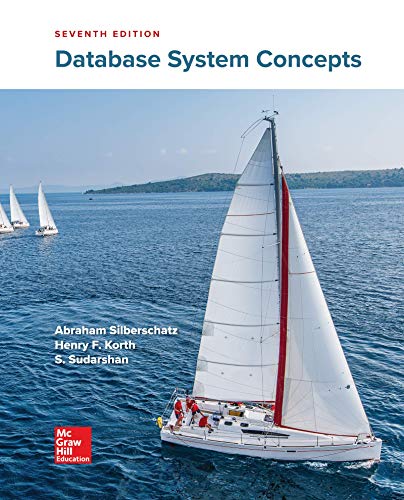
Concept explainers
Given code is provided down below. Please do not change the existing code. The instructions are in the image. Please explain and show each step and a screenshot of the input and output. Thank you.
main.cpp
#include "Triangle.h"
#include "Square.h"
#include <iostream>
using namespace std;
int main() {
//Do not modify
return 0;
}
-------------------------------------------
Square.cpp
#include "Square.h"
#include <iostream>
using namespace std;
Square::Square(){
base = 0;
height = 0;
}
Square::Square(int b, int h) : base(b), height(h) { }
void Square::setBase(int b){
base = b;
}
void Square::setHeight(int h){
height = h;
}
void Square::calculateArea(){
// calculate Area correctly depending on shape type
}
int Square::getBase() const{
return base;
}
int Square::getHeight() const{
return height;
}
double Square::getArea() const{
return area;
}
// Overloaded << operator
// Overloaded >> operator
-------------------------------------------
Square.h
#ifndef SQUARE_H_
#define SQUARE_H_
#include <iostream>
using namespace std;
class Square {
public:
Square();
Square(int b, int h);
//Setters
void setBase(int b);
void setHeight(int h);
//Getters
int getBase() const;
int getHeight() const;
double getArea() const;
void calculateArea();
// Overloaded << operator declaration
// Overloaded >> operator declaration
private:
int base;
int height;
double area;
};
#endif
-------------------------------------------
Triangle.cpp
#include "Triangle.h"
#include <iostream>
using namespace std;
Triangle::Triangle(){
base = 0;
height = 0;
}
Triangle::Triangle(int b, int h) : base(b), height(h) { }
void Triangle::setBase(int b){
base = b;
}
void Triangle::setHeight(int h){
height = h;
}
void Triangle::calculateArea(){
// calculate Area correctly depending on shape type
}
int Triangle::getBase() const{
return base;
}
int Triangle::getHeight() const{
return height;
}
double Triangle::getArea() const{
return area;
}
// Overloaded << operator declaration
// Overloaded >> operator declaration
-------------------------------------------
Triangle.h
#ifndef TRIANGLE_H_
#define TRIANGLE_H_
#include <iostream>
using namespace std;
class Triangle {
public:
Triangle();
Triangle(int b, int h);
//Setters
void setBase(int b);
void setHeight(int h);
//Getters
int getBase() const;
int getHeight() const;
double getArea() const;
void calculateArea();
// Overloaded << operator declaration
// Overloaded >> operator declaration
private:
int base;
int height;
double area;
};
#endif
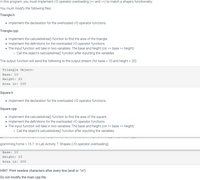

Trending nowThis is a popular solution!
Step by stepSolved in 7 steps with 1 images

- program Credit Card Validator - Takes in a credit card number from a common credit card vendor (Visa, MasterCard, American Express, Discover) and validates it to make sure that it is a valid number (look into how credit cards use a checksum). please use #include <iostream>arrow_forward// LargeSmall.cpp - This program calculates the largest and smallest of three integer values. #include <iostream> using namespace std; int main() { // This is the work done in the housekeeping() function // Declare and initialize variables here int largest; // Largest of the three values int smallest; // Smallest of the three values // Prompt the user to enter 3 integer values // Write assignment, add conditional statements here as appropriate // This is the work done in the endOfJob() function // Output largest and smallest number. cout << "The largest value is " << largest << endl; cout << "The smallest value is " << smallest << endl; return 0; }arrow_forwardPlease draw the flowchart for this code,I need under a few minutes please,it immediate and urgent #include int main() { int firstTerm, secondTerm, thirdTerm; std::cout << "Enter the first term: "; std::cin >> firstTerm; std::cout << "Enter the second term: "; std::cin >> secondTerm; std::cout << "Enter the third term: "; std::cin >> thirdTerm; int commonDifference = secondTerm - firstTerm; int sum = (300 * (2 * firstTerm + (300 - 1) * commonDifference)) / 2; std::cout << "Sum of the first 300 terms: " << sum << std::endl; std::cout << "The first fifty terms are: "; for (int i = 0; i < 50; i++) { std::cout << firstTerm + i * commonDifference << " "; } return 0; }arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
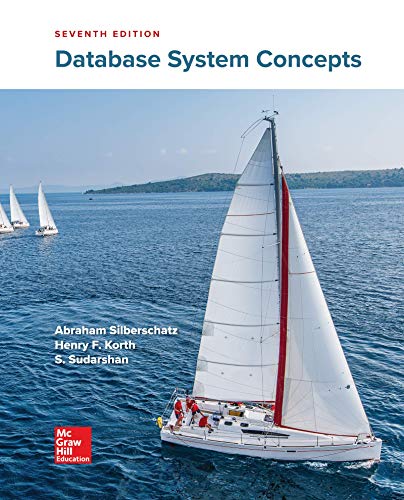
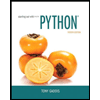
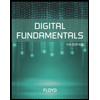
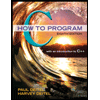
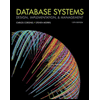
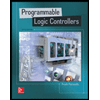