will report immediately.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
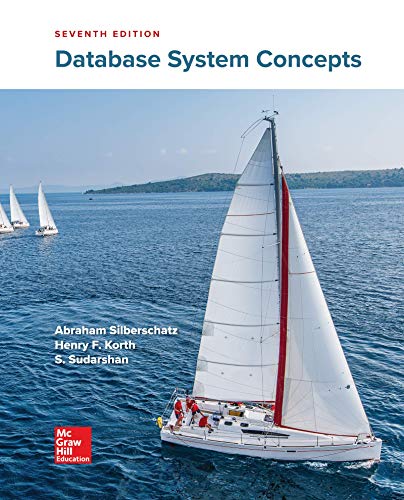
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
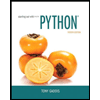
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
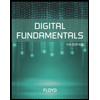
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
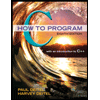
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
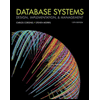
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
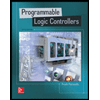
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education